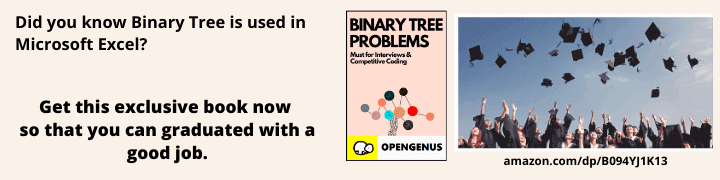
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We always think that giving interview is hard,but with right approach and preperation nothing is impossible. The magic doesn't happen in a day, you should PRACTICE and WORK enough. We all are on the journey of finding better opportunity and this article get's you covered of few aspects!
We are going to walk through the commonly asked questions in technical interview. In this article we will focus on sorting algorithms.
Areas to take a close look into:
- Time complexity.
- Space complexity.
- Data structures used in each algorithm.
- Psuedocode
- When to use which algorithm
This is just an overview of in which areas questions will be asked. Take the fun quiz and** practice ahead** !!
If you had to knock out an algorithm among those listed below, which one would it be?
Which algorithm would you prefer when the input is uniformely distributed?
If you had to choose Bubble sort for a reason why it would it be?
MinHeap is built as a part of Heap Sort Algorithm, which makes it more efficient.
The above statement is?
What is the best case complexity of the Selection Sort?
What is the disadvantages of merge sort?
How do you identify in-place algorithms?
According to you what is the exact logic used by the radix sort?
The worst case time complexities of shell sort and heap sort are:
You have an array which is already sorted. But, if you want to make sure, then which algorithms will do your task?
Which of the following two algorithms have same average case complexity?
1. Merge Sort,2. Selection Sort,3. Insertion Sort,4. Quick Sort,5. Bubble Sort ,6. Shell sort
Why do you use heap for sorting(like in heapsort)?
Descriptive Questions can be:
- About the logic used in the algorithm.
- Application of the algorithm, when given an array/collection to sort it.
- How would you implement the algorithm without using the particular data structure(typical question on some algorithms)
We just took a look at a dozen of questions as a part of practice. But, this is not enough!. Learn, practice, solve!
Bonus Tip !
- Before going to the interview ,we keep on worrying about the technical stuff we don't know. But the primary need to face the interview is the good mindset.If you are nervous and not confident then, even if we know each and every thing we won't be able to convey it to the interviewer. Believe in yourself and be confident.
- Don't loose hope if you get rejected.Sometimes,things don't get together.But it doesn't mean that you should stop integrating it.NEVER GIVE UP. Remember you are ment for higher things!!
There are some cool resources in OpenGenus, which you might end up enjoy reading it! Fire up the links below :)
Resources
- Comparison b/w Different Advanced Sorting algorithms. Level up your interview!
- Comparison b/w Different Advanced Sorting algorithms. Level up your interview preparation!
- Get your hands dirty in "C Programming language" by practicing interview questions.
Best wishes for your endeavours of life.