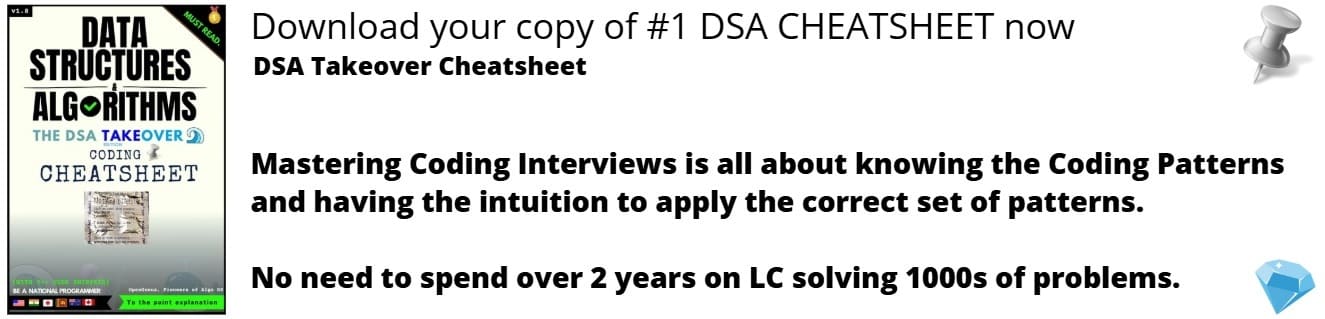
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Structures in C++ are alternative to classes and is useful in designing a program better. In terms of advanced implementation strategy, we can see this as:
Array < Structure < Class
In this, we have covered the following major points:
- Need for structures
- Idea of structures in C++ with code examples
- Array of structures
- Difference between class and structures
Need for Structures
So, to begin with we know that arrays are collection of variables of similar datatypes, they are very handy but often in many real-life situations we usually handle data containing a mix of types like int, strings, float all together for example consider the data of a set of students containing roll.no, Section and class, using the concept of arrays we can implement it like this .
int roll[3]={402,403,404};
char section[3]={'A','B','C'};
int class[3]={1,2,3};
The above code works and is okay to use but what if the data gets more complex like names and we want to store first name, middle name and last name seperately we will need more arrays but every time we need to access any data we need to iterate each and every time and still other issues like no relation between the data which results in waste of both computational power and time to add, append, access or remove data hence we need an array kind of structure which needs to store data of different data types thus we need structures in c++.
Structures in C++
Structure can be called as a user-defined data type which can be used to store or group a collection of different data types together.
Defining a structure -basic syntax
struct name
{ member1;
member2;
member3;
};
Struct is the keyword to define a structure and can be named according to our choice , note the semicolon in the end.
Example
#include<iostream>
using namespace std;
struct Rectangle
{
int length;
int breadth;
};
int main()
{
struct Rectangle r={10,5};
cout<<r.length<<endl<<r.breadth;
}
Explanation for above code :
The structure is named as Rectangle and both length and breadth are it's members. To access them or use them in the main program we declared the structure rectangle with a structure variable r and the dot ' . ' operator is used to access and modify the members of the structure rectangle.
r.length=25;
cout<<r.length
We used the name of the structure 'r' to access the data members. This is how Object Oriented concept comes in here 'r' acts like an object and using 'r'(name of the structure) we can access and modify the data realated to the structure easily. One more advatage is that once a structure is defined it can be re-used multiple times as needed just by creating as many as we need.
Array of Structures
Whoa! this is something huge but wait I just thought that structures act as replacement for arrays but why array of structures ?
It's the same reason we needed arrays multiple variables to store more data like we discussed in the beginning what if I really need to store data of fifty students now each of them having a different structure name would definately make things more complex hence we need and array of structures.
Program
#include<iostream>
using namespace std;
struct Student
{
int rollno;
char section;
int class;
};
int main()
{
struct Student stu[3]={
{401,'A',1},
{403,'B',2},
{404,'C',4}
};
cout<<deck[0].face<<deck[1].face<<deck[2].color;
}
Output :
All Student details below
Student roll.no :401
Student section :A
Student class :1
Student roll.no :403
Student section :B
Student class :2
Student roll.no :404
Student section :C
Student class :4
Explanation for above code :
Now using an array of structures is almost similar normal arrays where we access and modify using the index, this gives us a lot of flexilbility and help in re-using structures without multiple declarations.
So, strutures almost sound like classes and object.
What is the difference between stuctures and classes ?
A lot. Classes are much adavanced and complex, stuructures might be just a bit more advanced than arrays. There are a lot of differences between their purpose and implementation here are a few:
Inheritance
Classes can help define other classes using inheritance, this is a great advantage and helps in re-using the code and extedning the usage and access of classes.
Although structures can be re-used multiple times they don't offer inhertance features that classes offer.
Functions and Constructors
Functions in classes help us in working on the member data funtions and this entirely changes the way how we intreact with the data members.And the use of constructors takes this to the next level to perform more complex operations on the data members. We can include functions in structures however we can't inlcude constuctors in structures.
Data Abstraction
Classes are more complex and using the concept of data abstration and access specifirers makes it difficult to access class data members whereas a structure can be accessed and modified very easily just by knowing it's name or variable.
Conclusion
There are many differeneces like structure is created in stack memory and class is created in heap memory. Classes are very complex and implement the concept of object oriented programming to the core while stuctures are just a little ahead of arrays.
With this article at OpenGenus, you must have the complete idea of Structures in C++. Enjoy.