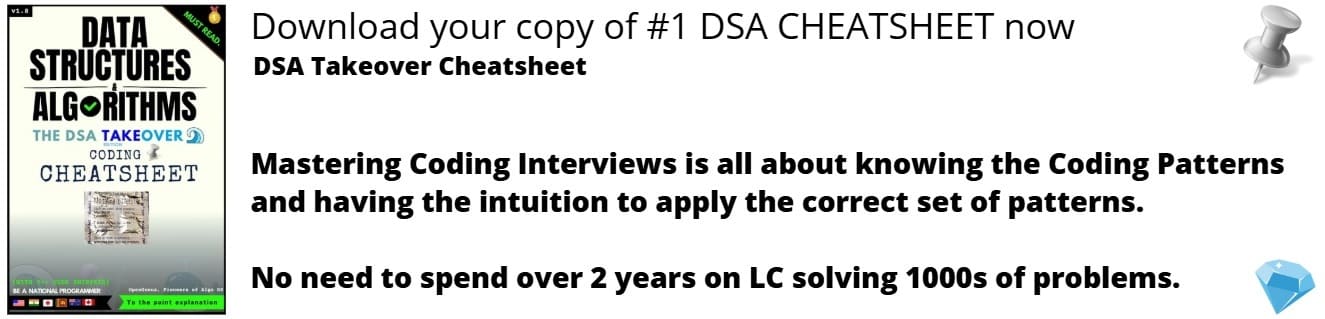
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore the Codeforces API. With codeforces API, one can get access to limited data from in machine-readable JSON format.
What is API?
API stands for Application Programming Interface. An API is a set of routines, protocols, and tools for building software applications. It is a software piece that can be used by another piece of software to allow applications to talk to each other.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition - December 1999.
JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
Getting Started with CodeForces API
To access the data, we need to send an HTTP-request to address
https://codeforces.com/api/{methodName} with method-specific parameters.
Each method call returns a JSON-object with three possible fields.
-
Status
It is either "OK" or "FAILED”. It refers to the status of our request. -
Comment
If the status is "FAILED" then comment contains the reason why our request failed. If the status is "OK", then there is no comment. -
Result
If the status is "OK" then the result contains method-dependent JSON-element, which will be described for each method separately. If the status is "FAILED", then there is no result.
Note:
From the codeforces website, API may be requested at most five times in one second. If more than five requests are sent, we will receive a response with "FAILED" status and "Call limit exceeded" comment.
Methods and Return Objects
The various methods that can be used anonymously to gain publicly available data.
Methods for information relating to a user.
- user.info
Returns the information about one or more than one user, such as name, ranking, title, et cetera.
Required handles: Semicolon-separated list of handles. No more than 10000 handles are accepted.
Example:
https://codeforces.com/api/user.info?handles=DmitriyH;Fefer_Ivan
- user.rating
Returns the list of Rating Change objects for the requested user.
Required handle: Codeforces handle of the user.
Example
https://codeforces.com/api/user.rating?handle=Fefer_Ivan
- user.status
Returns the submissions of a specified user.
Required handle: Codeforces handle of the user.
Parameters from and count can be added to specify the index of the first submission to return and the number of submissions to return, respectively.
Example
https://codeforces.com/api/user.status?handle=Fefer_Ivan&from=1&count=10
- user.blogEntries
Returns a list of all the blog entries by the specified user.
Required handle: Codeforces handle of the user.
Example
https://codeforces.com/api/user.blogEntries?handle=Fefer_Ivan
Methods for Information relating to contests
- contest.list
Returns a list of Contest objects. If this method is called not anonymously, then all available contests for a calling user will be returned, too, including mashups and private gyms.
Parameter gym can be added. If it is true, then gym contests are returned. Otherwise, regular contests are given.
Example
https://codeforces.com/api/contest.list?gym=true
- contest.hacks
Returns list of hacks in the specified contests. Full information about hacks is available only after some time after the contest end. During the contest, the user can see only their hacks.
Required contestId: Id of the contest. It is not the round number. It can be seen in the contest URL.
For example: /contest/566/status
Example
https://codeforces.com/api/contest.hacks?contestId=566
- contest.list
Returns information about all available contests.
Parameter gym can be added. If true, then gym contests are returned. Otherwise, regular contests are returned.
Example
https://codeforces.com/api/contest.list?gym=true
- contest.ratingChanges
Returns rating changes after the contest.
Required contestId: Id of the contest.
Example
https://codeforces.com/api/contest.ratingChanges?contestId=566
- contest.status
Returns submissions for the specified contest. Optionally can return submissions of the specified user.
Required contestId: Id of the contest.
Additionally, parameters handle (Codeforces user handle), from and count can be added.
Example
https://codeforces.com/api/contest.status?contestId=566&from=1&count=10
Methods for Information relating to problem sets
- problemset.problems
Returns all problems from the problem set. Problems can be filtered by tags.
Parameters tags, and problemsetName that are a semicolon-separated list of tags and custom problem set’s short name respectively can be added.
Example
https://codeforces.com/api/problemset.problems?tags=implementation
- problemset.recentStatus
Returns a list of recent submissions, sorted in decreasing order of submission id.
Required count :Number of submissions to return. Can be up to 1000.
The parameter problemsetName can be added.
Example
https://codeforces.com/api/problemset.recentStatus?count=10
Apart from these methods, a few other methods can be seen from Codeforces API Methods.
How to use Codeforces API?
Let us try an example program to get a JSON string about the information of a user.
We will be using json and urlib.request library of python to print the json string.
First, import the required libraries.
import json
import urllib.request
Second, create an empty string to store the JSON object.
user_data = ""
Next, manipulate the URL to get the required information.
user_URL = "https://codeforces.com/api/user.info?handles=" + "smeke"
Finally, load the JSON object.
with urllib.request.urlopen(user_URL) as url:
user_data = json.loads(url.read().decode())
This code returns a JSON string with information about the username provided, in our case user as "user_data".
print(user_data)
It prints the following JSON string:
{
"status":"OK",
"result":[
{
"country":"Japan",
"lastOnlineTimeSeconds":1597605124,
"rating":2624,
"friendOfCount":382,
"titlePhoto":"//userpic.codeforces.com/6559/title/a27a2f085d4cfda2.jpg",
"handle":"smeke",
"avatar":"//userpic.codeforces.com/6559/avatar/f62d05abd1c0eb44.jpg",
"contribution":77,
"organization":"Ildar Gainullin fun-club",
"rank":"international grandmaster",
"maxRating":2624,
"registrationTimeSeconds":1285050079,
"maxRank":"international grandmaster"
}
]
}
To get information about another user, we need to replace the username in the code file, in place of the given “smeke”.
For other kinds of information, we need to choose the appropriate method from the ones given above.
In this way, with this article at OpenGenus, you have learned methods to get information using Codeforces API. We hope this article helped you understand how Codeforces API works.