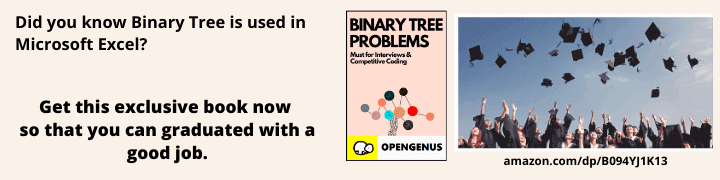
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Control flow statements are used for the controlled logical and conditional execution of a statement. All control flow statements are associated with boolean value - it can be either true or false, and depending on that, operates in a given pattern.
Note:
Because of the flexibility of the Ruby language, most of these statements can be used as statement modifiers - which was borrowed from Perl.
There may be different ways to write these statements. It would not be possible to cover all of them, but they can be accessed from other sources. The Ruby documentation would be a great place to start.
Warning!!:
Sometimes, loops don't stop, because their condition remains true. Such loops are called infinite loops and can hog all the PC resources, till it crashes. To close a process on the terminal, the universally common way to kill such process is Ctrl + C (although it can vary, depending on what terminal you're using), and follow the instructions. There can be times, where you may have to press the shortcut twice.
Decision-making Statements
if-else and elsif
An if statement is a very simple control flow statement, where conditions are checked to see if it matches or not. If it does, then the block inside will be executed.
if condition
# execute this statement
end
Here is a demo program of an if statement-
# frozen_string_literal: true
a = 7
puts 'a is 7' if a == 7
---OUPUT---
Value of a is 7
if can be paired with else, so that an alternative block can execute if the condition is false.
# frozen_string_literal: true
a = 5;
if a == 7
puts 'a is 7'
else
puts 'Not equal to 7'
end
---OUTPUT---
Not equal to 7
Note:
else is not just limited to if statement. It can also be used with case or unless
Sometimes there can also be cases where we can have a else statement to follow a given condition. We can use elsif in such a situation.
# frozen_string_literal: true
a = 3;
if a == 7
puts 'a is 7'
elsif a == 3
puts 'a is 3'
else
puts 'Not equal to 7'
end
---OUTPUT---
a is 3
There is also a ternary form of if-else statement. The syntax is-
value = condition ? 'this, if true' : 'this, if false'
This is how an program can be written as, for the ternary form-
# frozen_string_literal: true
a = 3
s = a == 7 ? 'Value is 7' : 'Not 7'
print s
---OUTPUT---
Not 7
Note:
Here, s is a string-type object, whereas a is an integer-type object.
Integers store numbers, whereas strings store sequence of characters. s is storing the string 'Not 7' because the following code "a == 7 ? 'Value is 7' : 'Not 7'" is executed first, and based on the condition, sets any one of the given string as the value of s.
unless
unless is a control statement that serves the opposite of a if statement. It is written as a modifier statement.
statement unless condition
An example code is-
# frozen_string_literal: true
x = 3
puts('x is not equal to 2') unless x == 2
---OUTPUT---
x is equal to 2
Note:
unless can also be used in place of if, but for best practices, it should be used with a single line statement, as a modifier.
# an example of normal unless statement
unless condition
# execute this statement
else
# execute the alternative statement
end
case
A case statement used to remove ambiguous if-else statements. It is similar to the switch statement in C/C++.
case expression(can be condition too)
when expression 1
# Execute for expression 1
when expression 2
# Execute for expression 2
when expression 3
# Execute for expression 3
else
# Execute the default, if all fails.
end
An example program would be-
# frozen_string_literal: true
number = 3
case number
when 1
puts 'The number is 1'
when 2
puts 'The number is 2'
when 3
puts 'The number is 3'
when 4
puts 'The number is 4'
else
'None of the provided conditions matches'
end
---OUTPUT---
The number is 3
Loop statements
while
while statement is used to create a loop, based on the fact that the given condition remains true.Once the condition fails, the loop is broken. while loop is an entry-controlled loop.
The syntax is-
while condition [do]
#statement to loop
end
An example would look something like-
# frozen_string_literal: true
x = 4
while x.positive?
puts x
x -= 1
end
---OUTPUT---
4
3
2
1
Note:
while can also be written as a statement modifier.
