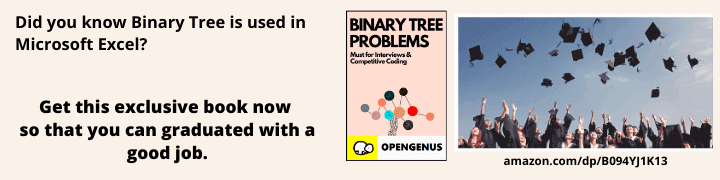
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The C programming language, with its versatility and powerful memory manipulation capabilities, is well-suited for system programming tasks such as file management, including file deletion. The topic "Different ways to delete file in C" explores the efficient and flexible approaches to deleting files using the C programming language. Since C is widely used for developing portable applications, the different file deletion methods can be applied to various operating systems and platforms.
This article explores various methods for file deletion in C programming language.
Table of contents
- Introduction
- Basic File Deletion in C
- Using System Calls for File Deletion
- Deleting Files Permanently
- Dealing with Errors
- Performance and Complexity
- Applications
Introduction
In modern computing environments, files are ubiquitous and play a vital role in a wide range of applications and systems. From text files and documents to media files and databases, files are essential building blocks that enable the creation and sharing of digital content.
However, the ability to effectively manage files also includes the ability to delete them. In this article, I will explore various methods for deleting files in the C programming language. my aim is to provide readers with a comprehensive understanding of the different approaches to file deletion, their strengths and weaknesses, and the practical implications for developers and system administrators.
I will begin by discussing the importance of file deletion in modern computing environments, and the need for effective and reliable methods to accomplish this task. From there, I will provide an overview of the different methods for file deletion that will be covered in this article. This will include a comparison between standard library functions and system calls, an exploration of permanently deleting files, and an examination of the performance and complexity of different file deletion methods.
By the end of this article, readers will have a solid understanding of the various methods for deleting files in C, and the considerations that must be taken into account when choosing the appropriate approach. Whether you are a developer working with file-based applications, or a system administrator responsible for managing file systems, this article will provide valuable insights and practical guidance.
Basic File Deletion in C
When it comes to file deletion in C, one of the most common approaches is to use the standard library functions that are provided by the language. These functions provide a convenient and straightforward way to delete files, and are suitable for many applications and use cases.
The standard library functions for file deletion in C are included in the <stdio.h>
header file, and are as follows:
remove(const char* filename)
: This function takes a filename as an argument, and deletes the file if it exists. If the file does not exist, the function returns a non-zero value to indicate an error.
unlink(const char* filename)
: This function is similar to remove()
, but is a bit more low-level. It is used to delete a file by unlinking it from the file system, rather than using the traditional delete operation. Like remove()
, this function returns a non-zero value if the file does not exist.
Here's an example code snippet that demonstrates the use of remove()
:
#include <stdio.h>
int main() {
char* filename = "myfile.txt";
int status = remove(filename);
if (status == 0) {
printf("File '%s' deleted successfully.\n", filename);
} else {
printf("Error deleting file '%s'.\n", filename);
}
return 0;
}
In this example, we have a file called myfile.txt that we want to delete. We pass the filename to the remove() function
, and check the return value to see if the operation was successful. If it was, we print a success message, and if not, we print an error message.
Similar to remove()
, here's an example code snippet that demonstrates the use of unlink()
:
#include <stdio.h>
int main() {
char* filename = "myfile.txt";
int status = unlink(filename);
if (status == 0) {
printf("File '%s' deleted successfully.\n", filename);
} else {
printf("Error deleting file '%s'.\n", filename);
}
return 0;
}
As you can see, the code for using unlink()
is nearly identical to that of remove()
. The main difference is that unlink()
is a bit more low-level, and does not perform the traditional delete operation that most users are familiar with. However, for many applications, this is not a problem, and using unlink()
can provide a simple and efficient way to delete files.
Using System Calls for File Deletion:
In addition to using standard library functions, developers can also use system calls to delete files in C. This section will provide an overview of how to use system calls for file deletion, including examples and code snippets.
System calls are lower-level functions that provide direct access to the operating system's kernel. Using system calls can offer greater control and flexibility over file deletion than standard library functions. One commonly used system call for file deletion in C is the unlink()
function.
The unlink()
function takes a single argument, which is the name of the file to be deleted. Here is an example of how to use unlink()
to delete a file:
#include <unistd.h>
int main() {
if (unlink("filename.txt") == -1) {
perror("Error deleting file");
} else {
printf("File deleted successfully");
}
return 0;
}
In this example, the unlink()
function is used to delete a file named filename.txt. The function returns 0 if the file is deleted successfully, and -1 if an error occurs. The perror()
function is used to print an error message if the deletion fails.
Using system calls for file deletion in C can offer greater control and flexibility than using standard library functions. However, it is important to use them with caution, as they can have more significant consequences and require a deeper understanding of the underlying system.
Deleting Files Permanently
When files are deleted using standard library functions or system calls, they are not always permanently removed from the system. In some cases, it may be possible to recover deleted files using data recovery software. This can pose a security risk, particularly when dealing with sensitive information.
