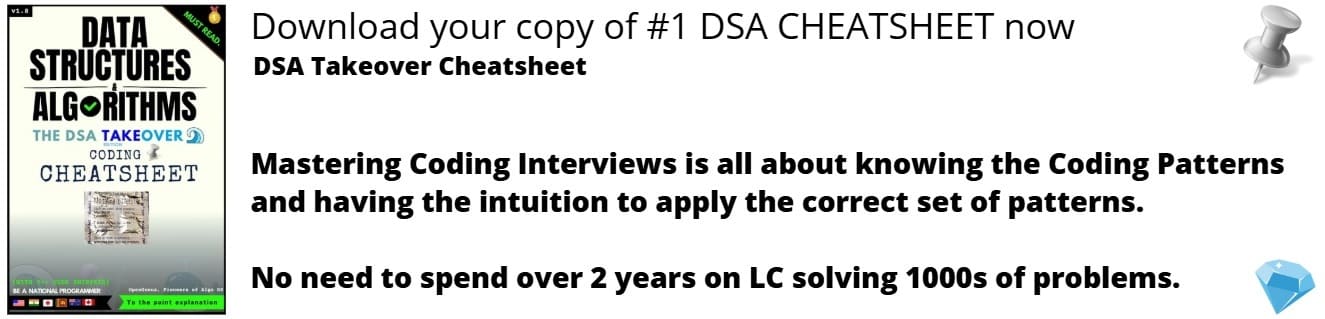
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained Different ways to initialize List in C++ STL including Initializing list with elements of another list, using a vector or array and much more.
Table of contents:
- Introduction to List in C++ STL
- Initializing an empty list
- Initializing list with specified values
- Initializing list with elements of another list
- Initializing list with an array or a vector
- Initializing list of specified size with specified variable
Prerequisite: List in C++ STL
Introduction to List in C++ STL
A list in C++ is a sequence container (a sequence container is a collection of objects which can be accessed sequentially), which allows objects to be stored in non-contiguous memory locations.
When talking about a list in C++, we refer to a doubly linked list (a singly linked list is referred to as a forward list). A doubly linked list is a linked list where each element has two pointers, one pointing to the next element and the other to the previous element in the list.
In order to use lists, one needs to include the header file list. There are several ways to initialize lists in C++, and we shall discuss them in detail:
- Initializing an empty list
- Initializing list with specified values
- Initializing list with elements of another list
- Initializing list with an array or a vector
- Initializing list of specified size with specified variable
Let us dive deeper into each technique.
1. Initializing an empty list
This is used to create a list without any elements. The syntax used here is as follows:
list<datatype> listname;
After initializing an empty list, once can insert and delete variables of the declared datatype.
2. Initializing list with specified values
This is used when one needs to create a list with a set of values, which needs to be stored inside an initializer list (a collection of elements within braces {}). The syntax for the same is as follows:
list<datatype> listname(initializer_list);
An example of initializing a list with specified values is given below:
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> list1({1,5,7});
for(int i:list1){
cout<<i<<endl;
}
}
Here, the for loop iterates through the list, and prints each element.
3. Initializing list with elements of another list
This method is used when one wants to initialize a list with the elements in a pre-existing list. Here, the syntax is as follows:
list<datatype> list2name(list1name);
Note that the datatype of objects of the new list needs to match that of the pre-existing list. An example of this method is given below:
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> list1({1, 5, 7});
list<int> list2(list1);
for(int i:list2){
cout<<i<<endl;
}
return 0;
}
4. Initializing list with an array or a vector
This method can be used when one already has a collection of objects stored in an array or a vector, and needs to copy it into a list. The syntax to be used is as follows:
list<datatype> listname(begin(vals), end(vals))
where vals is an array or vector, begin(vals) points to the first element of vals, and end(vals) points to the last element. Alternatively, one can use the sizeof() operator to point to the end of vals, if it is an array.
An example of such initialization is given below:
#include<iostream>
#include<list>
#include<vector>
using namespace std;
int main()
{
int A[] = {1,2,3};
vector<char> B({'A', 'B', 'C'});
list<int> list1(A, A + sizeof(A)/sizeof(int));
list<char> list2(begin(B), end(B));
for(int i:list1){
cout<<i<<endl;
}
for(char i:list2){
cout<<i<<endl;
}
return 0;
}
5. Initializing list of specified size with specified variable
This method is used to initialize a list with a given number of elements, each element being a copy of a specified element (which is passed as an argument). The syntax used is as follows:
list<datatype> listname(size, val);
where size refers to the size of the list, and val refers to the element whose copies shall be stored in each position of the list. An example of this method is given below:
#include<iostream>
#include<list>
using namespace std;
int main()
{
int size = 5;
char val = 'C';
list<char> list1(size, val);
for(char i:list1){
cout<<i<<endl;
}
return 0;
}
This initializes a list with 5 elements, each of them being 'C'.
Question
In C++, list refers to which of the following?
With this article at OpenGenus, you must have the complete idea of Different ways to initialize List in C++ STL.