# frozen_string_literal: true
y = 1
puts y while y < 10
# statement modifier form
---OUTPUT---
1
1
1
1
....
do-while
do-while is another such loop similar to while, except that it is an exit-controlled loop.
loop do
#code to be executed
break if booleanexpression
end
An example for do-while would look like-
# frozen_string_literal: true
solution = 0
loop do
puts 'Type any number[press q to quit]'
answer = gets.chomp
solution += answer.to_i if answer.to_i.is_a? Numeric
break if answer == 'q'
end
puts "Solution is: #{solution}"
---OUTPUT---
Type any number[press q to quit]
1
Type any number[press q to quit]
4
Type any number[press q to quit]
6
Type any number[press q to quit]
8
Type any number[press q to quit]
q
Solution is: 19
for
for loop is another such loop similar to while, but the only exception being that it can self-increment.
for variable [variable ...] in expression [do]
# write statement
end
An example code would be-
# frozen_string_literal: true
x = [1, 2, 3, 4]
for x in 0..4
puts "Value of x is : #{x}"
end
---OUTPUT---
Value of x is : 0
Value of x is : 1
Value of x is : 2
Value of x is : 3
Value of x is : 4
until
until is a control statement that serves the opposite of a while statement - it loops till the condition is true.
until conditional [do]
# code to be executed
end
This is how an example code would look like-
# frozen_string_literal: true
x = 4
until x.zero?
puts x
x -= 1
end
---OUTPUT---
4
3
2
1
break
break statement is used to terminate the loop
break
A simple program can be written as-
# frozen_string_literal: true
(0..5).each do |x|
break if x > 3
puts x
end
---OUTPUT---
0
1
2
3
4
next
next is another such statement that works similar to break, except that the flow is not broken, but instead skipped.
next
next can be shown with this simple example-
# frozen_string_literal: true
(0..5).each do |x|
next if x == 4
puts x
end
---OUTPUT---
0
1
2
3
5
redo
If redo is used inside a loop, it will start the loop without evaluating the condition. This can even cause an infinite loop.
redo if condition
redo can be added to a loop, as shown by the example-
# frozen_string_literal: true
5.times do |i|
puts "Iteration #{i}"
redo if i > 3
end
---OUTPUT---
Iteration 0
Iteration 1
Iteration 2
Iteration 3
Iteration 4
Iteration 4
Iteration 4
Iteration 4
Iteration 4
....
retry
There are instances when our code fails to get the required data, because of a timeout, or delay in recieving the information. retry is what we will be using, to reiterate a block, or a loop, in case of an exception.
retry
A simple code using retry looks like-
# frozen_string_literal:true
5.times do |i|
begin
puts "Currently at #{i}"
raise if i >= 4
rescue StandardError
retry
end
end
---OUTPUT---
Currently at 0
Currently at 1
Currently at 2
Currently at 3
Currently at 4
Currently at 4
Currently at 4
...
Iterator statements
times
times is another unique type of loop in Ruby, that is used mostly as a statement modifier.
number.times do { #statement }
A simple example would be
# frozen_string_literal: true
3.times { p 'Hello there' }
---OUTPUT---
Hello there
Hello there
Hello there
This statement can be written in a different form too-
# frozen_string_literal: true
3.times do |i|
puts "Printing #{i}"
end
---OUTPUT---
Printing 0
Printing 1
Printing 2
each
each loop in ruby is one of the most used statement, which simplifies the looping of values inside lists, dictionaries and arrays.
It's syntax is-
variable.each do |n|
# statement to execute
end
One simple example would be-
# frozen_string_literal: true
colors = %w[Red Blue Yellow]
colors.each do |n|
puts "This color is #{n}"
end
---OUTPUT---
This color is Red
This color is Blue
This color is Yellow
upto
upto is another such statement modifier that acts as an unconventional loop
It's syntax is-
a_number.upto(another_number) do something
An example program would be-
# frozen_string_literal: true
limit = 5
1.upto(limit) do
puts 'Hello world'
end
---OUTPUT---
Hello world
Hello world
Hello world
Hello world
Hello world
downto
downto works in the same way as step, except that it decrements the value.
a_number.downto(another_number) do something
An example would be-
# frozen_string_literal: true
limit = 0
5.downto(limit) do |x|
puts "Printing #{x}"
end
---OUTPUT---
Printing 5
Printing 4
Printing 3
Printing 2
Printing 1
Printing 0
step
step is another such iterator similar to upto and downto, but with an exception that it can go both ways, and rather than incrementing by one, we can choose by how many steps we want to increment.
number.step(limit, step-count) do something
This is how we can use a step iterator as-
# frozen_string_literal: true
0.step(12, 4) do |v|
puts v
end
---OUTPUT---
0
4
8
12
Question 1
Ruby was inspired by which of the following languages for statement modifiers?
Question 2
A ternary statement is a short-form of which of the given control-flow statements?
Question 3
Given below is a simple step statement
number.step(limit, step-count) do |something|
puts something
end
number.step(limit, step-count) do |something|
puts something
end
if number is 3, and limit is 10, what should the step-count be to print the following sequence?
3
5
7
9
With this, you must have a strong idea of Control Flow statements in Ruby.