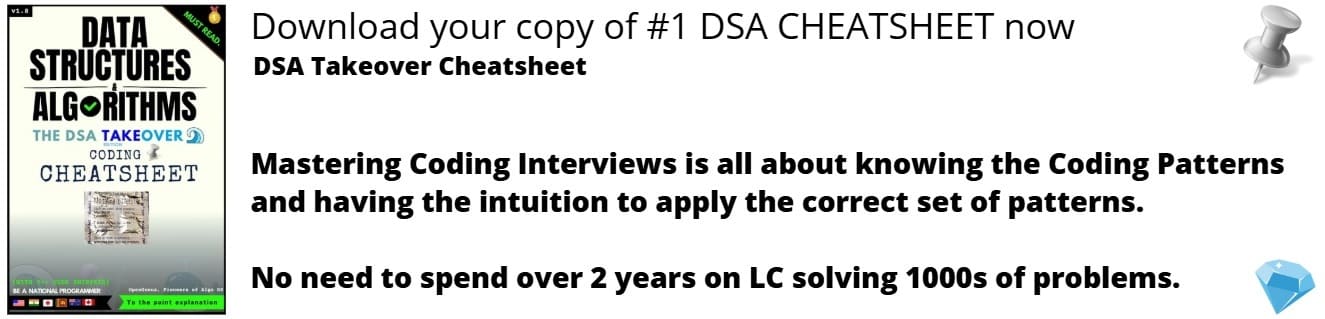
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to find the absolute value of a number and implement the program in C Programming Language.
Table of contents:
- Problem Statement
- Algorithm/ Steps
- Implementation in C
Problem Statement
The problem statement is:
Given a number A, take A as input and print the absolute value of the number A. Write a program in C Programming Language to solve this.
Absolute value of a number A is the number without any negative sign. This means if the number is -90, then the absolute value will be 90. For positive numbers, there will be no change.
Following are two sample input and output to help you understand the problem statement better.
Sample Input and output 1:
Input: 0
|0| = 0
Sample Input and output 2:
Input: -123
|-123| = 123
Algorithm/ Steps
The steps to find the absolute value of a number are as follows:
- Take the number (say A) as input
- If the number A is greater than or equal to 0, A is our answer.
- If the number A is less than 0, then multiply A by -1 and then, the result is our answer.
Pseudocode:
if (A >= 0)
answer = A
else
answer = A*(-1)
Implementation in C
Following is the C program to find the absolute value of a number:
// Part of iq.opengenus.org
#include<stdio.h>
int main()
{
int a,b;
printf("Enter a number=");
scanf("Input: %d",&a);
if(a<0)
{
b=a*(-1);
printf("|%d| = %d",a,b);
}
else
printf("|%d| = %d",a,a);
return 0;
}
Sample Input and output 1:
Input: 10
|10| = 10
Sample Input and output 2:
Input: -9
|-9| = 9
With this article at OpenGenus, you must have the complete idea of how to find the absolute value of a number / integer and implement the program in C.