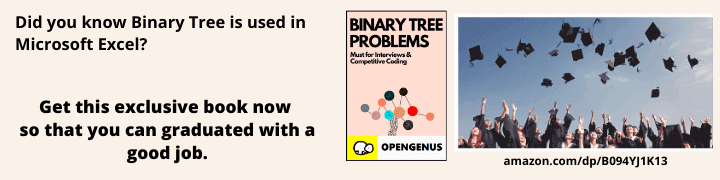
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have presented 3 approaches to use lambda to get the sum of all elements of a list along with complete Python code.
Table of contents:
- Lambda to sum 2 elements
- Lambda to get sum of a list using reduce
- Pure Lambda to get sum of a list
Lambda to sum 2 elements
Adding 2 elements using lambda in Python is straight forward. The lambda takes in two input parameters and returns the sum of the two elements. Following Python code demonstrates the process:
f = lambda x, y: x+y
sum = f(2, 9)
print(sum)
Output:
11
The challenge is to get the sum of all elements of a list using lambda.
Lambda to get sum of a list using reduce
We can leverage the above lambda to get the sum of two elements to find the sum of all elements of a list. The solution is to use reduce() built-in functions in Python.
reduce() built-in function applies the same function on all elements of an iterable object like list. This can be used to apply the previous lambda on each element of the list.
Following Python code using lambda explains the concept:
from functools import reduce
list = [59, 47, 7, 17, 45]
f = lambda x, y: x+y
sum = reduce(f, list)
print(sum)
Output:
175
Reduce applies the lambda on the first two elements followed by the result as the first element and the third element as the second element in lambda and so on. This explains the concept/ order in which the lambda is applied using reduce():
((((59, 47), 7), 17), 45)
This lambda in Python helps us find the sum of all elements of an iterable.
Pure Lambda to get sum of a list
It is possible to avoid the use of reduce(). The key is that:
- If size of list is 1, then the sum is same as the first element.
- Else, add the first element of the list to the sum of the rest of the list which is calculated using the lambda.
Following Python code using lambda explains the concept:
list = [59, 47, 7, 17, 45]
f = lambda l: l[0] if len(l) == 1 else l[0] + f(l[1:])
sum = f(list)
print(sum)
Output:
175
This is a pure approach of using only lambda to find the sum of all elements in an iterable.
Concluding Note
With this article at OpenGenus, you must have the complete idea of how to get the sum of all elements of a list using Lambda.