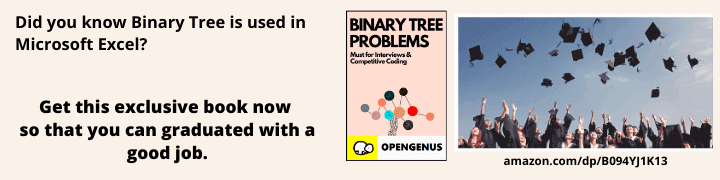
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we present the approach to implement a Python script that can refresh an URL / Tab in the browser. Manually, this can be done by pressing F5 key.
Table of contents
- About automation
- Package dependencies
- Setting up environment
- Hands on: Writing the browser refresh script
About automation
When I was still attending high school, one of the core attributes of computers that we were taught was diligence(Work for long hours and with high accuracy).
And with the prowess computers have exhibited, the statement 'Programmers will be replaced with computers' might ring a bell. Well uncle Bob clears the mist around that statement.Its worthy reading by yourself,...
To wield the power entrusted to us, we use programming languages to instruct computers on what to do and how to do it.
In this article, I will be using python (particularly python3) to demonstate a just a shred of its automation power. We shall write a script that refreshes(reloading a page) the browser for us automatically.
Package dependencies
Make sure you have the Firefox browser and python3 already installed.
In addition to the above dependencies, we shall also need selenium python package which we are going to install presently.
Selenium is a package that is used for browser automation.
I am more of a visual guy, so I would like you to picture a robot sitting infront of your computer making that amazon order instead of you doing it.
Most of the web browsers follow the W3C WebDriver Interface and it is this interface that allows introspection and control of browser programs by other programs.
Selenium simplifies it further and provides us with a more user friendly interface (abstraction) to the interact with the WebDriver interface implemeted in the browsers.
Setting up environment
We need an environment to operate in, and to localize our effects.
I encourage you to use a Linux based or Unix operating system to follow along smoothly.
virtualenv -p /usr/bin/python3 python-automation-sandbox
The above command creates an enviroment with the name python-automation-sandbox having base packages and in addition to that, I am pointing out the python interpreter to use for my enviroment.
Lets go ahead and acitvate the environment.
cd ~/python-automation-sandbox; source bin/activate
NOTE: My environment is in my home directory, yours might be in a different location depending on what was your current working directory when you created the environment.
Installing selenium with pip
pip install selenium
Selenium requires driver binaries that talk to the browsers and currently selenium supports Firefox, Chrome, Internet Explorer, Edge, Safari, Opera.
We shall be using firefox and the drivers are available on Mozilla Github repository
Installing firefox browser drivers (Linux)
- Extract the browser drivers. You may have noticed files ending .asc when downloading the drivers, those are PGP signatures that you can use to verify that the drivers have not been tampered with.
tar -xvf ~/Downloads/geckodriver-v0.31.0-linux64.tar.gz
- Make the geckodriver file executable
sudo chmod u+x geckodriver
- Move the geckodriver file to location /usr/local/bin
mv geckodriver /usr/local/bin
And thats it...
Hands on: Writing the browser refresh script
We only need one file. I have also placed it under a directory just to organised.
import time
from selenium import webdriver
We begin by importing the webdriver module from the selenium package and the time module.
The webdriver has all implementations of drivers for the supported browsers as I stated above.
url = "https://example.com"
waiting_duration_before_refresh = 5
driver = webdriver.Firefox()
driver.get(url)
We move on to creating a driver instance for Firefox browser. This is what we use to control the browser.
The get() method is used for loading a page in a tab. Similar to the way you enter an address like example.com in the search bar and then hit Return key.
The waiting_duration_before_refresh is just for you to visualize while selenium refreshes the page since to me, It happened before I could even see it.
assert "Example" in driver.title
The above line is just an assertion test to make sure that the page was loaded successfully and the title attribute of the driver instance holds the title of the web page.
time.sleep(waiting_duration_before_refresh)
driver.refresh()
After the browser successfully loading our page, then we wait for 5 seconds and then refresh the browser using the refresh() method of the driver instance.
Full Code
#!/usr/bin/python
import time
from selenium import webdriver
url = "https://example.com"
waiting_duration_before_refresh = 5
driver = webdriver.Firefox()
driver.get(url)
assert "Example" in driver.title
time.sleep(waiting_duration_before_refresh)
driver.refresh()
The code is also available on github
Congratulations, getting up to this point, the driver instance has a multitude of methods and attributes that you can use to automate more complex tasks. You can read about them in this documentation
Let me end on this note, Programmers are here to stay and don't expect them to be replaced with some robot.