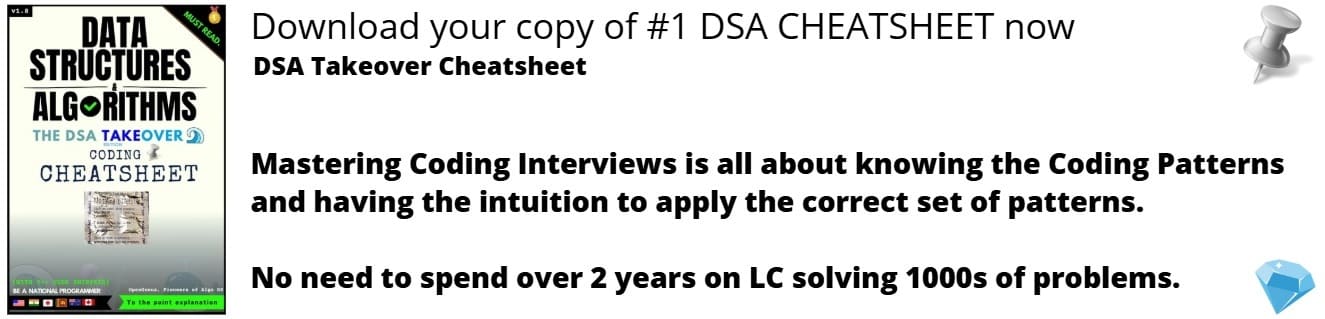
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
JavaScript is a popular programming language used for building interactive web applications. One of the key features that make JavaScript so powerful is its ability to receive input from users. In this article, we will explore the basics of user input in JavaScript and how it can be used to create dynamic and interactive web applications.
User input can be defined as any information that a user provides to a web application. This can include text, numbers, dates, images, and other types of data. User input is crucial for building interactive applications that respond to user actions in real-time.
There are several ways to capture user input in JavaScript. Let's take a look at some of the most common methods:
1. Form Input Fields: The most common method of capturing user input is through form input fields. HTML provides several types of input fields, including text fields, password fields, checkboxes, radio buttons, and dropdown menus. JavaScript can be used to access and manipulate the values entered in these fields.
2. Event Listeners: Event listeners can be used to capture user input when specific events occur, such as when a button is clicked or when a key is pressed. Event listeners are often used in conjunction with form input fields to create more dynamic and interactive user interfaces.
3. AJAX Requests: AJAX (Asynchronous JavaScript and XML) can be used to send and receive data from a web server without having to reload the entire page. This can be used to capture user input and send it to the server for processing, allowing for a seamless user experience.
4. Using the prompt() Method: The prompt() method is a built-in JavaScript function that displays a dialog box with a message to the user, a text input field, and two buttons (OK and Cancel).
Let's take a closer look at each of these methods and how they can be used to capture user input.
1. Form Input Fields
Form input fields are the most common method of capturing user input in JavaScript. HTML provides several types of input fields that can be used to capture different types of data.
-
Text Fields: Text fields are used to capture user input in the form of text. They are typically used for fields such as names, addresses, and messages.
-
Password Fields: Password fields are used to capture user input for passwords. They mask the input text so that it cannot be seen by other users.
-
Checkboxes: Checkboxes are used to capture user input for multiple choices. They allow users to select one or more options from a list of choices.
-
Radio Buttons: Radio buttons are used to capture user input for single choice. They allow users to select one option from a list of choices.
-
Dropdown Menus: Dropdown menus are used to capture user input for a list of choices. They allow users to select one option from a list of choices.
To access the value entered in a form input field using JavaScript, we can use the value property. For example, to access the value of a text field with the ID myText, we can use the following code:
<!DOCTYPE html>
<html>
<head>
<title>Using Form Input Fields in JavaScript</title>
</head>
<body>
<form>
<label for="myText">Enter some text:</label>
<input type="text" id="myText">
<button type="button" onclick="myFunction()">Submit</button>
</form>
<script>
function myFunction() {
var myText = document.getElementById("myText").value;
alert(myText);
}
</script>
</body>
</html>
This code retrieves the value of the text field with the ID myText and stores it in the variable myText.
