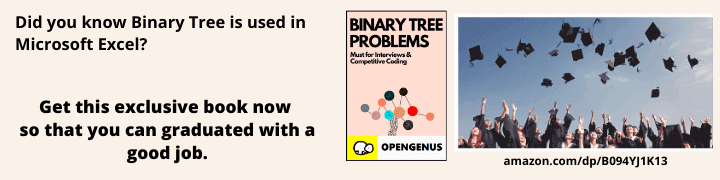
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered what is the With statement in Python and how to use it along with the idea of Context Manager in Python.
Table of contents:
- What is the With statement?
- Python Examples using the With statement
- How does the With statement work?
- Context Manager and using With in User Defined Objects
Let us get started with the exploration of With statement in Python.
What is the With statement?
The With statement is a keyword in Python is used to provide exception handling functionality that could be done with a try...except...finally block.
It is quite useful for managing resources by ensuring the safe acquisition and release of resources to avoid blocking other processes.
The With statement is especially used in streams such as file streams, locks, database connections etc.
Syntax of With statement
with expression as variable:
block
In the above code block:
- With and as are keywords
- Expression can be an arbitrary expression
- Variable holds the result of the expression evaluation
Python Examples using the With statement
1. Working with files
When working with files in Python, it's essential to close the file after performing read or write operations on it.
Without the with statement, we could use the try...finally approach to perform operations on the file.
- Using the try...finally approach
file = open('filename.txt','w')
try:
file.write('Hello World')
finally:
file.close()
Here the finally block enables us to close the file regardless of whether or not the write operation on the file was successful.
- Using the With statement
with open('filename.txt', 'w') as file:
file.write('Hello world!')
When using the With statement, we do not need to close the file as it can handle all the possible exceptions before closing the file.
How does the With statement work?
From the example given above, you may be wondering how the With statement works, how does the file get closed?
In this section, we have covered what goes on behind the scenes. The With statement works by using two Python magic methods __enter__
and __exit__
available on the given object.
When a file is opened in Python, it returns a file object. The file object has the __enter__
and __exit__
methods included in it.
The __enter__
method returns the file object itself while the __exit__
method closes the file.
Try running the following Python code on the terminal:
> file = open('myfile.txt')
__enter__
method
> file.__enter__()
Output
<open file 'myfile.txt', mode 'r' at 0x7f3b65d0bc00>
__exit__
method
> file.__exit__()
> file
Output:
<closed file 'myfile.txt', mode 'r' at 0x7f3b65d0bc00>
Context Manager and using With in User Defined Objects
A context manager is an object that has __enter__
and __exit__
methods.The open() function works with the With statement because the file object it returns implements the context manager protocol.
Any user-defined object can work with the With statement as long as it implements the context manager protocol.
Let's implement our context manager to have a deeper understanding of what happens when we use a With statement.
Run the code below to implement an open() function context manager.
class File:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, type, value, traceback):
if self.file:
self.file.close()
Usage
with File('filename.txt','w') as file:
file.write('Hello world!')
When the code above runs, the "Hello world!" text will be written to the file. The file will be closed without having to call the close() function.
Let's talk about how the code in the class works.
- The With statement stores the
__exit__
method of the File class. - It calls the
__enter__
method which opens the file and returns the file handle. - The file handle is then passed to the file variable which then performs the write operation on the file.
- The With statement then calls the
__exit__
method it stored earlier that closes the file.
With this article at OpenGenus, you must have a strong idea of how useful the With statement is in resource management and how to use it.