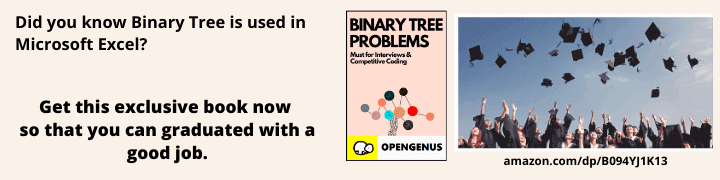
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
Climate change, one of the most daunting challenges faced by humanity, is primarily marked by fluctuations in global temperatures. These alterations have far-reaching consequences on ecosystems and human societies. Predicting these changes with accuracy is crucial for devising preparedness and mitigation strategies. Machine Learning (ML) and Deep Learning (DL), subfields of artificial intelligence, have demonstrated great promise in predictive modeling and data analysis. This article at OpenGenus explores how ML and DL can be effectively utilized for global temperature change prediction.
Understanding the Data
Sources of Climate Data
For accurate predictions, we require robust datasets. The main sources include:
Historical Weather Records: These are archived records containing data points such as temperature, humidity, and precipitation levels. Libraries like NOAA’s National Centers for Environmental Information are treasure troves for historical weather records.
Satellite Data: Remote sensing data obtained from satellites that orbit the Earth captures large-scale atmospheric conditions. This data includes cloud formations, sea surface temperatures, and atmospheric compositions.
Oceanic Data: This consists of information on ocean currents, sea surface temperatures, and salinity levels, which play a significant role in regulating global temperatures.
Preprocessing Climate Data
Raw data needs to be processed before being fed into models:
Data Cleaning: This involves removing inconsistencies, errors, and irrelevant information from the dataset.
Data Integration: As climate data comes from various sources, it is essential to integrate them into a consistent format.
Handling Missing Data: Climate datasets may have missing values, which need to be handled using imputation methods to ensure the model's effectiveness.
Introduction to Machine Learning (ML) and Deep Learning (DL)
What is Machine Learning?
Machine Learning is the science of enabling computers to learn from data without being explicitly programmed. The main categories include:
Supervised Learning: In this type, the model is trained on labeled data with known outcomes.
Unsupervised Learning: Here, the model seeks to find patterns in unlabeled data.
Reinforcement Learning: The model learns to perform actions through interaction with an environment.
Introduction to Deep Learning
Deep Learning is a subset of ML that focuses on artificial neural networks with multiple layers. This architecture enables the model to learn complex patterns and representations. Types of neural networks include:
Neural Networks
Convolutional Neural Networks (CNN)
Recurrent Neural Networks (RNN)
Differentiating between ML and DL
Scale and Complexity: DL is capable of handling much larger and more complex datasets than traditional ML.
Performance: In general, DL models can achieve higher accuracy, particularly in tasks like image and speech recognition.
Data Requirements: DL models typically require a larger volume of data for effective training.
Machine Learning Techniques for Global Temperature Prediction
Regression Analysis
Regression models are used to predict a continuous outcome, such as temperature:
Linear Regression: Assumes a linear relationship between the input features and the output.
Polynomial Regression: Useful when the relationship between input and output is non-linear.
Time Series Forecasting
Time series forecasting is crucial for predicting temperature trends:
Autoregressive Integrated Moving Average (ARIMA): A popular method for time series forecasting which captures various temporal structures.
Seasonal Decomposition of Time Series (STL): This method decomposes a series into seasonal, trend, and residual components, making it particularly useful for seasonal data.
Decision Trees and Random Forests
Decision trees are simple yet powerful models that split the data into branches to make predictions. Random forests combine multiple decision trees to make more accurate predictions.
Evaluating Model Performance
It is essential to assess how well a model performs:
Mean Absolute Error (MAE): The average absolute difference between observed actual outcomes and predictions made by the model.
Root Mean Square Error (RMSE): It’s similar to MAE but squares the differences before averaging them.
R-squared: Indicates the proportion of the variance in the dependent variable that is predictable from the independent variables.
Deep Learning Techniques for Global Temperature Prediction
Convolutional Neural Networks (CNNs) for Spatial Data
CNNs excel in analyzing visual data and are effective for processing spatial data such as satellite images:
Understanding Convolutional Layers: These layers apply filters to local features, detecting patterns such as edges.
Application to Climate Data: CNNs can analyze satellite images to detect changes in land and sea patterns.
Recurrent Neural Networks (RNNs) for Time Series Data
RNNs are designed for sequential data such as time series or natural language:
Understanding Recurrent Layers: Recurrent layers have loops to allow information persistence.
Long Short-Term Memory (LSTM): A special kind of RNN capable of learning long-term dependencies, which is useful for predicting climate patterns.
Application to Climate Data: Predicting temperature trends based on historical data.
