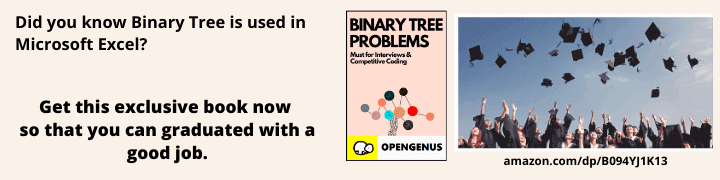
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will talk about webockets, it's a very important and fundamental topic for anyone interested in web and communication protocols. WebSocket is a computer communication protocol, providing full connections over a single TCP connection.
Table of contents:
- How HTTP works? (http:// , https://)
- How does websocket work? (ws:// , wss://)
- When to use websockets?
- Pros of using websockets
- Cons of using websockets
- Implementing a websocket in Python
- Popular WebSocket libraries
- Conclusion
Before we dive deeper into how it works, let’s discuss briefly on how HTTP works to know why we have web sockets!
How HTTP works? (http:// , https://)
Consider having a client and a server, the following flow will show us how the communication occur :
- The client opens a TCP connection to communicate with the server.
- The client sends a request to the server for example a GET request for an index.html file
- The server responds to that request with a certain status code such as:404 not found or 201 ok.
And this flow continues until the client closes the connection. We can conclude here that this protocol is unidirectional as we can’t receive a response without sending a request and vice versa.
Now we knew how http works, but in many situations this protocol will overhead, imagine we are having an application like facebook, while you are browsing there’s notifications maybe pop up, guess :
Is it good to be fetched using HTTP?
Here you as a client don’t know that the resource is modified because another user modified it or the service itself modified it, so in this case using HTTP is not the best thing, HTTP will be preferred when a resource that changes hourly or changes only after it knows that a related resource is modified, so that we need something STATEFUL.
How does websocket work? (ws:// , wss://)
Having the same client and server :
- The client opens a TCP connection.
- A websocket handshake request occurs, this is a normal http request with upgrade header that asks the server whether to establish a websocket connection or not.
- If it’s ok:
- The client now is able to send and receive information to and from the server without waiting, then this is a bidirectional protocol.
- If anyone closes the connection, the other will not be able to send information and the connection is terminated from both sides.
Hanshake Protocol
Briefly the handshake protocol allows the client and server to agree upon an encryption method and verify the client and server before any data is sent between them.Then they can exchange encryption keys and data between them.
When to use websockets?
There are some scenarios where websockets are preferred over HTTP such as Fast Reaction Time, Ongoing Updates.
It’s used in:
-
Chat Applications
*Consider a chat application that allows multiple users to chat in real-time. If WebSockets are used, each user can both send and receive messages in real-time and no one needs to wait for the other response.
Anyone can send you a message without you requesting to receive it!
Imagine you are chating with a friend and every time you refresh the page to get the new messages, tho you maybe don't know wether he sent a message or not.. that is very draining.... -
Real time Applications
- Consider a notification system in a feed application as we talked earlier.
- Sports updates applications such as live scores, stats of a current match.
- Editing a document with other collaborators at the same time while watching others activities and modifications, such as in the online google docs.
-
Gaming Applications
- Consider having a multiplayer game running in the browser which its ui is automatically updated upon the current state.
Pros of using websockets
Pros of using websockets are:
- Full duplex
- No polling required and we don’t have to ask for a response repeatedly.
Polling is a technique by which the client asking the server for new data regularly.
- No polling required and we don’t have to ask for a response repeatedly.
- Moderate overhead in establishing connection due to the http request that occur at the handshaking step, and minimal overhead in sending/receiving messages.
Cons of using websockets
Cons of using websockets are:
- Since it’s stateful it’s difficult to be horizontally scaled.
- Web browser must be running HTML5.
- Edge caching is not possible.
Implementing a websocket in Python
We will use python and js to write a very simple websocket to establish connection between a created server and the browser client side.
First of all, implement the server in python language that recieves a messages and instantly reply to it.
Don't forget to "pip install websockets"
Create a folder and place this script file in it.
import asyncio
import websockets
# create handler for each connection
async def handler(websocket, path):
data = await websocket.recv()
reply = f"Client:{data}\nServer:I am fine, thanks!"
await websocket.send(reply)
start_server = websockets.serve(handler, "localhost", 7000)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
Second, implement the client side using html and js
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Client</title>
</head>
<body>
<button onclick="contactServer">How are you?</button>
</body>
<script>
const socket = new WebSocket('ws://localhost:7000');
socket.addEventListener('open', function (event) {
socket.send('How are you?');
});
socket.addEventListener('message', function (event) {
console.log(event.data);
});
const contactServer = () => {
socket.send("Initialize");
}
</script>
</html>
After running the file and opening the HTML page, the results as follow
this was a very simple example that you can expand on your own 😉.
Popular WebSocket libraries
Popular WebSocket libraries are:
- Socket.io.
- WS.
- Sockjs.
- Feathers.
Conclusion
Finally, each technology has its pros and cons and using websockets or http depends on the nature of your application and the requirements needed.
If you only want to receive an update every hour, you will want to go with HTTP. If you want to receive updates every second, a WebSocket might be a better option, because establishing an HTTP connection takes a lot of time.
WebSockets has a lower cost, whereas HTTP REST based protocols is comparatively higher on the cost. With this article at OpenGenus, you must have the complete knowledge of Web Sockets.