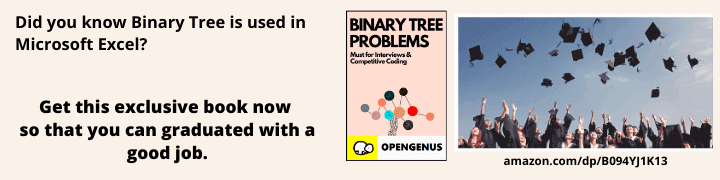
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
XMLHttpRequest can be defined as an API in the form of an object which is used to transfer data between the web browser and the web server. It gives us the ability to update parts of a web page without reloading the whole page.
The XML part in the XMLHttpRequest can sometimes create misunderstanding because XMLHttpRequest can not only work with data in the form of XML but also with JSON, HTML or plain text.
Create an XMLHttpRequest Object
var xhttp = new XMLHttpRequest();
Sending a request
open()
and send()
methods are of the XMLHttpRequest
object are used to send a request to the server.
xhttp.open(method, url, async);
xhttp.send()
Here we have
method
is a HTTP-method. Generally "GET" or "POST".url
is an address of a file on the web serverasync
is true (asynchronous) or false (synchronous)
By sending a request asynchronously, the JavaScript does not have to wait for the server response and in the meantime it can executes other scripts.
Getting a response
This code demonstrates how to make a GET request using XMLHttpRequest.
function getRequiredData() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
console.log(this.responseText);
}
};
xhttp.open("GET", "sone_valid_url", true);
xhttp.send();
}
onreadystatechange
It is used to define a function to be called when the readyState property changes.
readyState
It holds the status of the XMLHttpRequest. It can take the following values:
this.responseText stores the response text received from the GET request.
0 Request is not initialized
1 Request has been set up
2 Request has been sent
3 Request is in process
4 Request is completed
The status
and the statusText
holds the status of the XMLHttpRequest object
.
status
is a HTTP status code(a number): 200
, 403
, 404
.
statusText
is a HTTP status message (a string): usually OK
, Forbidden
, Page not found
and so on.
200: "OK"
403: "Forbidden"
404: "Page not found"
responseText
is used to get the response data from the web server as a string.
Code
Now that you have learnt how to make a request to the server and get you desired data from it, we will code a simple application to get current temperature of the day.
We will fetch data from the OpenWeatherMap API.
This file "index.html" defines the HTML interface to make the call. Once your function getLocation() gets loaded, it is called.
<!DOCTYPE html>
<html>
<head>
<title>Weather App</title>
</head>
<body onload="getLocation();">
<p id="temperature"></p>
</body>
<script src="script.js" ></script>
</html>
script.js
This function getLocation() with get the current location of the user using the geolocation API of Navigator DOM object.
function getLocation() {
if (navigator.geolocation){
navigator.geolocation.getCurrentPosition(showPosition);
} else {
alert("Geolocation is not supported by this browser.");
}
}
This function showPosition() extracts the latitude and longitude from the value of the above function and uses the OpenWeatherMap API to make a GET request and get the temperature value.
function showPosition(position) {
// getting latitude and longitude coordinates
var latitude = Math.floor(position.coords.latitude);
var longitude = Math.floor(position.coords.longitude);
// key to access OpenWeatherMap API
var key = "must be some valid key from OpenWeatherMap";
// path to fetch data
var url = "https://api.openweathermap.org/data/2.5/weather?lat=" + latitude +"&lon=" + longitude + "&appid=" + key + "";
// fetching data
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var myObj = JSON.parse(this.responseText);
document.getElementById("temperature").innerHTML = myObj.main.temp;
}
};
xmlhttp.open("GET", url , true);
xmlhttp.send();
}
To get a free valid API key, create an account on OpenWeatherMap .
If you're not satisfied with this one, you could choose and try another free weather API.
Here we are using HTML5 geolocation
to fetch the latitude
and longitude
coordinates of the user.
These are required as we are calling the API using the geographical coordinates of the user.
JSON.parse()
is used to convert the JSON reponse we got from the server to a JavaScript object.
So you just learnt how to call an API and fetch and display the response provided by the API.
For more details and work on the code of Weather Application
Refer to the following github repository weather App.