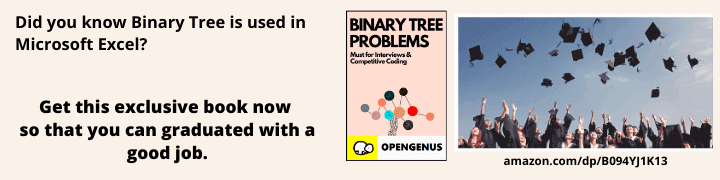
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we are going to talk about jest particularly in typescript scope, why should you test your code? Why use jest with typescript? And what developing method use?
Why test your code?
You may think that tests are not that important, you can console.log() and find out what your output is going to be, with just a typeof you can find out the type of something in your function, and the QA’s job are there for testing already right? So why should I learn testing?
Testing is essential for you when you are trying to get the best out of your code, you want to know about every parameter and make sure that you actually can analyze every aspect of your functions? Want to understand the system in and out? Want to make sure that the user experience is great? The QA’s test your pull requests and never accept because of a small bug or defect? Want to learn TDD to add to your curriculum?
Then you should learn how to test your code. In my opinion a great developer is not the one that knows every framework and can deal with bugs fast, a great developer can create a great base for your team, knowing how and why particular tools are used, searching about certain aspects of the system that they don’t know, and deep dives onto the system, so when something goes wrong he knows why it went wrong, how can the team avoid this bug, fixing it is good but fixing without knowing why something is fixed can make a mess on the system for next developers or future additions.
First, let’s think about what is typescript, in the official typescript site the description of the language is: “[…] is a strongly typed programming language that builds on JavaScript, giving you better tooling at any scale.”.
But if typescript already is a typed language you should already know what will the input and output type be, does that mean that jest is useless with it? No!
Typescript and Jest
Typescript can’t catch certain types of errors, so jest would be a complement for your development, it’ll improve your code quality, ensure that the system will go according to the expectations and will help the documentation too, for you to understand a codebase it’s much easier when it has tests.
Jest has an easy setup and configuration with typescript and JavaScript, it is the most famous testing tool of both languages, with the possibility of mocking functions, using test units and tracking the percentage of code coverage. There are also great documentation, a big community of users and a lot of tutorials on the internet, making your life easier when you are trying to learn about a framework, language, tool or database.
How to use ts and jest together
First you have to install the dependencies
npm install --save-dev jest @types/jest typescript ts-jest
Then you need to create a jest.config.js file at the root of your project to configure how typescript is going to work and to use ts-jest.
// jest.config.js
module.exports = {
preset: 'ts-jest',
testEnvironment: 'node',
// other configurations...
}
Now let’s write a really simple function to use as an example here:
export function sum(a: number, b: number): number {
return a + b;
}
After writing this function, let’s create a sum.test.ts file, so we can test this function with jest:
import { sum } from './sum';
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
To run this test you just need to use ‘npx jest‘ on your cmd, jest will automatically detect files with .test.ts.
TDD or BDD
Both are development methodologies that focus on testing, so the software can have it’s quality improved.
TDD is more of a developer testing, focusing on the technical side of the software, verifying if the code works the way it is intended to work. In TDD, you write the test first, so the code can fit the test and be analyzed. This process can be good for catching bugs in early development, but a lot of developers think that TDD can slow the development by having to make tests for each piece of functionality.
BDD is a methodology that bring the business side of the company on the development of the tests, this can help the developers see through the desires of the final users, making the perspective of how the system should behave for the costumer a very important part. This methodology can make the development slower too,
but it can catch bugs, inconsistency and resolve future complaint on the first MVP of the project.