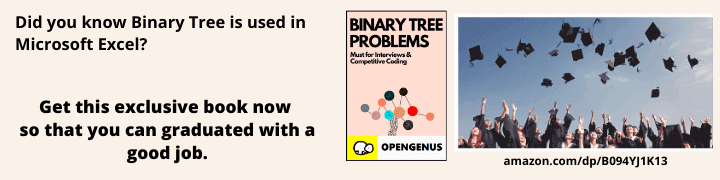
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn the concept of empty, undefined and null in JavaScript and how to use JavaScript to check the Empty, Null and Undefined string on the client/user side for authentication purpose.
This is functional to have accurate and consistent data before forwarding it to the server. We can assume that the main purpose of this function is to validate the values input by the users either empty or filled.
In formal language theory, the empty string, or empty word, is the unique string of length zero. A string is null if has not been assigned a value and lastly the undefined term means a variable that does not have a value. In this article we will be using JavaScript
functions to check Empty/Null/Undefined strings.
- undefined: A variable is said to be
undefined
if it has been declared but has not been assigned a value. For example:
let x;
console.log(x); // undefined
- null: A variable is said to be
null
if it has been explicitly assigned thenull
value. It is often used to represent the intentional absence of any object value. For example:
let y = null;
console.log(y); // null
- Empty value: An empty value is represented by an empty string ("" or ''). It is a string with no characters in it. For example:
let z = "";
console.log(z); // ""
Differences between Empty/Null/Undefined :
Empty | Null | Undefined |
---|---|---|
An "empty" value is a value that is an empty string. | "Null" is a value that represents the intentional absence of any object value. | A variable or value is "undefined" when it has been declared, but no value has been assigned to it. |
Empty values are not the same as undefined or null. | Null is different from undefined because it is an explicit value that can be assigned to a variable | It is also undefined when it is called as a function argument and no value is passed to it |
The methods are listed below:
1: Using === operator
2: By using the length and ! operator
3: By using replace
The basic concept of the methods:
-
The === operator: This operator compares two values for equality without type coercion. It returns true if the values are of the same type and have the same value, and false otherwise. To check for undefined or null values, you can use the === operator with undefined or null, respectively.
-
The ! (not) operator and length property: You can use the ! operator to check if a value is falsy (i.e., evaluates to false in a boolean context), which includes undefined, null, and an empty value ("" or ''). To check for an empty value specifically, you can also check its length property, which will be 0 for an empty string.
-
The replace() method: You can use the replace() method with a regular expression to check for an empty value ("" or ''). This method replaces a specified value with another value in a string. If the specified value is not found in the string, it returns the original string.
1: Using === operator
Code:
// Using === operator to check for undefined and null
let x;
console.log(x === undefined); // true
let y = null;
console.log(y === null); // true
Output:
True
False
2: By using the length and ! operator
Code:
// function to check string is empty or not
function checking(str) {
// checking the string using ! operator and length
// will return true if empty string and false if string is not empty
return (!str || str.length === 0 );
}
// calling the checking function with empty string
console.log(checking(""))
// calling the checking function with not an empty string
console.log(checking("OpenGenus"))
Output:
Empty String
Not Empty String
3: By using replace
Code:
// function to check string is empty or not
function checking(str){
if(str.replace(/\s/g,"") == ""){
console.log("Empty String")
}
else{
console.log("Not Empty String")
}
}
// calling the checking function with empty string
checking(" ")
// calling the checking function with not an empty string
checking("Hello Javascript")
Output:
Empty String
Not Empty String
Differences in the Methods:
=== operator | Length and ! operator | Replace method |
---|---|---|
Useful for checking if a value is specifically `undefined` or `null`. | Check if a value is false or an empty string. | Useful for checking if a string consists only of whitespace characters. |
Returns true or false output. | Returns Empty or Not Empty output. | Replaces a specified value with another value in a string. |
In conclusion of this article at OpenGenus, it's worth noting that null
is of type object, whereas undefined
is of type undefined
. Also, null
is considered false whiche mean it evaluates to false in a boolean context, while undefined
is not strictly false but evaluates to false in a boolean context. An empty value ("" or '') is also false.