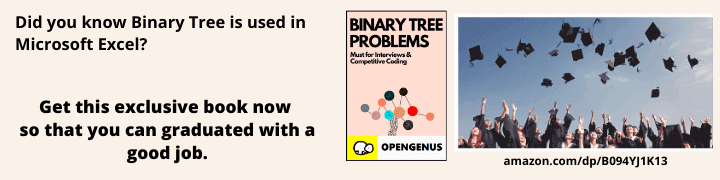
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will see how can we integrate our android application to firebase real-time database. So lets get started.
First of all lets see what are the prerequisites.
- A Google Firebase Account.
- Android Studio installed on your workstation.
- Internet (of course XD).
Table of contents:
- Creating database in firebase account.
- Connecting Android Studio to Database.
- Coding the application.
Creating database in firebase account
First of all, login to your firebase account. After logging in, Tap on Add Project in order to create a new firebase database in your account. Specify the name of our database and click on continue. You will be asked for google analytics for firebase project, you can enable or disable it. Then again click on continue. Now choose default account and press on continue. Now we have successfully created database for our application. We are ready for the next step.
Connecting Android Studio to Database
Open Android studio in you workstation and create a new android application with an empty activity. Specify you desired name and make sure you select Java as language. Now click on finish, we have successfully created our android application.
After creating the application, Navigate to Tools option in menubar and click on Firebase inside the dragdown list of tools. A Sidebar will appear after you click on Firebase. Now click on Realtime Database. Click the first option that says Get Started with Realtime Database and perform the following steps.
- Click on Connect to Firebase.
- It can ask you to build the application first, build it.
- It will redirect you to your browser in which you have to select the database for you application.
- Choose the database we created earlier.
- Click on Connect, it will show you acknowledgment that Your Android Studio project is connected to your Firebase Android app.
- Click on Add the Realtime Database to you app.
- It will add the firebase realtime database library to your android application.
- Click on Accept Changes.
- It will build you application again. Now we are good to go.
You can now minimize the sidebar of Firebase configuration.
Coding the application
Now its time to write the final code of our application which will perform database operations. Open up the activity_main.xml. You can choose whatever layout that you like, I'll be choosing relative layout. Add some EditText, Buttons, TextView in order to perform insert, delete and view operations. After adding 1 EditText, 1 Button and 1 TextView, your activity_main.xml will look like this.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/Input"
android:layout_width="320dp"
android:layout_height="40dp"
android:hint="Input your text here!"
android:layout_centerHorizontal="true"
android:layout_marginTop="150dp"
/>
<Button
android:id="@+id/Submit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Submit"
android:layout_below="@id/Input"
android:layout_centerInParent="true"
android:layout_marginTop="50dp"
/>
<Button
android:id="@+id/Output"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Fetch"
android:layout_below="@id/Submit"
android:layout_centerInParent="true"
android:layout_marginTop="120dp"
/>
</RelativeLayout>
We have successfully coded the frontend part of our application. This is how our application will look.
Now its time to write the backend code which helps us to do the main operations. Open up the MainActivity.java file. First of all, create objects of the views we created in our frontend part and link them using findViewById method, like this.
public class MainActivity extends AppCompatActivity {
EditText Input;
TextView Output;
Button submit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Input = findViewById(R.id.Input);
Output = findViewById(R.id.Output);
submit = findViewById(R.id.Submit);
}
}
Now lets see how can we insert data with the help of created controls and some code. Create object of FirebaseDatabase and DatabaseReference classes as it provides important methods to access database and perform operations on our database.
public class MainActivity extends AppCompatActivity {
EditText Input;
TextView Output;
Button submit;
FirebaseDatabase firebaseDatabase;
DatabaseReference databaseReference;
String fetchedText = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Input = findViewById(R.id.Input);
Output = findViewById(R.id.Output);
submit = findViewById(R.id.Submit);
firebaseDatabase = FirebaseDatabase.getInstance();
databaseReference = firebaseDatabase.getReference("root");
}
}
Now write an onClick() method for our button so when we press it, it inserts the data we entered in our EditText. Also add a Toast so that we get insert acknowledgment.
submit.setOnClickListener(new View.OnClickListener()
{
@Override
public void onClick(View view)
{
String text=Input.getText().toString().trim();
databaseReference.push().setValue(text);
Toast.makeText(getApplicationContext(),"Inserted!",Toast.LENGTH_LONG).show();
}
});
We have successfully written the logic of insert operation. Now lets see how can we perform read operation from our database. For read operation, let us create a new function fetchData() which retreives data from our database.
private void fetchData()
{
databaseReference.addChildEventListener(new ChildEventListener() {
@Override
public void onChildAdded(@NonNull DataSnapshot snapshot, @Nullable String previousChildName) {
String str = snapshot.getValue(String.class);
fetchedText += str;
}
@Override
public void onChildChanged(@NonNull DataSnapshot snapshot, @Nullable String previousChildName) {
}
@Override
public void onChildRemoved(@NonNull DataSnapshot snapshot) {
}
@Override
public void onChildMoved(@NonNull DataSnapshot snapshot, @Nullable String previousChildName) {
}
@Override
public void onCancelled(@NonNull DatabaseError error) {
Toast.makeText(getApplicationContext(),"Error: "+error.toString(),Toast.LENGTH_LONG).show();
}
});
}
The addChildEventListener() method helps us to fetch child nodes from the provided reference.
Testing the application
We have successfully coded our application. Now its time to check the working of our coded application. Lets insert some dummy data to our input.
- Inserting Lorem as input.
- Inserting Ipsum as input.
As you can see we got acknowledgement that we have successfully inserted text to our database. Now lets check our firebase realtime database.
Now lets test our fetch data operation. You simply need to click on Fetch button.
Thus, we got the data we provided in a toast message. We have now successfully tested our application and its working perfectly fine.
With this article at OpenGenus, you must have the complete idea of Integrating android application to firebase real-time database.