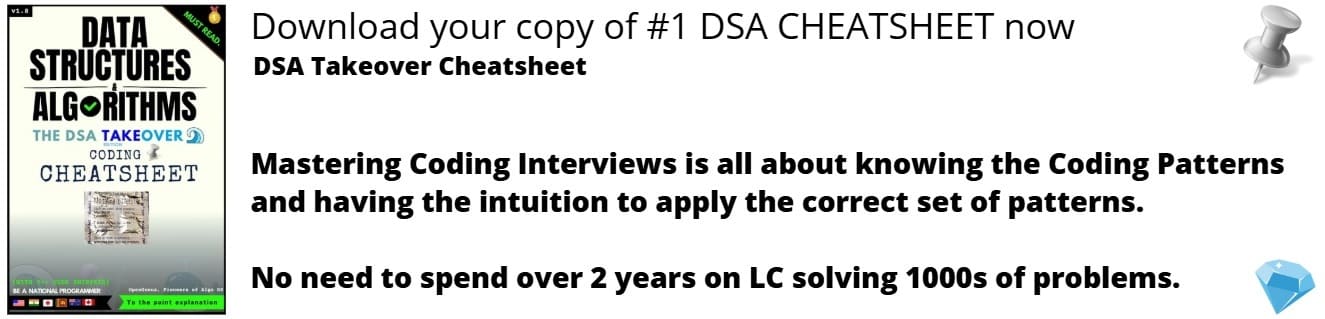
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
The continue statement is a jump statement in C. It is another one of the jump statements like the break statement as both the statements skip over a part of the code. But the continue statement is somewhat different from the break statement.
Instead of forcing termination, it forces the next iteration of the loop to take place, skipping any code in between.
Syntax: continue;
Working of continue statement:
**while loop**
while(expression)
{
statement1;
if(condition)
continue;
statement2;
}
statement3;
**for loop**
for(int;expression;update)
{
statement1;
if(condition)
continue;
statement2;
}
statement3;
**do-while loop**
do
{
statement1;
if(condition)
continue;
statement2;
}while(expression);
statement3;
- For the for loop continue causes the next iteration by updating the variable and then causing the test-expression's evaluation.
- For the while and do-while loops the program control passes to the conditional tests.
Do not confuse the break and continue statements
- A break statement inside a loop will abort the loop and tranfer control to the staement following the loop.
- A continue statement will just abandon the current iteration and let the loop start the next iteration.
Following example establishes the difference between the two:-
#include<iostream.h>
int main()
{
int i;
printf("The loop with break produces output as:\n");
for(i=1;i<=10;++i)
{
if((i%3)==0)
break;
else
printf("%d",i);
}
printf("The loop with continue produces output as:\n")
for(i=1;i<=10;++i)
{
if((i%3)==0)
continue;
else
printf("%d",i);
}
return 0;
}
Output
The loop with break produces output as:
1
2
The loop with continue produces output as:
1
2
4
5
7
8
10
Though, continue is one prominent jump statement, however, experts discourage its use whenever possible.Rather,code can be made more comprehensible by the appropriate use of if/else.