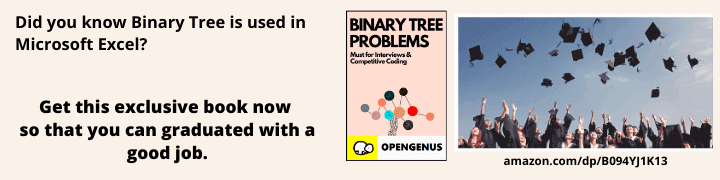
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 5 minutes
Ternary operator in C is an alternative syntax for if else conditional statement. It is usually used to condense if else statements.
Syntax:
condition between the inputs ? value_of_truecomp : value_of_falsecomp
Example:
// following ternary operator
// is calculating maximum among two numbers
maximum = (number1 > number2) ? number1 : number2;
We will go through some basic of operators to get the idea of ternary operator deeply.
An operator is a fuction that perform mathematical or logical operations. There are various types of operators:
- Arithmetic operators
- Relational operators
- Logical operators
- Bitwise operators
- Assignment operators
- Conditional operators
- Special operators
Under the class of conditional operator there exits a ternary operator.
A ternary operator in C is a conditional operator which is used in making various decisions. These decisions are nothing but condition statements that are similar to if and else. The ternary operator takes a minimum of three arguments. The arguments are as follow:
- The first argument is the comparison between inputs(the inputs are compared).
- The second argument is the result of the comparison between the inputs holds which is true.
- The third argument is the result of false comparison.
Syntax
The basic form of syntax that ternary operator follow is:
condition between the inputs ? value_of_truecomp : value_of_falsecomp
for eg:
c = (l1 < l2) ? l1 : l2;
l1 and l2 are the inputs. So the, evaluation of the code takes place if expression1 (a < b) holds true. Otherwise, it is evaluated by expression2 or expression3.
Difference between if/else and ternary operator:
Following is a C Code using if/else statement:
int main()
{
int i=15, j=20, k;
if(i<j)
{
k=i;
}
else
{
j=k;
}
printf("%d", k);
return 0;
}
Code using Ternary operator:
int main()
{
int i=15, j=20, k;
c= (i<j)? i:j ;
printf("%d", k);
return 0;
}
The output for the code will be k=i, because of the conditional operator which is true here i.e, i<j.
As seen in the above two codes for if/else and ternary operator one can see the difference of the length of code. Using if/else the code had more than 10 lines and on the other hand using ternary operator it took a maximum of 6 lines. Also, using ternary operator cuts downtime and line complexity which helps to find and edit errors easily and precisely. Moreover, the code itself is easy to read.
Ternary operator for three variables:
Suppose we are given to find the maximum of the given three inputs. The algorithm for it is:
l = (i > j) ? (i>j ? i:j) : (i>k ? i:k);
The syntax for the ternary operator remains the same. The only addition to it is the conditions in the second and third arguments. Now, (l1>l2) and (l2>l3) must hold as well.
Code for three variable
int main()
{
int i = 3, j= 6, k = 8, l;
l = (i > j) ? (i > k ? j : k) : (j > k ? j : k);
printf("Largest number is %d.", i, j, k, l);
return 0;
}
Difference between nested if/else and nested ternary operator:
Code using nested if-else statement:
int main()
{
int i = 5, j = 8, k;
if (i == 5) {
if (j == 8) {
k = 8;
}
else {
k = 5;
}
}
else {
k = 0;
}
printf ("%d", k);
return 0;
}
Code using nested ternary operator:
int i = 5, j = 8, k;
ans = (i == 5 ? (j == 8 ? 5 : 8) : 0);
printf ("%d", k);
Yet again in the above two codes for nested if/else and nested ternary operator there is a difference of the length of code.
Results and Facts
There always exist the significance of the operators. But, to limit the line of complexity, the number of lines and making code using algorithms, If/else statements can be replaced by the ternary operators because it performs the same as that of the if/else condtion. It changes the overall outlook of the code. Hence, makes it much algorithmic, professional and easy to understand.
But there are some unknowing facts associated of the ternary operator. Let's take a look:
-
Side Effects:
Any side effect associated with expression1 will be changed/updated immediately before executing the of the expression2 or expession3. So, if there are side effects, then only one of them will be evaluated. -
Return Type:
There does exist a return type in the ternary operator. The return type here depends on the convertibility of expression3 to expression2. Otherwise, the compiler throws an error.