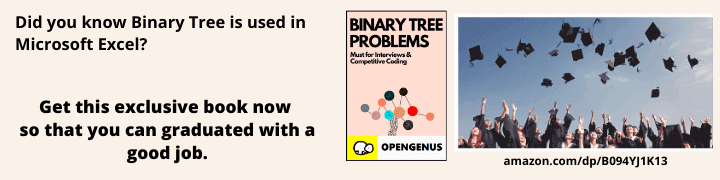
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered how to get the current stock price in Python from Yahoo Finance using two Python libraries, Beautiful Soup, and the Requests library.
Table of contents:
- Basics of Yahoo Finance
- Install the libraries needed
- Import the packages
- Use Requests to send an HTTP request to Yahoo Finance website
- Use Beautiful Soup to parse the content
- Inspect the page to retrieve the stock data
The full code is at the end of the article at OpenGenus.
Yahoo Finance
Yahoo Finance (finance.yahoo.com) is a media property that provides financial news and data about stock quotes, financial reports, financial summaries, etc. A ticker symbol or stock symbol is used to search for the stock information of a particular company. A stock symbol may consist of letters, numbers, or a combination of both. For example, the stock symbol for Apple Inc. is AAPL.
Following are the stock symbol of some of the most popular companies:
- Apple: AAPL
- Amazon: AMZN
- International Business Machines: IBM
- Google: GOOG
- Microsoft: MSFT
- Oracle: ORCL
Note: Amazon and the Amazon logo are trademarks of Amazon.com, Inc. or its affiliates.
Yahoo discontinued its official Finance API in 2017. However, there is an alternative called Yahoo Finance API. The API provides real-time and historical quote data for stocks, cryptocurrencies, ETFs, mutual funds, etc. The API supports different languages such as Python, Java, JavaScript, Ruby, and PHP.
Several Python packages are available to access market data such as yfinance, yahoo-finance, yahoo_fin.
There are alternatives to the Yahoo Finance API such as Alpha Vantage API and Polygon IO.
Install libraries
- To install the requests library, run the following command in your terminal:
python -m pip install requests
- To install Beautiful Soup, run the command below:
pip install beautifulsoup4
Import the packages
The lines of code below import the packages you installed in the previous section.
import requests
from bs4 import BeautifulSoup
Use Requests to send an HTTP request
The Requests library is used to make HTTP requests using Python.
We use the GET method or verb as we need to fetch the stock data from Yahoo Finance.
We call the HTTP GET method on the requests module passing it the URL of the webpage we want to scrape data.
url = f"https://finance.yahoo.com/quote/{symbol}/"
response = requests.get(url)
The symbol variable in the URL represents the stock name or company name whose stock data we are retrieving.
The HTTP request returns a Response object that contains response data such as the encoding, headers, content, status_code, text, etc.
The response. text returns the content of the response in Unicode format.
Parse the content using Beautiful Soup
The Beautiful Soup library is used to scrape websites to retrieve data from HTML and XML files. Beautiful Soup creates a parse tree from the given page that makes it easy to search and extract data.
soup = BeautifulSoup(response.text, "html.parser")
class_ = "My(6px) Pos(r) smartphone_Mt(6px) W(100%)"
We pass two arguments to the Beautiful Soup constructor:
- The content returned from the Response object
- The Html. parser we chose, which is also the default parser. There are parsers available such as lxml, html5lib, etc.
Inspect the page to find the data
To find the stock price we need from the webpage, we need to inspect the webpage.
To inspect the HTML page, we need to open the developer tools. There are several ways of opening the developer tools:
- Use the keyboard shortcut Ctrl + Shift + I
- Right-click on the webpage and select the Inspect option
- In Chrome, open the menu at the top of the page, select the More tools option and select the Developer tools option.
We can hover through the page and select the elements as needed. The corresponding code is on the elements tab.
The stock price is in a span element nested within a div with the class: "My(6px) Pos(r) smartphoneMt(6px) W(100%)".
class_ = "My(6px) Pos(r) smartphone_Mt(6px) W(100%)"
return soup.find("div", class_=class_).find("span").text
The find method takes two arguments, the HTML tag name we are searching for and the attribute. The attribute, in this case, is the class we passed above.
After finding the div with the specified class, we get the first span element and retrieve the text content. The return value of the stock_price function is the current stock price.
Final code
Following is the complete code to get the Get current stock price in Python:
import requests
from bs4 import BeautifulSoup
def stock_price(symbol: str = "AAPL") -> str:
url = f"https://finance.yahoo.com/quote/{symbol}/"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
class_ = "My(6px) Pos(r) smartphone_Mt(6px) W(100%)"
return soup.find("div", class_=class_).find("span").text
if __name__ == "__main__":
for symbol in "AAPL AMZN IBM GOOG MSFT ORCL".split():
print(f"Current {symbol:<4} stock price is {stock_price(symbol):>8}")
Output
Current AAPL stock price is 149.32
Current AMZN stock price is 3,320.37
Current IBM stock price is 127.88
Current GOOG stock price is 2,793.44
Current MSFT stock price is 310.11
Current ORCL stock price is 98.25
With this article at OpenGenus, you must have a strong idea of how to get the current stock price using Beautiful Soup and the Requests library.