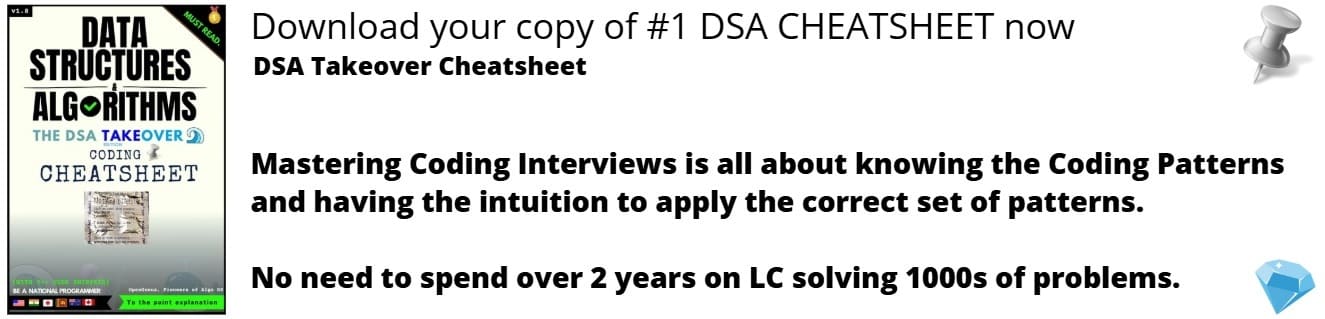
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes
In this article, we have explored the basic ideas behind Restful API along with its five principles and illustrate it with an example of Twitter API.
⭐ What is an API ?
API stands for Application Programming Interface. It is a piece of software that can be used by another piece of software , in order to allow applications to talk to each other.
REST(Representational States Transfer) Architecture is the most popular architecture for building APIs.
⭐ REST ?
It stands for Representational States Transfer.
It is an architecture style for designing loosely coupled applications over HTTP, that is often used in the development of web services. REST does not enforce any rule regarding how it should be implemented at lower level, it just put high level design guidelines and leave you to think of your own implementation.
In simple words, it is basically a way of building web APIs in a logical way such that it becomes easy and hassle-free for the users.
⭐ 5 Priciples of REST-
We have 5 priciples to build RESTful APIs(APIs following the API architecture).
Now. let's go through the priciples one by one -
📝 1. Seperate API into logical resources.
The key abstraction of information in REST is a resource, and therefore all the data that we want to share in the API, should be divided into logical resources.
**Resource **- A Resource is an object or representation of something, which has data associated to it.
For intances users
, recipes
, tours
, games
etc.
It just has to be a name though, and not a verb.
📝 2. Expose structured,resource-based URLs.
Now, we need a structured URL which will be used by the client to fetch the data.
For instance, www.mywebsite.com/users
, here www.mywebsite.com/users
is the URL, while /users
is the endpoint. An API can have many endpoints just like the ones in the diagram below -
Each of these endpoints can send different data back to the client or can perform different actions.
Now, the endpoints may seem fine to us, but they violate our 3rd priciple i.e we should only use the HTTP methods in order to perform actions on data.
📝 3. Use HTTP Methods(verbs).
The principle basically states that the endpoint should contain our resources and not the actions to be performed.
Here's how these endpoints should actually look like. Say for instance, we have an endpoint /getRecipe
. So this /getRecipe
endpoint is to get data about a recipe.
Here, we should simply name the endpoint /recipes
and send the data whenever a GET request is made to this endpoint.
So in other words, when a client uses a GET HTTP method to access the endpoint.
And similarly, we should always opt to keep resources as the endpoint. Also, it's a common practice to always use the resource name in plural which is why we wrote /recipes
here and not /recipe
.
In case, we only want the recipe with one I.D., let's say 15, we add 15 after another slash - /recipes/15
.
Or it could also be the name of a recipe instead of the I.D., or some other unique identifier.
GET - The first HTTP method or verb that we can respond to is GET and it's used to perform the Read operation on data.
POST - If the client wants to create a new resource in our database, in this example, a new tour, the POST method should be used.
What's really important to note here is how the endpoint name is the exact same name as before.The only difference is really the HTTP method that is used for the request. If the /recipe
endpoint is accessed with GET
, we send data to the client. But if the same endpoint/recipe
is accessed with POST
, we expect data to come in with a request, so that we can then create a new resource on the server side.