Fine-tuning and Optimization
Dropout: A regularization technique that prevents overfitting.
Batch Normalization: Helps in faster convergence during training.
Evaluating Deep Learning Models
Loss Function: Measures how well the model is performing.
Accuracy: The proportion of true results among the total number of cases.
Overfitting and Underfitting: Ensuring that the model generalizes well to new data.
Implementation with Deep Learning: A LSTM Approach
Long Short Term Memory (LSTM) networks are a special kind of Recurrent Neural Network (RNN) that are well-suited to learning from data on long-term dependencies. They can be used effectively to model time series data like global temperatures.
Creating Synthetic Climate Data
Before we dive into real-world data, let's begin with a synthetic dataset to understand the LSTM model's working. Our synthetic data will represent average global temperature over a century, with a yearly time step. We'll simulate some cyclical patterns to represent seasonal changes and a small upward trend to represent global warming.
import numpy as np
import pandas as pd
# Generate synthetic time data
time = np.arange(0, 100, 1)
# Simulate a global warming trend
trend = 0.01 * time
# Simulate seasonal patterns
seasonal_pattern = np.sin(time * 2 * np.pi / time.max())
# Combine trend and seasonal patterns
temperature = trend + seasonal_pattern
# Add some random noise
temperature += np.random.normal(scale=0.5, size=time.size)
# Create a pandas dataframe
climate_df = pd.DataFrame({'Year': time, 'Temperature': temperature})
Training the LSTM Model
After creating the synthetic data, we can train an LSTM model using a portion of the data and then test the model's predictions against the remaining data. Here, we will use 80% of the data for training and 20% for testing.
from sklearn.model_selection import train_test_split
from keras.models import Sequential
from keras.layers import LSTM, Dense
# Preprocess data for LSTM
def create_dataset(X, y, time_steps=1):
Xs, ys = [], []
for i in range(len(X) - time_steps):
Xs.append(X.iloc[i:(i + time_steps)].values)
ys.append(y.iloc[i + time_steps])
return np.array(Xs), np.array(ys)
# Create LSTM dataset
TIME_STEPS = 10
X, y = create_dataset(climate_df['Year'], climate_df['Temperature'], TIME_STEPS)
# Split into training and test data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Here X_train and X_test need to be reshaped
X_train = X_train.reshape(X_train.shape[0], X_train.shape[1], 1)
X_test = X_test.reshape(X_test.shape[0], X_test.shape[1], 1)
# Then define the model
model = Sequential()
model.add(LSTM(units=50, input_shape=(X_train.shape[1], X_train.shape[2])))
model.add(Dense(1))
# Compile and train the model
model.compile(loss='mean_squared_error', optimizer='adam')
history = model.fit(X_train, y_train, epochs=20, batch_size=32, validation_split=0.1, verbose=0)
Model Evaluation and Prediction
After training the model, we evaluate it using the testing dataset. We'll use root mean square error (RMSE) as the evaluation metric.
from sklearn.metrics import mean_squared_error
# Predict on test data
y_pred = model.predict(X_test)
# Calculate RMSE
rmse = np.sqrt(mean_squared_error(y_test, y_pred))
print(f'Test RMSE: {rmse:.3f}')
The RMSE gives us an indication of the error we can expect from the model's predictions. Lower RMSE values indicate better fitting models.
In practice, you would replace the synthetic data with real-world climate data and follow a similar process, fine-tuning the model as necessary to improve its predictive performance. The understanding gained from this synthetic data implementation will be helpful when working with real-world data, and LSTM's architecture can be particularly useful for capturing long-term dependencies in global temperature data.
Challenges and Future Prospects
Challenges in ML/DL for Climate Prediction
Data Availability and Quality: Obtaining comprehensive and high-quality data is challenging.
Model Interpretability: DL models are often criticized as "black boxes" because it’s difficult to understand why they make certain predictions.
Computation Resources: DL models often require substantial computing power.
Future Prospects and Developments
Federated Learning: Allows for model training across many decentralized data sources, which is particularly useful when data cannot be shared easily due to size or privacy concerns.
Transfer Learning: Utilizing pre-trained models on a different but related problem can reduce resource requirements.
Climate-Informed AI: Combining traditional climate models with AI to enhance predictions.
Ethical Considerations and Responsible AI
As AI plays an increasingly significant role in climate science, it is critical to consider ethical dimensions including transparency, fairness, and the unintended environmental impact of computing.
Conclusion
Machine Learning and Deep Learning offer powerful tools for predicting global temperature changes. These technologies, combined with the ever-growing datasets available, could revolutionize climate science. However, challenges such as data quality, model interpretability, and computational costs must be addressed. Through continued research, collaboration between climatologists and AI experts, and careful consideration of ethical implications, ML and DL can play a pivotal role in our global response to climate change.
Check how much have you understood by answering the following question.