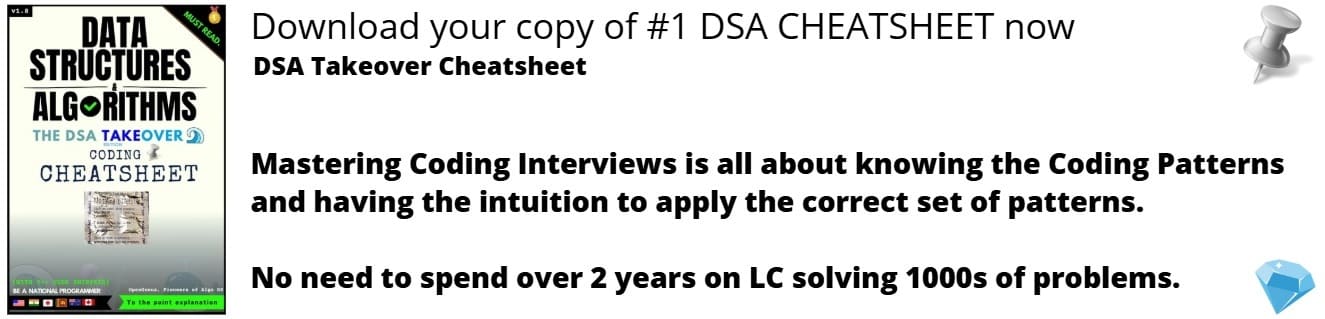
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Set is a data structure that stores elements. It is a simple yet effective data structure when used correctly. In C++'s Standard Template library (STL), we have set as a container that is used to store the values which are unique i.e no value in a set can be repeated or edited. If you want to edit the value added by you the only way is to remove the wrong element and add the correct one.
To understand rbegin and rend function of set, let us revisit the concept of iterators and reverse iterators:
- Iterator in C++ is an object that points to an element in a container. It can be used to traverse elements in the container (such as set, linked list and others).
There are different types of iterators which different functionalities one of which is reverse iterators.
- reverse iterators is an iterator that reverses the direction of a given iterator. When provided with a bidirectional iterator, std::reverse_iterator produces a new iterator that moves from the end to the beginning of the sequence defined by the underlying bidirectional iterator.
The onl difference between begin(), end() and rbegin(), rend() is that:
- begin() and end() will return bidirectional iterator
- rbegin() and rend() will return reverse iterator
set::rbegin()
It is a built-in function which returns a reverse iterator pointing to the last element in the container.
Syntax:
reverse_iterator set_name.rbegin()
- Parameters: No parameter.
- Return value: The function returns a reverse iterator pointing to the last element in the container.
set::rend()
It is an in-built function which returns a reverse iterator pointing to the element right before the first element in the set container.
Syntax:
reverse_iterator set_name.rend()
- Parameter: No parameter
- Return value: The function returns a reverse iterator pointing to the element right before the first element in the set container.
Example 1: Print set in reverse order
In this example we define a set of integer and with the help of reverse iterator print the reverse order of our set.
#include <iostream>
#include <set>
using namespace std;
int main()
{
set<int> myset{1, 2, 3, 4, 5};
for (auto it=myset.rbegin(); it != myset.rend(); it++)
cout << ' ' << *it;
return 0;
}
Output :
5 4 3 2 1
Example 2
In this example we define a set of character and with the help of reverse iterator print the reverse order of our set.
#include <iostream>
#include <set>
using namespace std;
int main()
{
set<char> myset{'a', 'b', 'c', 'd'};
for (auto it=myset.rbegin(); it != myset.rend(); it++)
cout << ' ' << *it;
return 0;
}
Output :
d c b a
Example 3
In below example we define objects of our class with a value and then print the reverse of our object decleration.
#include<bits/stdc++.h>
using namespace std;
class Test
{
public :
int id;
bool operator<(const Test& t) const
{
return (this->id < t.id);
}
};
// Driver method
int main()
{
// put values in each
// structure define below.
Test t1 = { 10 }, t2 = { 20 },
t3 = { 90 }, t4 = { 100 };
set<class Test> s;
// insert structure in set
s.insert(t1);
s.insert(t2);
s.insert(t3);
s.insert(t4);
// define an iterator to iterate the whole set.
set<class Test>::reverse_iterator it;
for (it = s.rbegin(); it != s.rend(); it++)
{
cout << (*it).id << endl;
}
return 0;
}
Output:
100
90
20
10
Errors and Exceptions
- No exception throw guarantee.
- Shows error when a parameter is passed.
Use
rbegin and rend is mainly used to traverse a given set in reverse order efficiently.