This is the beauty of using HTTTp methods, instead of verbs in the endpoint.
PUT AND PATCH -We use PUT or PATCH to update our data.
The difference between them is that with PUT, the client is supposed to send the entire updated object, while with PATCH, it is supposed to send only the part of the object that has been changed. But both of them have the ability to send data to the server.
DELETE - To delete a resource, we have the DELETE http method. Again, the I.D. or some other unique identifier of the resource to be deleted should be part of the URL.
These were the five HTTP methods that we generally respond in a RESTful API, enabling the user to perform basic CRUD operations (Create,Read,Update,Delete).
📝 4. Send data as JSON (usually).
Now, about the data that the client actually receives, or that the server receives from the client, usually, we use the JSON Data Format. And so let's briefly learn what JSON actually is and how to format our API responses. JSON is a very lightweight data interchange format which is heavily used by web APIs coded in any programming language.
JSON is based on the JavaScript object notation.Its syntax is derived from JavaScript object notation syntax:
- Data is in key/value pairs (key->value)
- Data is separated by commas
- Curly braces hold {objects}
- Square brackets hold [arrays]
Key-value pair :
Syntax :
"field-name" : value
For instance,
The field-name is always a string wrapped in double-quotes.
"name" : "Priyanka"
JSON Objects :
Syntax :
{"field-1" : value, "field-2":value}
It is similar to a JavaScript object. The keys are always string, while the value can be of any data type (string, number, array or even another object).
For instance,
{
"name":"Priyanka",
"_id":14
}
Note: The key-value pairs inside the object are seperated with commas.
JSON Arrays :
Syntax :
[data-1,data-2,data-3]
In JSON, array values/data must be of type string, number, object, array, boolean or null.
For instance,
var obj = ["Priyanka", 14, true]
The elements of an array can be accessed using index number, For eg.
console.log(obj[1])
will give
14
as output.
📝 5. Be stateless.
A RESTful API should always be stateless. So, what does stateless actually mean?
Well, in a stateless RESTful API, all state is handled on the client and not on the server. And state simply refers to a piece of data in the application that might change over time.
For example, whether a certain user is logged in or
The fact that the state should be handled on the client means that each request coming from the client, must contain all the information that is necessary to process a certain request on the server. So that the server should never have to remember previous requests in order to process the current request.
In simple words - statless means No client session on the server.
For instance, while reading an ebook, the user is on Page 5, and wants to move to Page 6. One of the approach can be to send a request with endpoint similar to ebook/next-page
.
But in this case, the server would have to figure out what the current page is and based on that send the next page to the client.
In other words, the server would have to remember the previous request. It would have to handle the state server side and that is exactly what we want to avoid in RESTful APIs.
Instead, we can create an endpoint such as ebook/page
and replace the page
with the next page number. This way, we store the state (current page in this case) in client-side, and hence server need not remember any session info of the client.
⭐ Example of REST API: Twitter API
Let's see a real world example of REST API. We will see Twitter API to understand the REST API architecture.
For instance, it provides us with GET lists/list
which returns all lists the authenticating or specified user subscribes to, including their own.
The resource URL for the same is - https://api.twitter.com/1.1/lists/list.json
.
Now, let'see how Twitter API implements the REST architecture. Head over to Twitter API Reference Index , and you may notice, that the data has been divided into logical resources such as lists, users, account, friendships, blocks, collections etc.
Hence, our first point i.e Seperate API into logical resources.
Twitter also provides us with structured- URLS as https://api.twitter.com/1.1/lists/list.json
is the URL, but /lists
is the endpoint which refers to subscribed users.
As you may have noticed, we do not have endpoints named as getLists
or postLists
, instead we have used HTTP methods everywhere. For instance -
- GET lists/list
- GET lists/members
- GET lists/members/show
- POST lists/create
- POST lists/destroy
- POST lists/members/create
Also, the data is sent in the json format, as in the URL - https://api.twitter.com/1.1/lists/list.json
, we are accessing the file that contains JSON data (list.json ).
Twitter also saves the data on the client-side, making it stateless, which is our fifth point.
And we're all done ! 🎉