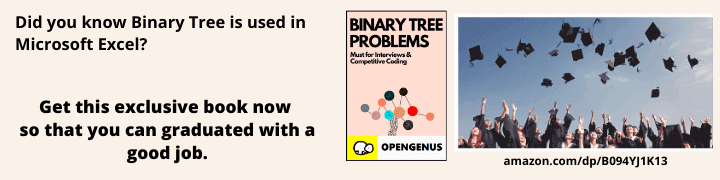
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored Integer (Int Variable) in Python in depth along with complete Python code examples.
Table of Contents:
- Introduction to variables
- What are integer variables?
- How can we manually create int variables?
1. Introduction to Variables
What exactly are variables, and why should we care about int variables? We'll go over such topics in this article and more.
First, variables in programming can be defined as reserved memory locations - or points - that store information in a program. It basically stores data in the program for the computer to utilize whenever it needs to access that data again.
To name variables in Python, there are several rules, including:
- No special characters allowed other than underscore
- Variable name has to start with a letter or underscore
- Can have numbers included in the name but not at the beginning
- Variable names cannot be a Python keyword
So, now that we know the basic idea of variables, why are integer variables different from the others?
2. What are Integer variables?
Integer variables, or "int" variables, are variables that specifically store, as the name suggests, integers as its value. As such, all whole numbers (0, 1, 2, 3, 4, 5, ...) are included in integer variables, including negative numbers (0, -1, -2, -3, -4, -5, ...)
Using the variable naming rules stated before, an example of an integer variable in Python can be:
hello_ = 3
In the example, we can see that the variable "hello_" was assigned 3, and therefore become an integer variable.
If you're curious as to how we can check if the variable is actually an integer variable, you can also use the "type()" function in order to confirm the type of variable you have.
Using our last example of the "hello_" variable, if we type the following line:
type(hello_)
We would get this result:
<class 'int'>
So what would happen if we divide two integer variables? Let's say for instance, 5 and 2.
First, we would assign the two variables, in this case "number_one" and "number_two" can be assigned 5 and 2, respectively, and then we can use Python's built in division operator in order to divide the two numbers.
Like so:
number_one = 5
number_two = 2
result = number_one/number_two
print(result)
And it will give an output of:
2.5
From this, we can see that when you divide two integers and the quotient is not a whole number, Python automatically sets the result variable as a float variable, which can be shown by:
type(result)
Output:
<class 'float'>
If you ever need to double check the type of a variable, utilizing the type() function is very useful.
Furthermore, integer variables are always useful often when dealing with real world objects. For instance, you can't have 4.5 cars, so you would make the variable that is representing cars an integer.
3. How can we manually state int variables?
In order to manually state int variables in python, we can utilize the int() method. This can be useful when you want to convert a float variable into an integer variable.
A float number is used whenever you need to represent real numbers, such as any number that is on the continuous number line (π, 7.5, √2, ...)
Such numbers are useful to represent precise and exact values through decimals, such as 7.99. In order to convert this number into an integer number, you use the float() function in Python.
Be wary though that whenever you convert a float number to an integer variable in Python, your float number will round down to the nearest integer. This is because the int() function trims the numbers after the decimal, returning the integer number.
For an example:
floatnumber = 5.5
newinteger = int(floatnumber)
print(newinteger)
Will output:
5
You could also make an int variable just by utilize the int() variable when you are assigning a variable from the beginning.
For instance, running this code:
integer_variable = int(6)
type(integer_variable)
Will give:
<class 'int'>
In summary: which one of the following is an integer variable?
Question
Which one of the assigned variables is an integer variable?
With this article at OpenGenus, you must have the complete idea of Integer (Int Variable) in Python.