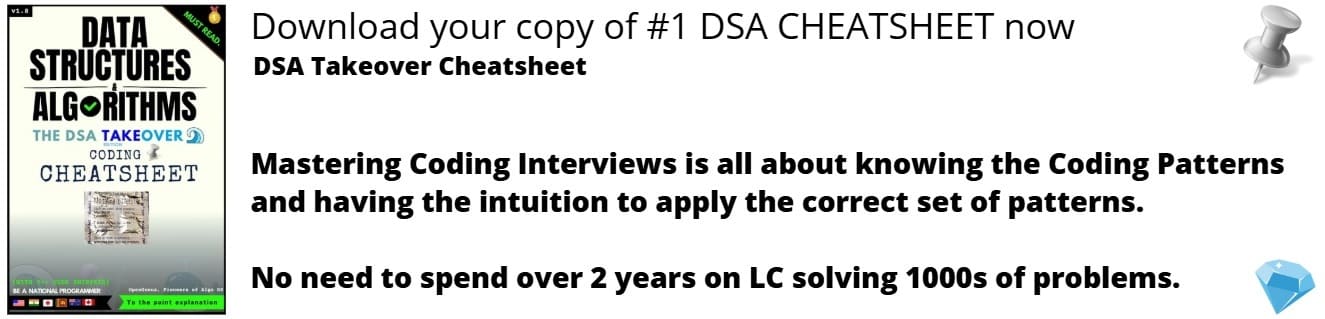
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Java Compiler executes the code in accordance of the order of their appearance , the order followed is top to bottom, hence the code at top is executed prior to the code below it. However , Java provides users with certain statements that facilitate them to control the flow of their program. Such statements are called Control Flow Statements. They are quite crucial for the smooth functioning of any program and are one of the most important features of Java language.
There are prominently three types of Control Flow Statements in Java :
(i) Loop Statements :
* for loop
* while loop
* do while loop
(ii) Decision Making Statements :
* if statements
* switch statement
(iii) Jump Statements :
* break statement
* continue statement
* return statement
Decision Making Statement
These statments basically have two parts- condition and execution statement.
They evaluate boolean expressions and perform control flow based upon the result of the condition. if statements and switch statments are the two types of decision making statements in Java
1. if statement :
(i) if-else statement :
The whole functioning of this statement depends upon the condition, therefore it has two paths.
Syntax :
if(condition) statement1;
else statement2;
Flow Chart
Remember that here each statment can be single or compound enclosed in curly braces or block. The condition basically returns the boolean value. The else part gets executed only when the if part becomes false.
Here is the working:
If the condition is true then statment1 gets executed, otherwise statement2 gets executed.
Code Snippet :
int a = 5 , b = 6;
if(a < b)
System.out.println(a+" is smaller than "+b);
else
System.out.println(a+" is greater than "+b);
Output
5 is smaller than 6
(ii) Simple if statement :
Syntax :
if(condition)
statement;
Code Snippet :
int x = 10 , y = 7;
if(x + y > 15)
System.out.println("Large");
Output
Large
(iii) Nested ifs :
The layman meaning of nested if is "if inside a if statement".
Syntax :
if(condition1){
statement1; // executes when condition1 is true
if(condition2){
statement2; // executes when condition2 is true
}
}
else{
statement3 // executes when condition1 is false
Code Snippet :
int i = 10 , j = 14;
if(i == 10){
if(j < 20){
System.out.println(i + j);
}
}
else{
System.out.println(j - i);
}
Output
24
(iv) The if-else-if ladder :
The if-else-if statement contains the if-statement followed by multiple else-if statements.