Output:
2. Event Listeners
Event listeners can be used to capture user input when specific events occur, such as when a button is clicked or when a key is pressed. Event listeners are often used in conjunction with form input fields to create more dynamic and interactive user interfaces.
To add an event listener to an HTML element using JavaScript, we can use the addEventListener method. For example, to add an event listener to a button with the ID myButton, we can use the following code:
document.getElementById("myButton").addEventListener("click", myFunction);
This code adds an event listener to the button with the ID myButton that listens for the click event. When the button is clicked, the myFunction function will be called.
Inside the event listener function, we can access the value of form input fields using the same method as shown earlier.
For example, let's say we have a text field with the ID myText and a button with the ID myButton. When the button is clicked, we want to retrieve the value of the text field and display it in an alert message. We can use the following code:
<!DOCTYPE html>
<html>
<head>
<title>Using Event Listeners in JavaScript</title>
</head>
<body>
<label for="myText">Enter some text:</label>
<input type="text" id="myText">
<button type="button" id="myButton">Submit</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
var myText = document.getElementById("myText").value;
alert(myText);
});
</script>
</body>
</html>
This code adds an event listener to the button with the ID myButton that listens for the click event. When the button is clicked, the anonymous function is called. Inside the function, we retrieve the value of the text field with the ID myText and store it in the variable myText. We then display the value of myText in an alert message.
Have a look at output:
3. AJAX Requests
AJAX can be used to capture user input and send it to a web server for processing without having to reload the entire page. This can be used to create more dynamic and interactive web applications.
To send an AJAX request using JavaScript, we can use the XMLHttpRequest object. For example, let's say we have a form with a text field and a button. When the button is clicked, we want to send the value of the text field to a server-side script for processing. We can use the following code:
<!DOCTYPE html>
<html>
<head>
<title>Using AJAX Requests in JavaScript</title>
</head>
<body>
<label for="myText">Enter some text:</label>
<input type="text" id="myText">
<button type="button" id="myButton">Submit</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
alert(this.responseText);
}
};
xhttp.open("POST", "process.php", true);
xhttp.setRequestHeader("Content-type", "application/x-www-form-urlencoded");
var myText = document.getElementById("myText").value;
xhttp.send("myText=" + myText);
});
</script>
</body>
</html>
This code adds an event listener to the button with the ID myButton that listens for the click event. When the button is clicked, an AJAX request is sent to the server-side script process.php. The value of the text field with the ID myText is sent to the server-side script as a parameter named myText.
The XMLHttpRequest object has a onreadystatechange property that is set to a function that is called whenever the state of the request changes. In this function, we check if the readyState is 4 (which indicates that the request is complete) and the status is 200 (which indicates that the request was successful). If both of these conditions are true, we display the response text in an alert message.
4. The prompt() Method
The user can enter text into the input field and click OK to submit the input, or click Cancel to close the dialog box without submitting any input.
To use the prompt() method, we can call it with a string parameter that contains the message to be displayed to the user. For example:
<!DOCTYPE html>
<html>
<head>
<title>Using the prompt() Method in JavaScript</title>
</head>
<body>
<script>
var name = prompt("Please enter your name:");
alert("Hello, " + name + "!");
</script>
</body>
</html>
This code displays a dialog box with the message "Please enter your name:" and an input field. When the user enters their name into the input field and clicks OK, the prompt() method returns the input value as a string and assigns it to the variable name.
We can use the prompt() method to capture user input in a variety of ways. For example, we could use it to ask the user for their age, like this:
var age = prompt("Please enter your age:");
Or we could use it to ask the user for a yes/no answer, like this:
var answer = prompt("Do you want to continue? (yes or no)");
Once we have captured user input using the prompt() method, we can use it in our JavaScript code just like any other variable.
Conclusion
User input is an essential aspect of building dynamic and interactive web applications. JavaScript provides several methods for capturing user input, including form input fields, event listeners, AJAX requests and the prompt() method. By using these methods, developers can create web applications that respond to user actions in real-time, providing a seamless user experience.