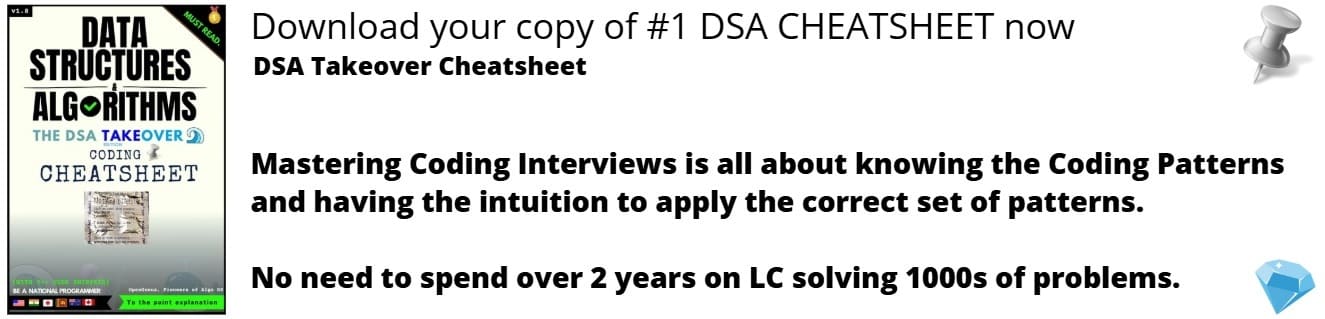
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained how to convert / compile a C or C++ code to Assembly code using GCC or G++ compiler.
Table of contents:
- Convert C code
- Convert C++ code
Convert C code
The command to convert a given C code to Assembly code is as follows:
gcc -S opengenus.c
This command will save the assembly code in a filename opengenus.s. The filename syntax is "<filename>.s
".
For example, if this is your C code:
int main() {
int a = 5, b = -1;
int sum = a + b;
return 0;
}
The corresponding Assembly Code will be as follows:
.file "opengenus.c"
.text
.def ___main; .scl 2; .type 32; .endef
.globl _main
.def _main; .scl 2; .type 32; .endef
_main:
LFB0:
.cfi_startproc
pushl %ebp
.cfi_def_cfa_offset 8
.cfi_offset 5, -8
movl %esp, %ebp
.cfi_def_cfa_register 5
andl $-16, %esp
subl $16, %esp
call ___main
movl $5, 12(%esp)
movl $-1, 8(%esp)
movl 12(%esp), %edx
movl 8(%esp), %eax
addl %edx, %eax
movl %eax, 4(%esp)
movl $0, %eax
leave
.cfi_restore 5
.cfi_def_cfa 4, 4
ret
.cfi_endproc
LFE0:
.ident "GCC: (GNU) 7.4.0"
Convert C++ code
The command to convert a given C++ code to Assembly code is as follows:
g++ -S opengenus.cpp
Note, we have to use g++ compiler for C++ code. It is not a good practice to use gcc compiler for C code.
This will save the Assembly code.
For example, if this is your C++ code:
int main() {
int a = 12, b = 4;
int multiply = a * b;
return 0;
}
The corresponding Assembly Code will be as follows:
.file "opengenus.cpp"
.text
.def ___main; .scl 2; .type 32; .endef
.globl _main
.def _main; .scl 2; .type 32; .endef
_main:
LFB0:
.cfi_startproc
pushl %ebp
.cfi_def_cfa_offset 8
.cfi_offset 5, -8
movl %esp, %ebp
.cfi_def_cfa_register 5
andl $-16, %esp
subl $16, %esp
call ___main
movl $12, 12(%esp)
movl $4, 8(%esp)
movl 12(%esp), %eax
imull 8(%esp), %eax
movl %eax, 4(%esp)
movl $0, %eax
leave
.cfi_restore 5
.cfi_def_cfa 4, 4
ret
.cfi_endproc
LFE0:
.ident "GCC: (GNU) 7.4.0"
With this article at OpenGenus, you must have the complete idea of generating assembly code from C and C++ code.