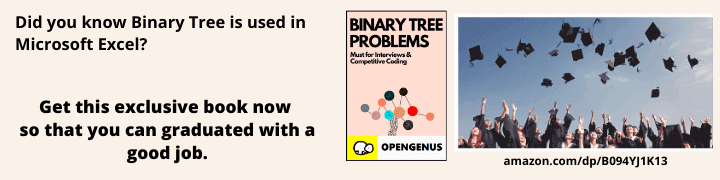
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented how to use if else elif as a part of Lambda function in Python. We have covered different cases like if, if else and if else elif with Python code examples.
Table of contents:
- Lambda with if else
- Lambda with if without else
- Lambda with if else elif
- Concluding Note
Lambda with if else
Lambda always takes in a single line expression and if else will involve multiple lines and is not a simple expression by default. This is how if else looks like in Python:
if a > b:
return a
else
return b
There is an alternative in Python which allows if else to be written as a single line expression like:
a if a>b else b
As this is one line expression, this can be used in Lambda. Following Python code demonstrates how to use if else in Lambda in Python:
f = lambda a, b: a if a>b else b
maximum = f(50, 95)
print(maximum)
Output:
95
Lambda with if without else
Consider the following Lambda without else part of if:
f = lambda a, b: a if a>b
maximum = f(50, 95)
print(maximum)
This will give the following compilation error:
File "code.py", line 1
f = lambda a, b: a if a>b
^
SyntaxError: invalid syntax
This is because the one line alternative of if else has the else part as mandatory. Hence, it is not possible to create a Lambda in Python with only if part that is without else part.
As an alternative, place a default value or NoneType in else part of the Lambda function in Python.
Lambda with if else elif
The idea of using if else elif in Lambda is to add another if else part in the else part of the one line alternative of if else in Python. Consider the following if else elif code in Python:
if a>b:
return a
elif b>c:
return b
else
return c
This can be written as a one line expression in Python as:
a if a>b else b if b>c else c
This can be used in Lambda. Following Python code demonstrates the idea of using if elif else in Lambda in Python:
f = lambda a, b, c: a if a>b else b if b>c else c
maximum = f(50, 95, 91)
print(maximum)
Output:
95
Concluding Note
In this article at OpenGenus, you must have the complete idea of how to use if else in Lambda in Python.
This allows to add conditional checks in Lambda and this makes it even more powerful.