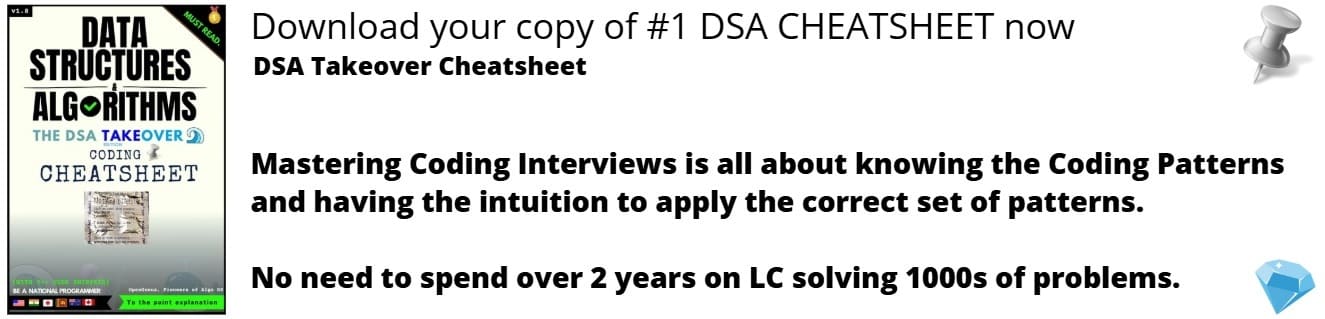
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have take a look at how to print a rectangular matrix of size 2 * 3 with integers in C Programming Language with numbers from 1 to 6.
The pattern is as follows:
246
135
Table of contents
- Problem statement
- Approach to solve
- Implementation
- Output
Problem statement
In this problem, we have to print the following rectangular matrix of size 2*3:
246
135
Approach to solve the problem
Following is the approach to solve this problem:
-
We can solve this problem by using 2D array.
-
First we declare a variable a[2][3] where 2 represents the number of rows and 3 represents the number of columns. We also declare a variable count and initialise it with a value 1.
-
Then we run two loops-
-
Outer loop for the rows and inner for the columns.
-
The positions of the elements in the matrix are as follows-
2-(0,0) 4-(0,1) 6-(0,2)
1-(1,0) 3-(1,1) 5-(1,2)
-
In each of the columns the second coordinate of the element is same and the first coordinate varies by 1 or 0. So we assume the second coordinate value to be in the outer loop and the first coordinate value to be in the inner loop.
-
The second coordinate values vary from 0 to 2 so the outer loop runs from 0 till 2.
-
The first coordinate values vary from 0 to 1. The first element lies in (1,0), second in (0,0), third in (1,1) and so on.
So the inner loop runs from 1 to 0 while decrementing its value by 1.
Inside this loop we take assign j as row and i as column and assign the value of count and increment count by 1 in the next line. -
Initially the value of i=0, we enter the loop. The value of j=1 so a[j][i]=a[1][0] i.e. 1, then j=0 so a[j][i]=a[0][0] i.e. 2 as we had incremented the value of count.
Now j=-1 which doesn't satisfy the condition of j>=0 so it exits the loop and cursor goes to next line due to the command"printf("\n")"
. This way the loop continues and we get the desired output.
Implementation
Following is the implementation of the code in c programming language-
#include<stdio.h>
int main()
{
int i,j, count=1,a[2][3];
for(i=0;i<3;i++)
{
for(j=1;j>=0;j--)
{
a[j][i]=count;
count++;
}
}
for(i=0;i<2;i++)
{
for(j=0;j<3;j++)
{
printf("%d",a[i][j]);
}
printf("\n");
}
return 0;
}
Output
The command to run the program is:
gcc code.c
./a.out
The output will be:
246
135
With this article at OpenGenus, you must have the complete idea of printing the given pattern.