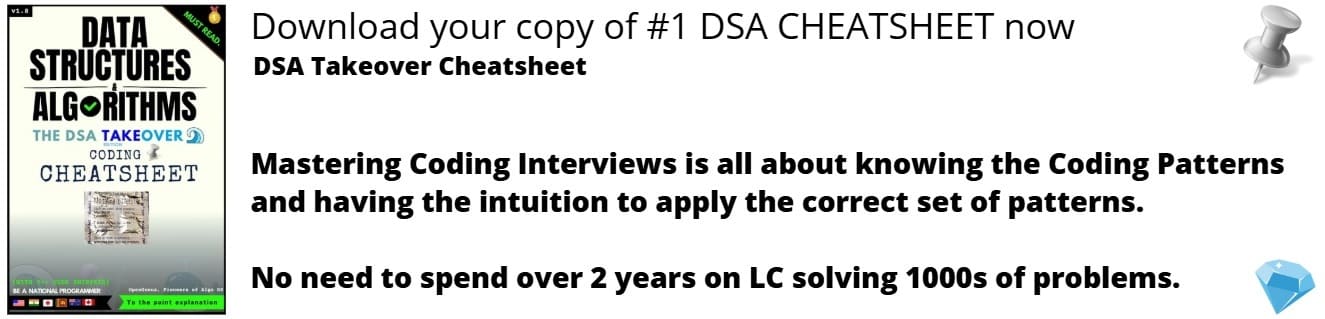
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to discuss different methods to iterate a String word-by-word using C++ libraries/techniques.
Table of content:
- Introduction of the problem
- Methods
i. Solving by sstream standard library
ii. Using User define character iteration
iii. Using getline function
iv. Using Split from Boost(External library)
v. Using cstring library (strtok) - Applications
- Conclusion
Introduction
In programming, we mostly deal with arrays and strings. so, it is the basic requirement to iterate strings and arrays. Althougt iterating arrays and strings (character by cahracter) are quite easy. But if we are asked to iterate an string (word by word) than we might need to scratch our head a bit. It is not a hard concept and it is perfectly fine if you know your way around this by some other method but it is always good to know more than one method to solve a problem. So, hereby we are going to practice few methods to iterate strings word by word in c++.
Methods
The different methods to Iterate String word by word are:
i. Solving by sstream standard library
ii. Using User define character iteration
iii. Using getline function
iv. Using Split from Boost(External library)
v. Using cstring library (strtok)
Solving by sstream standard library
To implement this method we simply need to include sstream library. The main purpose of this library is to put string in stream exactly like cin and then we can extract words one-by-one.
Syntax
istringstream ss(str)
ss>>wordss -> identifier of stream
str ->input string
word ->variable to store word from stream
Example
code:
istringstream ss("split me!")
output:
Implementation in C++
#include<iostream>
#include<sstream>
#include<string>
using namespace std;
int main(){
string s = "Contribute to the community";
istringstream iss(s); //or use stringstream
string word;
while(iss>>word){
cout<<word<<endl;
}
return 0;
}
Output:
Contribute
to
the
community
Explanation
- In the above code we used istringstream iss(s), here s is the string input and iss is the stream identifier.
- After the assignment we have the stream iss which contains the string s.
- use of >> operator passes the first word ,seperated by delimeter, of stream to the word variable. now in stream the first word has been deleted (just like we pop an element from a stack)
- similarly we will get the rest of words by looping the stream.
Using User define character iteration
In this method we are basically iterating through each character and storing that character in a variable.
Implementation in C++
#include<iostream>
#include<sstream>
#include<string>
using namespace std;
void split_string(string text)
{
int i=0;
char ch;
string word;
while(ch=text[i++]){
if (isspace(ch)){
if (!word.empty()){
cout<<word<<endl;
}
word = "";
}
else{
word += ch;
}
}
if (!word.empty()){
cout<<word<<endl;
}
}
int main(){
string s ="contribution is the gift for developers";
split_string(s);
return 0;
}
Output:
contribution
is
the
gift
for
developers
Explanation
- This program is simply based to direct iteration of string (char-by-char).
- Here each character is compared with the desired seperator, if the character is not the seperator, we will append it in a word variable.
- if we find the character equal to seperator we will print the previous stored value in word variable.
- keep reperating the loop till the length of string.
Note:
There is no bold reason to discuss complexity of this program as it is the simple looping which runs for n(no. of words) iterations. but if we were asked to get ith word then we must need to think the process by keeping in mind of multiple queries.
Using getline function
Implementation in C++
#include<iostream>
#include<string>
#include<sstream>
#include<vector>
using namespace std;
vector<string> split(const string &s, char delim) {
vector<string> elems;
stringstream ss(s);
string item;
while (getline(ss, item, delim)) {
elems.push_back(item);
}
return elems;
}
int main() {
vector<string> x = split("A line to split",' ');
unsigned int i;
for(i=0;i<x.size();i++)
cout<<i<<":"<<x[i]<<endl;
return 0;
}
Output
A
line
to
split
Explanation
The getline function in the above code is handling an stream of data and deciding
to break the flow of stream whenever a delimeter seperatror is encountered in the stream.
Using Split from Boost(External library)
Syntax
boost::split(store,input,boost::is_any_of(st))
store -> vector to store output
input -> to store input
is_any_of -> spliting function
st -> desired spliting delimeter
Example
code:
boost::split(store,input,boost::is_any_of(" "))
input="split the text"
output:
split
the
text
Implementation in C++
#include <bits/stdc++.h>
#include <boost/algorithm/string.hpp>
using namespace std;
int main()
{
string input("Remember what to say?");
vector<string> result;
boost::split(result, input, boost::is_any_of(" "));
for (int i = 0; i < result.size(); i++)
cout << result[i] << endl;
return 0;
}
Output
Remember
what
to
say?
Explanation
The above code is an utility of External library of c++ boost . In this libarary there is a seperate class defined to handle spliting.
- The above function splites the passed string on matched delimeter and store the resultant array of strings in passed vector.
Using cstring library
syntax
strtok(string,deli)
string -> string to be split and iterate
deli -> seperator character
Example
code:
strtok(string,deli)
string="Hello Hello World!"
deli=" "
output:
Hello
Hello
World!
Implementation in C++
#include <cstring>
#include <iostream>
using namespace std;
int main() {
char quote[] = "Remember me when you look at the moon!";
// break the string when it encounters empty space
// str = quote, delim = " "
char* word = strtok(quote, " ");
cout << word << endl;
while(word=strtok(NULL," ")){
cout<<word<<endl;
}
return 0;
}
Output
Remember
me
when
you
look
at
the
moon!
Explanation
The above code uses cstring library which is an c-library. This code uses a mix concept of 1 and 3 method.
- Firstly This code works exactly like the previous code but we need to give an
extra attention to the NULL parameter. - the concept of this method is very simple we only need to pass the string and the desired seperator but it in single execution it returns only the front word. now for execution second time we need to put NULL parameter instead of string because it already contains the remaining stream of string.
Note:
if we put string instead of NULL in second execution this function will again return the first word of original string.
Applications
- It can be used for tokenization in NLP(Natural processing language).
- In parsers.
- In sanatization of data retrival
- In word counting Problems etc.
Conclusion
Above discussed methods are the most popular methods but there are many more methods to work around this problem. Despite that the basic structure of all the methods is like above discussed. We can make some alteration by using different storage structures or using some different character iteration techniques.