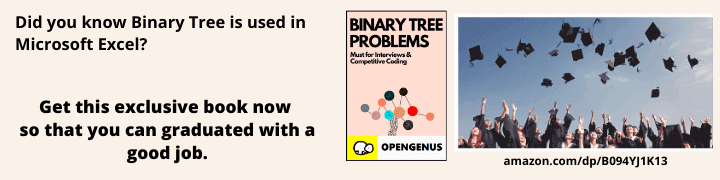
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A dictionary is a collection of key:value pairs which are unordered, changeable and indexed. In Python, dictionaries are written with curly brackets, and they have keys and values in them.
Dictionaries are sometimes called as associative arrays in other languages such as PHP.
The main operation on a dictionary is storing a value with some key and extracting the value through a key. It is also possible to delete a key:value pair using del keyword. If you use the same key for storing a different value, then the new value is overwritten on the old value. If you try to extract a value using a non-existent key then that would give an error.
A dictionary can be created by placing sequence of elements within curly {} braces, separated by comma. A pair of key and value is separated by colon. Dictionary holds a pair of values, one being the Key and the other corresponding pair element being its Key:value. Values in a dictionary can be of any datatype and can be duplicated.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
print(myDict)
Output -
{'site': 'ganofins.com', 'abc': 'qwerty'}
In the above code, we are creating a dictionary named as myDict having site and abc as the key and ganofins.com and qwerty as their values.
How to access an element from a dictionary?
To access a dictionary's element, we can use the key inside the square brackets to obtain its value.
Example 1 -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
print(myDict["site"])
Output 1 -
ganofins.com
Example 2 -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
print(myDict.get("site"))
Output 2 -
ganofins.com
We can also use get() method of a dictionary object to access an element.
How to change value of an item in a dictionary?
We can update a dictionary by adding a new entry or a key-value pair, modifying an existing entry, or deleting an existing entry as shown below -
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
print(myDict["site"])
myDict["site"] = "ganofins.com/blog"
print("After update")
print(myDict["site"])
Output -
>>> print(myDict["site"])
ganofins.com
>>> myDict["site"] = "ganofins.com/blog"
>>> print("After update")
After update
>>> print(myDict["site"])
ganofins.com/blog
In the above code, we updated the value of site key by directly assigning a new value to it.
How to print the whole dictionary, item by item?
Let's see, how we can print a dictionary items one by one. We will be using for loop in the below code.
Example 1 -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
for each_item in myDict:
print(each_item)
Output 1 -
site
abc
In the above code, we used for loop to iterate over each item of a dictionary.
Example 2 -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
for each_item in myDict:
print(myDict[each_item])
Output 2 -
ganofins.com
qwerty
We can also use the dictionary variable myDict to access its item.
Example 3 -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
for each_key, each_value in myDict.items():
print(each_key, each_value)
Output 3 -
site ganofins.com
abc qwerty
In the above code, we used items() method of dictionary object to print the key and value. It returns view object and that view object contains key:value pair.
How to check if a key already exists in the dictionary?
Let's see how we can check if a key already exists within a dictionary.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty"
}
if "site" in myDict:
print("site key already exists")
Output -
site key already exists
Using python's in keyword we can check whether a key already exists in a dictionary or not.
How to calculate the length of dictionary?
Let's see how we can check how many key:value pair a dictionary holds.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"name" : "ganofins"
}
print(len(myDict))
Output -
>>> print(len(myDict))
3
Using python's len() function, we can get the number of key:value pair in a dictionary.
How to add an item to an already created dictionary?
We can add an element to a dictionary in multiple ways. One value at a time can be added to a Dictionary by defining value along with the key e.g. Dict[Key] = ‘Value’. Updating an existing value in a Dictionary can be done by using the built-in update() method. Nested key values can also be added to an existing Dictionary.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"name" : "ganofins"
}
myDict["test"] = 123
print(myDict)
Output -
>>> print(myDict)
{'site': 'ganofins.com', 'abc': 'qwerty', 'name': 'ganofins', 'test': 123}
In the above code, we are creating a new key test and assigning a value to it.
How to remove an item from a dictionary?
We can remove individual dictionary item or key:value using pop() method. Items in a Nested dictionary can also be deleted by using del keyword and providing specific nested key and particular key to be deleted from that nested Dictionary.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"name" : "ganofins"
}
myDict.pop("name")
print(myDict)
Output -
>>> myDict.pop("name")
'ganofins'
>>> print(myDict)
{'site': 'ganofins.com', 'abc': 'qwerty'}
In the above code, we are using pop() method of dictionary object to delete the last key:value pair from a dictionary.
How to completely delete or remove a dictionary?
To explicitly remove an entire dictionary, just use the del keyword.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"name" : "ganofins"
}
del myDict
print(myDict)
Output -
>>> del myDict
>>> print(myDict)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'myDict' is not defined
In the above code, we are using del keyword to delete a key:value pair from a dictionary
How to generate a reference of dictionary to another dictionary?
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"name" : "ganofins"
}
dict = myDict
myDict["test"] = 123
print(dict)
Output -
>>> dict = myDict
>>> myDict["test"] = 123
>>> print(dict)
{'site': 'ganofins.com', 'abc': 'qwerty', 'name': 'ganofins', 'test': 123}
Above, you can see we added test item with value 123 to the myDict dictionary but as we created dict as the reference of myDict. Thus, any changes made in myDict dictionary also reflects in dict dictionary.
More about dictionary
In short a dictionary is a set of key: value pairs, with the requirement that the keys are unique (within one dictionary). A pair of braces creates an empty dictionary: {}. Placing a comma-separated list of key:value pairs within the braces adds initial key:value pairs to the dictionary.
Nested Dictionary
In simple words, a dictionary inside a dictionary is known as nested dictionary. It's a collection of dictionaries into one single dictionary. We can put as many dictionaries as we like in a single dictionary. We're going to create a dictionary within a dictionary.
Example -
myDict = {
"site" : "ganofins.com",
"abc" : "qwerty",
"social" : {
"fb" : "ganofins",
"twitter" : "ganofins"
}
}
print(myDict)
Output -
>>> print(myDict)
{'site': 'ganofins.com', 'abc': 'qwerty', 'social': {'fb': 'ganofins', 'twitter': 'ganofins'}}
In the above code, we created a social dictionary inside myDict dictionary.
The operations above performed on a simple dictionary such addition of an element, popping of an element, updating value of a key, etc can be performed upon the nested dictionary too.
Other than dictionary in a dictionary, we can also put list or tuple inside a dictionary or a dictionary in a list or tuple.
Well I hope you understood all about Dictionary in Python with this article at OpenGenus. If you have any query regarding this, feel free to ask me in the discussion section below.