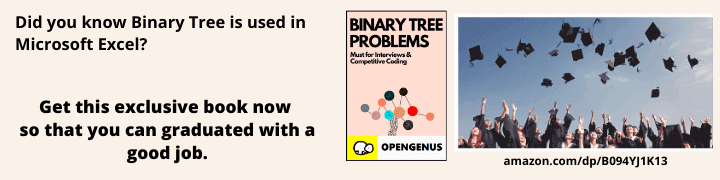
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the concept of Simple Interest with the twists in it and implemented a program in C Programming Language to calculate Simple Interest.
Introduction to Simple Interest
Simple Interest is a concept that calculates the interest provided on the sum of money. This is a basic finance concept and comes in case of Fixed Deposits in bank.
The steps are as follows:
- A person deposits amount X in a bank account as fixed deposit
- The rate of interest offered by the bank is Y (% per year) at that time. It is important to note that this rate of interest will remain fixed for this person and any duration.
- The person keeps the money in the fixed deposit for M years.
- The interest earned every year is same and is accumulated over the M years.
Interest on first year I = Y * X
Total interest in M years = M * I = M * Y * X
The catch is there is a cap of the maximum duration. It is usually 10 years. Additionally, the interest is given out every 3 months. It is the 1/4 th of the total annual interest.
C Program to calculate Simple Interest
We have one main function: calSimpleInterest(). It simply calculates the total interest.
Note that the principle and time variable are integers while rate is in float.
Following is the C Program to calculate Simple Interest:
#include <stdio.h>
float calSimpleInterest(int principle, float rate, int time) {
float simple_interest;
simple_interest = (principle * rate * time) / 100;
return simple_interest;
}
int main() {
int principle, time;
float rate;
float simple_interest;
printf("Enter principle amount in USD: ");
scanf("%d", &principle);
printf("\nEnter rate of interest: ");
scanf("%f", &rate);
printf("\nEnter time in years: ");
scanf("%d", &time);
// Calculate simple interest
simple_interest = calSimpleInterest(principle, rate, time);
printf("\nThe interest amount is: %f USD\n", simple_interest);
return 0;
}
Compile and run the above code as follows:
gcc opengenus.c
./a.out
Input + Output:
Enter principle amount in USD: 1000
Enter rate of interest: 2.4
Enter time in years: 5
The interest amount is: 120.000000 USD
C Program to calculate Simple Interest with all real-life catches
The real catches/ changes will be:
- All principle, rate and time will be in float.
- Time can be in multiples of 0.25 only (as interest is deposited every 3 months)
- Total time should be less than 10.
We check the total time and return immediately if it is greater than the limit.
if(time > 10.0) {
printf("Time should be less than 10 years");
return 0;
}
We handle the time to be in multiples of 0.25 only. The idea is to get the integer part and check the remaining decimal part.
- If the decimal part is less than 0.25, it contributes 0.
- If the decimal part is less than 0.50, it contributes 0.25.
- If the decimal part is less than 0.75, it contributes 0.5.
- else the contribution is 0.75.
Following function handles this:
float roundRate(float rate) {
int baseRate = floor(rate);
float remainingRate = rate - baseRate;
float finalRate = 0;
if(remainingRate < 0.25)
finalRate = baseRate;
else if(remainingRate < 0.5)
finalRate = baseRate + 0.25;
else if(remainingRate < 0.75)
finalRate = baseRate + 0.5;
else
finalRate = baseRate + 0.75;
return finalRate;
}
Following is the C Program to calculate Simple Interest:
#include <stdio.h>
#include <math.h>
float calSimpleInterest(int principle, float rate, int time) {
float simple_interest;
simple_interest = (principle * rate * time) / 100;
return simple_interest;
}
float roundRate(float rate) {
int baseRate = floor(rate);
float remainingRate = rate - baseRate;
float finalRate = 0;
if(remainingRate < 0.25)
finalRate = baseRate;
else if(remainingRate < 0.5)
finalRate = baseRate + 0.25;
else if(remainingRate < 0.75)
finalRate = baseRate + 0.5;
else
finalRate = baseRate + 0.75;
return finalRate;
}
int main() {
int principle, time;
float rate;
float simple_interest;
printf("Enter principle amount in USD: ");
scanf("%d", &principle);
printf("\nEnter rate of interest: ");
scanf("%f", &rate);
printf("\nEnter time in years: ");
scanf("%d", &time);
if(time > 10.0) {
printf("Time should be less than 10 years");
return 0;
}
rate = roundRate(rate);
// Calculate simple interest
simple_interest = calSimpleInterest(principle, rate, time);
printf("\nThe interest amount is: %f USD\n", simple_interest);
return 0;
}
Input + Output:
Enter principle amount in USD: 1200
Enter rate of interest: 2.57
Enter time in years: 5.49
The interest amount is: 150.000000 USD
With this article at OpenGenus, you must have the complete idea of how to implement a C program to calculate Simple Interest.