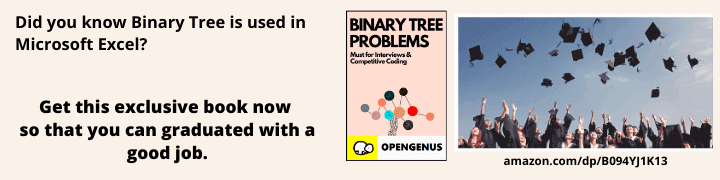
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
A-frame is an open-source web framework for building Virtual Reality(VR) applications. It is used for a well-known javascript library known as "Three.js". It allows developers to create 3D and virtual reality experiences using HTML.
A-Frame was originally developed within the Mozilla VR team during 2015. It was released on December 16, 2015. A-frame creates scenes that works across desktops and smartphones.
A Basic example from A-frame is:
<a-scene>
<a-cube
color="#0095DD"
rotation="20 40 0"
position="0 1 0">
</a-cube>
<a-sky color="#DDDDDD"></a-sky>
</a-scene>
In the above example:
- There is a basic shapes cube created using "a-cube" tag of HTML
- We use "a-sky" for the background color.
Output for the above example
A-Frame is a framework that make use of Entity Component System(ECS). It is a three.js framework that is capable enough to do almost anything that three.js can do. In ECS, entity is referred to as every object in scene and components are plugged into entity which brings appearance, behaviour and functionality. For example, we have color, tire and engine components for a car, these can be used as entities and we can compose it by configuring and mixing different components.
In A-Frame -
- An entity is represented by "a-entity".
- A component is represented by an attribute(e.g. "a-entity engine" tag)
- If a component has only one property to define, then it looks like a normal HTML attribute (e.g. a-entity visible="false" tag).
- If a component has more than one property to define, then properties are passed in through a syntax similar to inline CSS styles (e.g. a-entity engine="cylinders: 3; horsepower: 150; mass: 180" tag).
Some of the features for A-Frame are:
- Virtual Reality made simple.
- HTML is easy to read and understand.
- It uses Entity Component System.
- Performance is a top priority.
- Applications built are cross platform.
- It provides a visual 3D inspector.
We can include A-Frame in our application by npm or yarn :
To install aframe using npm, use the following command:
npm install aframe --save
To install aframe using yarn, use the following command:
yarn add aframe
then,
require('aframe');
To include A-Frame in your application just inlcude
<script src="https://aframe.io/releases/0.7.0/aframe.min.js"></script>
in between the <head></head>
tags.
A-Frame Text Component
A-Frame uses "a-text" tag to add a text. Adding text is as simple as adding a value to a text component. However, there are lots of other values that can be changed as well.
For example:
<a-text
value="Hello"
position="0.05 0.80 -2"
rotation="0 180 0"
font="mozillavr"
color="#e43e31"
side="double"
align="center"
width="6">
</a-text>
A-Frame animation component
A-Frame uses "a-animation" tag to animate the text. It is possible by nesting this component with the component we want to animate. We can also animate multiple objects. For this we must choose a property which we want to animate. Suppose we want to bounce our text up and down.
For this, we will write:
<a-animation
attribute="position"
to="0.05 0.85 -2"
dur="1000"
direction="alternate"
easing="ease-in-out"
repeat="indefinite">
</a-animation>
Text + Animation
Using A-Frame, we can animate text as well. For this, we need to add a-animation tag within a-text tag. The whole text and animation will be :
<a-text
value="VR Gallery"
position="0.05 0.80 -2"
rotation="0 180 0"
font="mozillavr"
color="#e43e31"
side="double"
align="center"
width="6">
<a-animation
attribute="position"
dur="1000"
direction="alternate"
to="0.05 0.85 -2"
easing="ease-in-out"
repeat="indefinite">
</a-animation>
</a-text>
Some of the different attributes for A-Frame are:
- "direction" - It tells the direction of the animation(between from and to). Some are- normal, reverse, alternate, alternateReverse. Default value is normal
- "dur" - It tells the duration of the animation in milliseconds. default value is 1000.
- "fill" - It determines the effect of animation when not active. Some of them are - bakwards, forwards, none, both. Default value is forwards.
- "from" - It tells the starting value. Default is "current value".
- "to" - It tells the ending value. Default value is "none".
Complete example
We can add multiple components with a-scene tag. A comlete code for giving three shapes with a background and a text printed on it.
<script src="https://aframe.io/releases/0.5.0/aframe.min.js"></script>
<a-scene>
<a-sphere position="0 1.25 -5" radius="1.25" color="#EF2D5E">
</a-sphere>
<a-box position="-1 0.5 -3" rotation="0 45 0" width="1" height="1" depth="1" color="#4CC3D9">
</a-box>
<a-cylinder position="1 0.75 -3" radius="0.5" height="1.5" color="#FFC65D">
</a-cylinder>
<a-plane position="0 0 -4" rotation="-90 0 0" width="4" height="4" color="#7BC8A4">
</a-plane>
<a-sky color="#ECECEC">
</a-sky>
<a-text
value="Hello"
position="0.05 0.80 -2"
rotation="0 180 0"
font="mozillavr"
color="#e43e31"
side="double"
align="center"
width="6">
</a-text>
</a-scene>
Output:
With this, you have the complete basic knowledge of building virtual reality components on the Web using A-Frame. Enjoy.