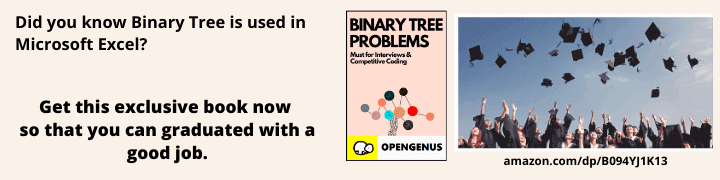
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
C++ is a vast language and it houses many data types just for the ease of the end user. Struct and Class are two of them. In this article, we have covered the points of differences between them.
Table of Contents:
- Recap (Structures and Classes)
- Difference between struct and class in C++
- Myths and Misconceptions about struct in C language and class in C++
Recap : Structures
A structure is a user-defined data type which is used to group items of same or different data types into a single type. It is declared using the keyword "struct".
Recap : Class
A class is also a user-defined data type which holds its own data members and member functions, which can be accessed and used by creating an instance of that class (called object). It is defined using the keyword "class".
Difference between class and struct in C++
Although, both class and struct are user defined data types and are similar in many ways, they both differ significantly. In this section, we will see the points of difference between class and struct in C++.
Struct | Class |
---|---|
Declared using keyword "struct" | Declared using keyword "class" |
In case of no access specifier, all members are public by default |
In case of no access specifier, all members are private by default |
During Inheritance, the members of the base class are inherited in public mode by default |
During Inheritance, the members of the base class are inherited in private mode by default |
Differences in detail
- One of the major point of diffence between these two are that the members of a class are private by default while on the other hand the members of the structure are public by default.
Example of class- CPP Program
#include <bits/stdc++.h>
class c1
{
// By default the access specifier is private in class
int n; // private data member of the class
public: // public access specifier
int roll; // public data member
};
int main()
{
c1 b; // creating an object of class c1
b.n=5; //this statement gives a compiler error as "name" is private
return 0;
}
Output:-
[Error] 'int c1::n' is private
Example of struct- CPP Program
#include <bits/stdc++.h>
struct s1{
int n; // by default n is public
};
int main()
{
s1 s;
s.n=5; // compiles successfully as x is public
std::cout<<"The value is=>"<<s.n;
return 0;
}
Output:-
The value is=>5
- Another major difference between them is that during inheritance , the class keyword inherits the members in private mode, while the struct keyword inherits the members in public mode by default. It is to be noted that the private members of the base class cannot be inherited by the derived class.
Inheriting from class- CPP Program
#include <bits/stdc++.h>
class Parent
{
// by default the access specifier is private in class
int n1; // private data member of the class
public: // public access specifier
int roll; // public data member
};
class Derived:Parent{ //Inheriting from the Parent class in private mode (by default)
int n2; //private member of Derived class
};
int main()
{
Derived obj; // creating an object of class Derived
obj.roll=5; //this statement gives a compiler error as "roll" is private member of Derived class
return 0;
}
Output
[Error] 'int Parent::roll' is inaccessible
Inheriting from struct- CPP Program
#include <bits/stdc++.h>
#include <iostream>
struct Parent
{
// by default the access specifier is public in structure
int roll; // public data member
};
struct Derived:Parent{ //Inheriting from the Parent struct in public mode (by default)
int n; //public member of Derived struct
};
int main()
{
Derived obj; // creating an object of struct Derived
obj.roll=5;
std::cout<<"The accessed value is=>"<<obj.roll;
return 0;
}
The above code compiles successfully and the result is as follows:-
Output
The accessed value is=>5
The above mentioned points are the points of difference between the two data structures in C++.
Important Note to the reader
The below mentioned points are the difference between struct in C and class in C++ language and are therefore not to be confused with class and struct in C++. (C language do not support classes).
Struct in C | Class in C++ |
---|---|
Cannot contain member functions | Can have both data members and member functions |
Inheritance is not allowed | Inheritance is allowed |
Variables cannot be initialized during structure declaration | Variables can be initialized during class declaration |
Null values are not possible | Null values are possible |
It may have only parameterized constructor | It may have all types of constructors |
- Structure(in C) cannot contain member functions unlike a class(in C++), which can contain both data members and member functions.
- Structures cannot be inherited in C language but can be inherited in C++.
The following C program demonstrates this point:
C program
#include <stdio.h>
struct Parent
{
// by default the access specifier is public in structure
int roll;
};
struct Derived: Parent{ //gives an error as C doesn't support inheritance with structures
int n2; //public member of Derived struct
};
int main()
{
struct Parent obj; // creating an object of struct Parent
return 0;
}
- During the declaration of a structure(in C), variables cannot be initialized while it can be done in the case of classes and structures(in C++).
The following C program demonstrates this point:
C program
#include <stdio.h>
struct Parent
{
// by default the access specifier is public in structure
int roll=7; // gives an error as initialization in a structure is not supported in C
};
int main()
{
struct Parent obj; // creating an object of struct Parent
printf("The accessed value is=>%d",obj.roll);
return 0;
}
Output
[Error] expected ':', ',', ';', '}' or '__attribute__' before '=' token
These were the popular misconceptions about difference in struct and class in C++ and how they are different from the C language.
With this article at OpenGenus, you must have the complete idea of the difference between struct and class in C++ and common misconceptions about it.