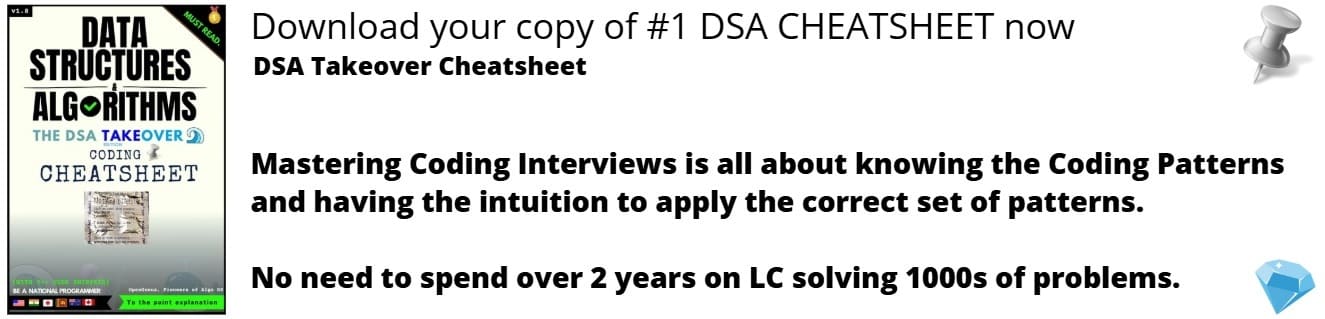
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Training, testing, validation, and holdout sets are essential components of machine learning models that allow for effective evaluation of model performance and generalization. In this article, we will delve into what these sets are, how they are used, and why they are important.
Machine learning models aim to make predictions about unseen data based on patterns learned from a training set. However, the performance of these models on the training set does not necessarily guarantee good performance on new data. This is where testing, validation, and holdout sets come into play.
The training set is used to train the model, while the testing set is used to evaluate the model's performance on unseen data. In addition to the testing set, the validation set is also used to fine-tune the model during the training process. Lastly, the holdout set is a final evaluation set that is only used once the model has been fully trained and optimized.
1. Training Set:
The training set is a portion of the data used to train the machine learning model. It is the input data used to learn the parameters of the model. This set is used to develop a model that can learn and recognize patterns within the data.
The size of the training set is crucial as it can have a significant impact on the performance of the model. A larger training set is generally preferred as it allows for the model to learn more complex patterns within the data, leading to better generalization performance.
2. Testing Set:
The testing set is a portion of the data that is held out from the training set and is used to evaluate the performance of the model on unseen data. The testing set is essential to evaluate the generalization performance of the model. It allows us to evaluate how well the model has learned the patterns within the data and how well it can predict new data.
It is important to note that the testing set should not be used during the training process, as this can lead to overfitting. Overfitting occurs when a model performs well on the training data but poorly on the testing data, indicating that it has not generalized well.
3. Validation Set:
The validation set is used to fine-tune the model during the training process. This set is used to evaluate the performance of the model during training and adjust the model's hyperparameters accordingly. The hyperparameters are variables that cannot be learned from the data, such as the learning rate, regularization parameter, etc.
The validation set is used to prevent overfitting during the training process. By monitoring the model's performance on the validation set, we can identify when the model is starting to overfit and adjust the hyperparameters accordingly. This can lead to a more accurate and robust model.
4. Holdout Set:
The holdout set is a final evaluation set that is only used once the model has been fully trained and optimized. The holdout set is essential to evaluate the final performance of the model on completely unseen data. It is important to have a holdout set to ensure that the model has not overfit to the training, validation, or testing sets.
The size of the holdout set is also crucial. A larger holdout set can provide a better estimate of the model's generalization performance, but it can also reduce the amount of data available for training and validation.
Implement these sets using Python's scikit-learn library
from sklearn.model_selection import train_test_split
# split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# split the training set into training and validation sets
X_train, X_val, y_train, y_val = train_test_split(X_train, y_train, test_size=0.2, random_state=42)
# split the holdout set from the original data
X_holdout, y_holdout = X_test, y_test
The first line of the code uses train_test_split() to split the original dataset X and y into two sets: the training set (X_train and y_train) and the testing set (X_test and y_test). The test_size parameter is set to 0.3, which means that 30% of the original dataset will be used for testing, and the remaining 70% will be used for training.
The second line of the code further splits the training set into two sets: the new training set (X_train and y_train) and the validation set (X_val and y_val). The test_size parameter is set to 0.2, which means that 20% of the training set will be used for validation, and the remaining 80% will be used for training.
Finally, the third line of the code creates the holdout set by copying the testing set to X_holdout and y_holdout.
Applications:
The concept of Testing, Training, Validation, and Holdout sets is widely used in machine learning for developing and evaluating predictive models. These sets are used in a variety of applications, including image recognition, natural language processing, and financial modeling. For example, in image recognition, a model can be trained to identify different objects
Differences between Training, Testing, Validation and Holdout Sets:
Criteria | Training Set | Testing Set | Validation Set | Holdout Set |
---|---|---|---|---|
Purpose | To train the model parameters | To evaluate the model performance | To tune the hyperparameters | To estimate the model performance |
Size | Large | Moderate | Small | Moderate |
Shuffle | Yes | No | Yes | No |