Syntax :
if(condition1)
statement1; // executes when condition1 is true
else if(condition2)
statement2; // executes when condition2 is true
else if(condition3)
statement3; // executes when condition3 is true
else
statement4; // executes when condition1 , condition2 and condition3 are false
Code Snippet :
class if_else_if
{
public static void main(String[] abc)
{
int percentage = 50;
if(percentage > 0 && percentage < 40){
System.out.println("Fail);}
else if(percentage >= 40 && percentage < 60){
System.out.println("Pass");}
else if(percentage >= 60 && percentage < 80){
System.out.println("Good");}
else if(percentage >= 80 && percentage < 90){
System.out.println("Very Good");}
else if(percentage >= 90 && percentage <= 100){
System.out.println("Outstanding");}
else{
System.out.println("Invalid Marks");}
}
}
Output
Pass
2. Switch statement :
The switch statement in java is a multidimensional statement. It provides easy and efficient way to execute different part of code based on the value of a expression. It is often considered as a better alternative of if-else-if statements.
Syntax :
switch(expression) {
case value1:
// statement sequence
break;
case value2:
// statement sequence
break;
case value3:
// statement sequence
break;
case value4:
// statement sequence
break;
defualt:
// default statement sequence
}
The switch statement works like this: The value of the expression is compared with each of the values of case statements. If a match if found, the code sequence following that case statement is executed. However, in case no case values match default statement is executed. The choice of default is optional. If no case matches and no default is present, then no further action is taken.
The break statement is used inside the switch to terminate a statement sequence. When a break statement is encountered, execution branches to the first line of code that follows the entire switch statement. This has the ability of jumping out of the switch statement.
Code Snippet :
class switch_demons
{
public static void main(String[] asdf)
{
int day = 2;
switch(day)
{
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
case 4:
System.out.println("Wednesday");
break;
case 5:
System.out.println("Thursday");
break;
case 6:
System.out.println("Friday");
break;
case 7:
System.out.println("Saturday");
break;
default:
System.out.println("Invalid Input");
}
}
}
Output
Tuesday
Points to be noted about switch statement :
- No duplicate cases allowed.
- Case variables can be byte , short , int , char and string as well.
- Default case is optional and gets executed only if no case matches.
- Break statement is optional as well , if not present then control goes to the next case.
Loop Statements
Java statements like while , do while and for are basically iteration statements that are used to achieve what we call loop.
A loop repeatedly executes the same set of instructions until a termination condition is met.
(i) while :
The while loop is one of the most fundamental statements of java. It repeats a statement or block while its controlling expression is true.
Syntax :
while(condition) {
// body of the loop
}
Flow Chart
The condition can be any boolean expression. The body of the loop will be executed till the condition is true. When the condition becomes false, control passes to the next line of the code immediately following the loop. Curly braces are optional in case there is only one statement inside the loop.
Code Snippet :
class while_demons
{
public static void main(String[] args)
{
int val = 5;
while(val > 0)
{
System.out.println("Value : "+val);
val--;
}
}
}
Output
Value : 5
Value : 4
Value : 3
Value : 2
Value : 1
Note : The body of the while loop can be empty because a null statement is syntactically valid.
(ii) do while :
Having seen while loop, it is quite clear that statement contained in the body of the loop will not be executed even once if the condition is false. But sometimes it is desirable to execute the body of the loop even once. In simple words, there are times when one needs to test the termination condition at the end of the loop rather than at the beginning. For this purpost , do while comes to our rescue. The do while loop always executes its body at least once, because its conditional expression is at the bottom of the loop.
Syntax :
do {
// body of the loop
} while(condition);
Code Snippet :
class do_while
{
public static void main(String[] args)
{
int val = 5;
do {
System.out.println("Value : "+val);
val--;
} while(val >= 0);
}
}
Output
Value : 5
Value : 4
Value : 3
Value : 2
Value : 1
Value : 0
(iii) for loop :
for loop is a powerful and versatile construct of Java. They are basically of two form : traditional form and for-each form.
Let's first take a look at traditional form :
Syntax :
for(initialisation; condition; iteration) {
// body
}
The for loop operates as follows : When the loop first starts, the initialisation portion of the loop is executed. Generally, this is an expression that sets the value of the loop control varible, which acts as the counter that controls the loop. Once should note that initialisation part is executed only once. Next condition is evaluated. This basically contains the boolean expression that controls the entire loop. If the condition becomes false then the loop terminates immediately. Next , the iteration portion of the loop is executed. This is usually an expression that increments or decrements the loop control variable. This process repeats until the controlling expression is false.
