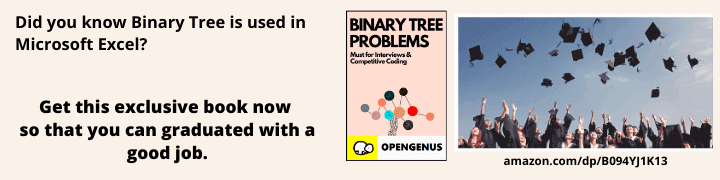
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes | Coding time: 15 minutes
The NASA's Astronomy Picture of the Day (APOD) API helps to get the astronomy picture based on the date that user inputs. We'll walk through the step by step procedure of creating a web application which will display the Astronomy picture of the day using NASA API provided the user gives in a date as input. We made the NodeJS web app using React.
Before starting, you may download the following extension: React Developer Tools by Facebook to analyze the web app you will develop along. It is not a necessary.
We will proceed in steps:
- Preparing the basic NodeJS app
- Making the actual API call to get the image
- Check the details returned by the API
So, lets begin:
Initializing/starting the App -
-> npm init react-app nasa-api
-> cd nasa-api
-> npm start
You can replace "nasa-api" with any name of your choice. Since, this is for the NASA API therefore, I am naming it as nasa-api. This sets up a React app. In your browser you will be redirected to http://localhost:3000/ and see the following screen ->
Now, for getting started, we have to make changes to src/App.js file.
Components
In our App, there will be two main components ->
- Date - It is used to get the date from the user.
- FetchedPhoto - It is used to display the photo fetched from the API.
For this, we have to first create a folder called components in src folder.
-> mkdir src/components
Then, We will have to create a file for each of these components in this folder ->
-> touch src/components/Date.js
-> touch src/components/FetchedPhoto.js
Now, We'll start with a very simple version of our app ->
//App.js
import React, { Component } from "react";
import Date from "./components/Date";
import FetchedPhoto from "./components/FetchedPhoto.js";
class App extends Component {
render() {
return (
<div>
<h1>NASA's Astronomy Picture of the Day</h1>
<Date />
<FetchedPhoto />
</div>
);
}
}
export default App;
//Date.js
import React from "react";
const Date = props => <div>Date</div>;
export default Date;
//FetchedPhoto.js
import React from "react";
const PhotoFromapi = props => <div>FetchedPhoto</div>;
export default FetchedPhoto;
States in our App
The "Date" component will take the date as the input from the user that is used to display the picture from the NASA’s Astronomy Picture of the Day API and display it in the "FetchedPhoto" component.
The "Date" component will update the date which will be used to fetch and update the picture in the "FetchedPhoto" component. Therefore, we will keep the "date" and "FetchedPhoto" components in the App.
We'll add the "date" and "PhotoFromapi" states to our App, and initialize each with empty string ->
class App extends Component {
state = {
date: "",
PhotoFromapi: ""
};
... //The remaining part which we've discussed above
}
We can verify our states by using the React Developer Tools. To verify, just start the app by "npm start", right click on the page and goto Inspect, from inspect you can goto components section.
Getting a input for date from the user
Now, we need a form in "Date" to take the input from the user and update it in the state of App. This implies we need a function in App to update the state and pass it to "Date" component. The function will be considered when the date is entered and submitted. Since we haven't made the form yet, therefore to prevent the default behaviour of the event, make the changes to the previous code ->
class App extends Component {
...
updateDate = e => {
e.preventDefault();
console.log(e.target);
};
render() {
return (
<div>
<h1>NASA's Astronomy Picture of the Day</h1>
<Date updateDate={this.updateDate} />
<FetchedPhoto />
</div>
);
}
}
Now, we need a form to take the input from the user. When the user submits the form the "updateDate" function is called. So, in Date.js file make the following changes ->
const Date = props => (
<form onSubmit={props.updateDate}>
Enter a date (YYYY-MM-DD):
<input />
<input type="submit" />
</form>
);
We see the following form on the screen ->
Now, for the value of the first field of the form(that is Date), in our "updateDate" function, update the code in "App.js" as ->
class App extends Component {
...
updateDate = e => {
e.preventDefault();
let dateFromInput = e.target[0].value;
this.setState({ date: dateFromInput });
};
...
}
Fetching Photo from the API according to the date entered by the user
To use the NASA APOD API just signup to this Your Unique API Key and it will generate your API Key. It looks like https://api.nasa.gov/planetary/apod?date=2009-12-19&api_key=YOUR_KEY. Here, YOUR_KEY is your own unique and personal key.
