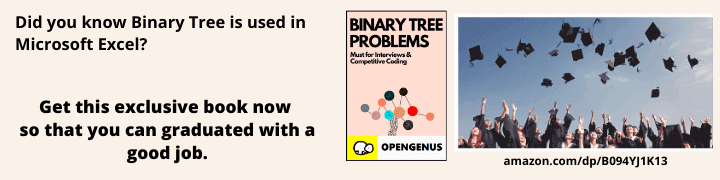
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we are going to discuss the importance of documentation, how learning to document your systems is going to help you become a better programmer and systems architect and why should you use swagger when developing an API.
Most programmers have been in a situation where you open your code files that were written months ago, and you start reading, trying to understand what each of those functions and logic are supposed to do, after trying a lot you just give up and choose not to understand what this code is doing. Documenting your code can help you with those situations.
Documentation, why is important?
First we have to understand that a bad code with an awesome documentation is not going to help a lot, when writing your code you have to think not only about if it is going to run or not, you should think about if it is readable enough for other people to read and maybe help with the development of your software. But some codes even with readable development can be confusing, maybe you are trying to tell what type of answer the API should get when developing it, but commenting this in every requisition or endpoint wouldn’t be ideal.
So you should document the choices you make when developing a software, writing documentation make the system more understandable, making a better user experience, that way you are saving time and resource because the user won’t seek support for help.
The maintenance and updates of the system will be faster, easier, and updates or fixing of bugs will be much more accurate because of this system “map”. Making it very accessible for new developers and juniors that have a hard time understanding systems that they are going to start developing, this way the onboarding and training of new developers is faster, and it can make devs more specialize on the business side or other important aspects of the system.
Overall, documenting your systems won’t have any downside, even if you think writing documentation is a waste of time, and you should focus more on developing, when going back to your old repository you are going to thank yourself.
Why it will make you a better developer and system architect
For you to be a great developer or architect you should understand deeply what you are talking about, when writing documentation you can think through your code and architecture and organize your thoughts in a way you can understand all your choices. For a software architect, it is essential good communication for when you are explaining complex technical concepts for a group of developers or even for non-technical people. Documentation can also reinforce the importance of good coding practices, and it makes you think about edge cases more clearly, helping you identify uncommon usages of the system you are developing, that way making you a better developer or system architect
Using swagger as a documenting tool.
So if you want to start documenting your API’s, I would recommend you using swagger. Swagger is an excellent tool, it gives you several utilities like giving you an endpoint for documentation on your API, it gives a consistency ensure to follow the industry best practices. You can generate code for your API with a variety of languages, you can also test your API with swagger UI, without having to write a client code just to make sure everything is alright.
import express from 'express';
import swaggerJsdoc from 'swagger-jsdoc';
import swaggerUi from 'swagger-ui-express';
const app = express();
const options = {
definition: {
openapi: '3.0.0',
info: {
title: 'My API',
version: '1.0.0',
description: 'A sample API'
},
servers: [{
url: '<http://localhost:3000>'
}]
},
apis: ['./routes/*.ts']
};
const swaggerSpec = swaggerJsdoc(options);
app.use('/docs', swaggerUi.serve, swaggerUi.setup(swaggerSpec));
app.get('/hello', (req, res) => {
/**
* @swagger
* /hello:
* get:
* description: Returns a hello message
* responses:
* 200:
* description: hello message
*/
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In this previous example, you can see how swagger works in a really simple way, it gives you a new endpoint (‘/docs’) for testing and visually analyzing the responses of each request.
Of course as it is a simple API example the documentation will be simple as well, and with time and more developing the documentation along with the server code can be a little confusing, but nothing a controller and router can’t resolve.