To ensure that files are deleted permanently and cannot be recovered, there are several methods that can be used. One method is to overwrite the contents of the file with random data before deleting it. Another method is to use specialized tools that are designed to securely delete files.
In C, one way to overwrite the contents of a file before deleting it is to use the fopen()
and fwrite()
functions to open the file, write random data to it, and then close the file. Here is an example:
#include <stdio.h>
int main() {
FILE *fp = fopen("filename.txt", "wb");
char data[1024] = "random data";
fwrite(data, 1, sizeof(data), fp);
fclose(fp);
remove("filename.txt");
return 0;
}
In this example, the fopen()
function is used to open the file filename.txt in binary mode. The fwrite()
function is then used to write random data to the file, and the fclose()
function is used to close the file. Finally, the remove()
function is used to delete the file.
It is important to note that overwriting the contents of a file before deleting it may not always guarantee that the file is permanently erased, particularly on systems with advanced data recovery tools. Using specialized tools that are designed to securely delete files, such as the shred command on Linux systems, may be a more reliable option for ensuring that files are permanently deleted and cannot be recovered.
Dealing with Errors when deleting files
When deleting files in C, it is important to be aware of common errors that can occur during the process. These errors can range from simple mistakes in the code to more complex issues that may require troubleshooting and debugging.
One common error that can occur is attempting to delete a file that does not exist. This can happen if the file has already been deleted or if the file path is incorrect. In this case, the program should check for the existence of the file before attempting to delete it, and handle the error if the file does not exist.
Another error that can occur is when the file is in use by another program or process. In this case, the program may be unable to delete the file until the other program has released its hold on the file. To handle this error, the program can use system calls or library functions to check if the file is in use before attempting to delete it.
Other errors that can occur during file deletion include permission errors, disk full errors, and errors caused by issues with the file system. To handle these errors, the program should check for appropriate permissions before attempting to delete the file, ensure that there is sufficient disk space available, and use error handling techniques such as try-catch blocks or error codes to handle any issues that arise.
By being aware of common errors that can occur during file deletion and taking steps to handle them, developers can ensure that their code is reliable and able to handle a wide range of scenarios.
Performance and Complexity
The performance and complexity of different file deletion methods in C is an important consideration for developers and system administrators. In this section, i will discuss the different factors that can affect the performance and complexity of file deletion, and provide an analysis of some of the most common file deletion methods.
One factor that can affect the performance of file deletion is the size of the file being deleted. In general, deleting larger files will take longer than deleting smaller files. This is because larger files require more disk I/O operations, which can be a time-consuming process.
Another factor that can affect the performance of file deletion is the location of the file being deleted. For example, deleting a file from a local hard drive may be faster than deleting a file from a remote network drive, as network operations tend to be slower.
The complexity of file deletion methods in C can also vary depending on the method being used. For example, using the standard library functions for file deletion can be a relatively simple and straightforward process, while using system calls may require more detailed knowledge of the operating system and its file system.
Overall, the performance and complexity of file deletion methods in C is an important consideration for developers and system administrators.
Applications
In this section, i will explore some of the real-life applications and systems that make use of file deletion in C programming.
One common application of file deletion is in operating system file management. Operating systems frequently need to delete temporary files, log files, and other system files that are no longer needed. These files can accumulate over time and take up valuable disk space, so it is important to delete them in a timely and efficient manner. C programming provides a range of functions and system calls that allow developers to manage these files and ensure that they are deleted as needed.
Another important application of file deletion in C is in software development. Developers frequently need to delete old versions of source code files, build files, and other temporary files that are no longer needed. These files can take up significant disk space and cause issues with version control, so it is important to delete them in a timely and efficient manner. C programming provides a range of tools and techniques for managing and deleting these files, including standard library functions, system calls, and custom scripts.
File deletion is also an important part of data security and privacy. When files are deleted, they can often be recovered using specialized software tools, which can pose a risk to sensitive or confidential data. C programming provides a range of techniques for ensuring that files are deleted permanently and cannot be recovered, including overwriting the file with random data, renaming the file multiple times, and deleting the file using specialized system calls.
In summary, file deletion is a crucial aspect of modern computing, and C programming provides a range of tools and techniques for managing and deleting files in a reliable and efficient manner. By understanding the applications and use cases for file deletion in real-life systems and applications, developers and system administrators can ensure that their systems are secure, performant, and well-managed.
Sample questions for an article on Different ways to delete file in C
- What are the different methods for file deletion in C?
- What is the difference between using standard library functions and system calls for file deletion?
- How can you ensure that files are deleted permanently and cannot be recovered
- What are some common errors that can occur when deleting files in C, and how can they be handled?
- How does the performance and complexity of different file deletion methods vary in C?
- Can you use a combination of methods for file deletion in C?
- How can you check if a file has been successfully deleted in C?
- What are the security implications of file deletion in C, and how can they be addressed?
- How can you handle errors related to file permissions when deleting files in C?
- Give an example of a real-life application or system where file deletion is important.