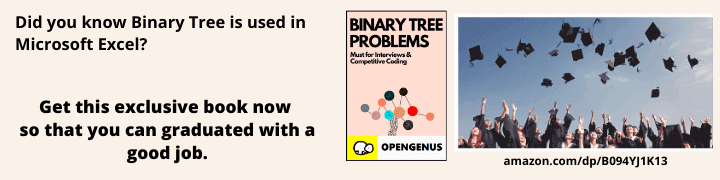
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 35 minutes | Coding time: 10 minutes
In this article, we will understand the basic concepts in MongoDB and go through an example of creating and updating a document as well.
Before going into MongoDB, we will visit the idea of NoSQL and relational database.
📝 NOSQL Database vs Relational Database
Before we learn about MongoDb, let us understand the difference between NoSql database and relational database.
Relational Database -
- It consists of structured data, stored in tables.
- It has static schema i.e all rows will have same kind of schema.
- It is mostly queried through Structured Query Language(SQL).
- It has a rigid schema and is bound to relationship.
NOSQL Database -
- It is a non-relational kind of database.
- It consists of unstructured data, stored in JSON format.
- It has dynamic schema i.e the schema is not fixed for all documents.
- It is very flexible and has non-rigid schema.
In NONSQL databases, documents correspond to rows and collection corresponds to table of Realtional Database.
📝 MongoDb
MongoDb is a NoSQL Database. The other type of database that we've been traditonally using is relational database.
In MongoDb, each database can contain one or more c
ollection. A collection basically refers to a table in Relational Database. Then, each collection can contain one or more documents, where document is a data structure respresenting a row in a table.
So, basically a document contains info about a single entity, and collection is like a parent structure that containes all the entities.
For instance, a recipe collection for all recipes. Here each recipe will be considered as a document.
Going with the official definition,
MongoDB is a document database with the scalability and flexibility that you want, and with the querying and indexing that you need.
Now let's understand what this means. We already know that MongoDB stores data in documents which are field-value paired data structures like JSON. Hence, we can say that it is document-based database.
In addition, Mongo provides built-in scalability through Sharding i.e distributing data across several machines and facilitating high throughput operations with large sets of data.
Mongo is also very flexible as it is not required to define a schema for the document beforehand, hence each document can have altogether different fields.
MongoDB is also a free and open-source database, published under the SSPL license.
So in summary, we can say that MongoDB is a great database system to build all kinds of modern, scalable and flexible web applications.
📝 Document structure in MongoDb :
MongoDB uses a data format similar to JSON for data storage called BSON. It looks like JSON, but it's typed, meaning that all values will have a data type such as string, Boolean or Number.
Now just like JSON, these BSON documents will also have fields and data stored in key value pairs.
⭐ Install MongoDb -
To install MongoDb on Windows, follow the instructions -
1, Download the .msi file from the downloads section .
- After it is downloaded, open the file in the file explore and double click on it to install Mongo.
- Follow the following prompts to install MongoDB -
- Once it is sucessfully installed, start the mongo shell using by traversing to
C:\path\to\mongodb\bin\mongod.exe
using terminal. - Connect to the MongoDB shell, using
C:\path\to\mongodb\bin\mongo.exe
.
This starts your Mongo Shell.
To install MongoDb on Linux, follow the instructions here.
To install MongoDb on macOs, follow the instructions here.
⭐ Creating a Local Database -
After you've installed mongo successfully, open the Mongo shell. Use command mongo
in your terminal to open it.
📝 Creating a new database -
- Use the command
use mydb
to create/switch to the database named as "mydb".
If the database specified does not exist, Mongo creates a new database with the specified name, and switches to it.
- Now, to create collections, use the following syntax -
db.<collection-name>.insertOne()
Here, db refers to the current database
, also to insert documents , you can use methods such as insertOne()
, insertMany()
etc.
We will learn more about insert operations in CRUD section.
- To see all the documents of a collection, use the command -
db.<collection-name>.find()
here, we use db.users.find()
- To see all the databases, use command
show dbs
.
- To see all the collections of the current database, use command -
show collections
- To quit MongoDb, use
quit()
⭐ CRUD - Creating Documents
To create Documents, we can use methods such as insertOne()
and insertMany()
. Since, we have already used insertOne()
, let us use insertMany()
-
insertMany()
takes in array of objects as input. The object here refers to the document that we want to insert in our collections.
As the result of our query, we receive an object, in which acknowledged:true
means that our query was semantiaclly correct and has been acknowldged by Mongo Db. Also, Mongo sends back the automatically generated IDs for the documents that we inserted.
Note : Here both of the documents have different schema, this is what gives MongoDb its flexibility.
⭐ CRUD - Reading and Query Documents
- To read a document, the easiest way is to use
find()
, which we have already used before. - Now, to query and search for a specific document, we can send the query object as a parameter to the
find()
object.
Here, we wanted to search for the document with name:"Garlic Noodles"
, and mongo returns us the associated document.
- To query the document on the basis of some condition, we have some operators such as
$lte
,$gte
,gt
,lt
and$ne
.
where
- $lte means less than equal to
- $gte means greater than equal to
- $gt means greater than
- $lt means less than
- $ne means not equal
Suppose, we need to get recipes with amount less than 3. We use the following query-
db.recipes.find({amount:{$lt:3}})
Now, let's fetch recipes that have amount lesser than or equal to 3.
Now, let's fetch recipes that have amount greater than 2.
⭐ CRUD - Updating Documents
Our collection recipes
looks like this as of now -
📝 1. Update a single document
We can use updateOne()
to update a document, it is similar to insertOne()
.
It takes in two parameters - the query and the update object
. The query object specifies the document that needs to be updated, and the update oject specifies the attributes that needs to be updated.
We use the $set
operator to set the new values to the object -
Note, how Mongo returns an object that consists of all the information of how the query affected the database.
📝 2. Update many documents
We can use updateMany()
to update all the documents that meet a specific criteria.
Let's update all the documents with amount:3
.
As you may see from the result that modifiedCount:2
which means that both of our documents with amount:2
have been updated.
Now, our collection looks like this - we use db.recipes.find()
to see the collections.
As you may have noticed, the value of amount has been updated for all our documents.
⭐ CRUD - Deleting Documents
📝 1. Delete single document
We have deleteOne()
to delete a single document. deleteOne
deletes the first document matching your query.
It accepts an object specifying the object that needs to be deleted. For instance -
In the above example, we have deleted the document that has name:"Pasta"
.After it is deleted, we get the response as acknowledged:true
which means that our request was successfully acknowledged and it deleted 1 document as specified by deletedCount:1
.
📝 2. Delete multiple documents
We use deleteMany()
to delete multiple documents. It deletes all the documents that meet the query object.
For instance,
Here, we have deleted all the documents that have amount less than equal to 10, as specified by {amount:{$lte:10}}
. We had two documents that had amount less than or equal to 10, and both of them got deleted as specified by deletedCount:10
.
⭐ When to Use MongoDb ?
Mongo DB is good for the following cases -
- For apps that need real time analytics and high scalability.
- For apps that deal with blogging and Content Management.
- When there's a need to maintain geospatial data.
- For building social networking platforms.
- For applications that maybe prone to changes, and have loosely coupled objectives.
For instance- Adobe, ebay, Google, Facebook, Nokia etc. all use MongoDb. To learn more about MongoDb customers, you may refer here.
⭐ When not to Use MongoDb ?
MongoDb should not be used for -
- For apps that have tightly coupled objectives and architecture.
- For apps that are highly transactional.
- And for applications whose data model is designed upfront.
And we're all done ! 🎉