Now, We have to first pass the photo down to the "FetchedPhoto" component, which will render it ->
class App extends Component {
...
render() {
return (
<div>
<h1>NASA's Astronomy Picture of the Day</h1>
<Date updateDate={this.updateDate} />
<FetchedPhoto photo={this.state.photo} />
</div>
);
}
}
Now, we need to update out "FetchedPhoto" component to display the photo from the date provided by the user. It will show the Photo's title, the photo and the explaination of the photo.Therefore, in the "FetchedPhoto.js" file, update the following ->
const PhotoFromapi = props => (
<div>
<h3>{props.photo.title}</h3>
<img src={props.photo.url} alt={props.photo.title} />
<p>{props.photo.explanation}</p>
</div>
);
Displaying the picture from the API
For this, we need to write a function that will take the date from the user, fetch the photo with the date given and set the photo. Therefore, write the following function to "App.js" file->
class App extends Component {
...
FetchPhotoFromApi = date => {
fetch(`https://api.nasa.gov/planetary/apod?date=${date}&api_key=YOUR_UNIQUE_KEY`)
.then(response => response.json())
.then(photoData => this.setState({ photo: photoData }));
};
...
}
After a user inputs a date and submit the "updateDate" function is called. To call "FetchPhotoFromApi" function at the same time just type the following in App.js ->
class App extends Component {
...
updateDate = e => {
e.preventDefault();
let dateFromInput = e.target[0].value;
this.setState({ date: dateFromInput });
this.FetchPhotoFromApi(dateFromInput);
};
}
Now, our application is completed. The complete source code is as follows:
#App.js
import React, { Component } from "react";
import Date from "./components/Date";
import FetchedPhoto from "./components/FetchedPhoto.js";
class App extends Component {
state = {
date: "",
PhotoFromapi: ""
};
updateDate = e => {
e.preventDefault();
let dateFromInput = e.target[0].value;
this.setState({ date: dateFromInput });
this.FetchPhotoFromApi(dateFromInput);
};
FetchPhotoFromApi = date => {
fetch(`https://api.nasa.gov/planetary/apod?date=${date}&api_key=YOUR_UNIQUE_KEY`)
.then(response => response.json())
.then(photoData => this.setState({ photo: photoData }));
};
render() {
return (
<div>
<h1>NASA's Astronomy Picture of the Day</h1>
<Date updateDate={this.updateDate} />
<FetchedPhoto photo={this.state.photo} />
</div>
);
}
}
export default App;
#Date.js
import React from "react";
const Date = props => (
<form onSubmit={props.updateDate}>
Enter a date (YYYY-MM-DD) :
<input />
<input type="submit" />
</form>
);
export default Date;
#FetchedPhoto.js
import React from "react";
const Photo = props => (
<div>
<h3>{props.photo.title}</h3>
<img src={props.photo.url} alt={props.photo.title} />
<p>{props.photo.explanation}</p>
</div>
);
export default FetchedPhoto;
Output
Lets take a date -> 2019-12-28
You can checkout in React Developer Tools, In the Components section, the state of our app after updating the date. Here is the states:
date
"2019-12-28"
PhotoFromapi
{copyright: "Elias Chasiotis", date: "2019-12-28", …}
copyright
"Elias Chasiotis"
date
"2019-12-28"
explanation
"Yes, but have you ever seen a sunrise like this? Here, after initial cloudiness, the Sun appeared to rise in two pieces and during partial eclipse, causing the photographer to describe it as the most stunning sunrise of his life. The dark circle near the top of the atmospherically-reddened Sun is the Moon -- but so is the dark peak just below it. This is because along the way, the Earth's atmosphere had an inversion layer of unusually warm air which acted like a gigantic lens and created a se..."
hdurl
"https://apod.nasa.gov/apod/image/1912/DistortedSunrise_Chasiotis_2442.jpg"
media_type
"image"
service_version
"v1"
title
"A Distorted Sunrise Eclipse"
url
"https://apod.nasa.gov/apod/image/1912/DistortedSunrise_Chasiotis_1080.jpg"
Note that the key information you can get from the NASA API are:
- Image URL which you can download
- Title of the image
- Explanation of the image
- Date on which the image has been featured
- Photographer or copyright owner of the image
Links to learn more:
- NASA APOD API by NASA
- Source code of this application at OpenGenus GitHub
With this, you have the complete knowledge of getting the Astronomy picture of the day using NASA API. Enjoy.