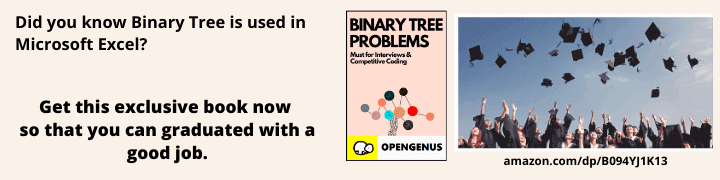
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
If you know the object, then you will easily get the Javascript.Object is the complex data type in javascript and it is used to represent collection of data and functionality.
Objects in JavaScript are created using many ways:
1. Object Literal notation:
You can create an object using the object literal notation, which is simply a comma-separated list of name-value pairs enclosed in curly braces {}. For example:
let car={
model:"city",
year:2022,
autoGear:true,
getCarDetails:function()
{
console.log(this.model+" "+this.year+" manufactured");
}
};
2. Constructor function:
You can create an object using a constructor function. A constructor function is a function that is used to create objects of a particular type. Here's an example:
function Car(model,year,autoGear){
this.model=model;
this.year=year;
this.autoGear=autoGear;
}
const honda = new Car("city",2022,true);
3. ES6 Classes:
You can create objects using ES6 classes. A class is a template for creating objects, similar to a constructor function. Here's an example:
class Car{
constructor(model,year,autoGear)
{
this.model=model;
this.year=year;
this.autoGear=autoGear;
}
}
const honda = new Car("city",2022,true)
4. Object.create():
You can also create an object using Object.create() method. This method creates a new object with the specified prototype object and properties. Here's an example:
const car = Object.create(null);
car.model = 'city';
car.year = 2022;
car.autoGear = true;
Nested object
- In JavaScript, a nested object refers to an object that is contained within another object. This means that the value of a property in an object can be another object. Nested objects are a common way to represent complex data structures and hierarchies.
Here is an example of a nested object:
const student = {
name: "Dharmik",
age: 18,
address: {
street: "123 Skyrise",
city: "Ahd",
state: "GJ",
zip: "388620"
},
marks:[
{"phy":95},
{"maths":80},
{"chem":89}
]
};
In this example, the student object has four properties: name, age, address and marks. The address property is a nested object that contains four properties: street, city, state, and zip.The marks property is a array of object which contains the marks of the various subject with key value pair.
To access the properties of a nested object, you can use dot notation or bracket notation. For example, to access the city property of the address object in the person object, you can use either of the following:
person.address.city
or
person["address"]["city"]
How to access property or method of object
- To access a property or method of an object, you can use dot notation or bracket notation:
console.log(car.model); // Output: "city"
console.log(car["year"]); // Output: 2022
car.getCarDetails(); // Output: "city 2022 manufactured"
In the first example, we use the dot notation to access the model property of the car object. In the second example, we use the bracket notation to access the year property. The bracket notation is useful when you need to access a property whose name is stored in a variable or when the property name contains spaces or special characters.In the third example, we use the dot notation to access the function of an object which will give the output "city 2022 manufactured".
- JavaScript objects are mutable, which means that you can add, modify, and delete properties and methods at any time:
car.model="civic";
car["fuel"]="petrol";
delete car.year;
In the first line, we use the dot notation to modify the value of the model property of the car object. In the second line, we use the bracket notation to add a new fuel property to the car object.The third line represent how we can delete the any one of the property of object.
Object Methods
In addition to properties, objects can also have methods, which are functions that are associated with the object. Methods are useful when you want to encapsulate a set of related operations or behaviors within an object. Here's an example of an object with a method:
const calculator = {
add: function(a, b) {
return a + b;
},
subtract: function(a, b) {
return a - b;
},
multiply: function(a, b) {
return a * b;
},
divide: function(a, b) {
return a / b;
}
};
In this example, we have created an object called calculator that has four methods: add, subtract, multiply, and divide. Each method takes two parameters and performs a mathematical operation on them.
You can call an object's method using the dot notation. Here's an example:
console.log(calculator.add(2, 3)); // Output: 5
In this example, we call the add method of the calculator object with two arguments (2 and 3), which returns the sum of the two numbers (5).
- Built-in methods
In JavaScript, objects have a number of built-in methods:
- Object.assign(target, ...sources): This method is used to copy the values of all enumerable properties from one or more source objects to a target object.Below is the example.
const target = { "aadhya": 1, "bobby": 2 };
const source = { "bobby": 4, "kundan": 5 };
const result = Object.assign(target, source);
console.log(result); // { "aadhya": 1, "bobby": 4, "kundan": 5 }
- Object.entries(obj): This method returns an array of key-value pairs for each enumerable property on an object.
Here is the example:
const student = { name: 'Dharmik', enrollment: 76 };
const entries = Object.entries(student);
console.log(entries); // [ ['name', 'Dharmik'], ['enrollment', 76] ]
- Object.keys(obj): This method returns an array of all the enumerable property names of a given object.Let's see example.
const obj = { "aadhya": 1, "sher": 2, "dax": 3 };
const keys = Object.keys(obj);
console.log(keys); // ['aadhya', 'sher', 'dax']
- Object.freeze(obj): This method prevents an object from being modified by preventing new properties from being added, existing properties from being removed, or existing properties from being changed.
const obj = { a: 1, b: 2 };
Object.freeze(obj);
obj.a = 5; // Error: Cannot assign to read only property 'a' of object
console.log(obj); // { a: 1, b: 2 }
- These are just a few examples of the many built-in methods available in JavaScript's Object class.
conclusion:
In JavaScript, objects are one of the fundamental data types and are used to store and organize data. Objects can be created using the object literal syntax, constructor functions, or classes.
Objects consist of properties, which are key-value pairs, and methods, which are functions attached to the object. Properties can be accessed and modified using dot notation or bracket notation.
Objects can also be used to create more complex data structures, such as arrays, maps, and sets.
In conclusion, objects are a crucial component of JavaScript and are used extensively in web development and other applications. Understanding how to create, manipulate, and work with objects is essential for developing robust and scalable JavaScript applications.