Code Snippet :
class demons_for
{
public static void main(String[] args)
{
int val = 5;
for(int i = 1 ; i <= val ; i++)
{
System.out.println(i);
}
}
}
Output
1
2
3
4
5
Now let's take a look at the for-each loop :
A for-each loop has been designed to cycle through a collection of objects, such as array, in start to finish fashion. The for-each style of for is also referred to as the enhanced for loop.
Syntax :
for(data_type itr-var : collection/array)
{
// block of statement
}
Here, itr-var specifies the name of the iteration variable that will receive the elements from a collection, one at a time, from beginning to end. The collection being cycled throught is specified by collection. The loop repeats until all elements in the collection have been obtained.
Because the iteration variable receives values from the collection, data_type myst be the same as the elements stored in the collection. Thus, when iterating over arrays, var_type must be compatible with the element type of the array.
Code Snippet :
class demons_for_each_loop
{
public static void main(String[] args)
{
String[] names = {"Suraj","Shivam","Pratik","Sonu","Monu"};
for(String name:names) {
System.out.println(name);
}
}
}
Output
Suraj
Shivam
Pratik
Sonu
Monu
Jump Statements
Java supports three jump statements : break , continue and return. These statements are used to transfer the program control to another part of the program.
(i) break statement :
It terminates statements in switch block, exits a loop and used as a form goto as well. It does not have independent existence in program.
Let's see break statement to exit a loop:
Code Snippet :
class BreakLoop
{
public static void main(String[] asdf)
{
for(int i = 1 ; i < 5 ; i++) {
if(i == 4)
break;
System.out.println("i: "+i);
}
}
}
Output
i: 1
i: 2
i: 3
Now let's see break statement as a form of Goto:
Code Snippet :
class break_demons_goto
{
public static void main(String[] adf)
{
boolean t = true;
a: {
b: {
c: {
System.out.println("Before the break");
if(t)
break b;
System.out.println("Won't execute");
}
System.out.println("Won't execute");
}
System.out.println("After second block");
}
}
}
Output
Before the break
After second block
(ii) continue statement :
Sometimes it becomes necessary to transfer the program control directly to the conditional expression while ignoring the statments. In while and do while loops, a continue statement causes the control to transfer directly to the conditional expression, in case of for loop control goes to the iteration portion and then to the conditional expression. For all the three loops, the code in between gets ignored.
Code Snippet :
class Continue_demons {
public static void main(String[] args){
for(int x = 0 ; x <=2 ; x++) {
for(int y = x ; y <=4 ; y++) {
if(y == 4) {
continue;
}
System.out.println(y);
}
}
}
}
Output
0
1
2
3
1
2
3
2
3
(iii) return statement :
The return statement is used to return a value on completion of program code. It basically returns the value to the part calling the desired method in the program.
Code Snippet :
class demons_return {
public static void main(String[] abc)
{
int val = 10;
if(val % 2 == 0) {
System.out.println("Even number");
return; }
if(val % 10 == 0){
System.out.println("Divisible by 10");
}
}
}
Output
Even number
Here we notice that only "Even number" gets printed because the return statement takes the control out of the main method.
Here are few questions:
1. Which among the follwing is a jump statement in Java?
(A) while
(B) do while
(C) for
(D) break
Answer : (D)
2. for-each loop is also known as-
(A) enhanced for loop
(B) improved for loop
(C) advanced for loop
(D) mature for loop
Answer : (A)
3. What is the difference between while and do while loop?
Answer : while loop does not even execute once if condition is false, whereas do while loop executes atleast once if condition is false.
4. Which among the following is decision making statement in Java?
(A) if statement
(B) nested if statement
(C) do while
(D) continue
Answer : (A) and (B)
5. Which of the following is false about switch statment in Java?
(A) break statement is optional
(B) defualt statement is optional
(C) Any data type can be used as variable
(D) better alternative of if-else-if ladder
Answer : (C)
With this article at OpenGenus, you must have a strong hold on control flow in Java.