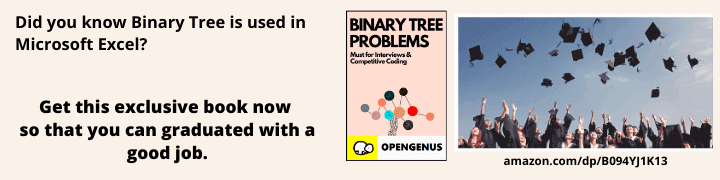
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the 3.4 release of Python, many new features were introduced. One of which is known as the pathlib module. Pathlib has changed the way many programmers perceive file handling by making code more intuitive and in some cases can even make code shorter than its predecessor os.path. Pathlib has made handling files such a breeze that it became a part of the standard library in Python 3.6. But, you may be wondering what exactly this file path business is all about. Let's explore it further.
Why Should You Use Pathlib?
Handling files can involve many different tasks from reading and writing to more complex cases, such as creating a list of every file of the same type within a directory. In the past, completing these tasks using the os.path module has often introduced bugs. The clunky syntax of os.path is less readable and maintainable, which makes it a less desirable solution when compared with pathlib.
Dilemmas with os.path
The os.path module has a few quirks about it that pathlib has improved upon. For one thing, os.path only joins paths with strings, which turns out to be quite an issue in certain cases. Particularly when separators (\
, /
) used in file paths are of opposing directions. This happens to be the case with Windows and Mac. Using os.path.join()
was the only solution to this problem prior to pathlib. However, it's unintuitive syntax has led to more than a few bugs coming up in code later on. Here's a taste of a typical use case of the os.path module.
import os.path
new_folder = os.path.join('my_folder', 'example_files')
file_to_open = os.path.join(new_folder, 'my_file.txt')
open_file = open(file_to_open)
print(open_file.read())
The Benefits of Pathlib
The pathlib module, on the other hand, deals with the file paths for you. So, if you decide that you want to explicitly convert a pure path for a particular operating system (Windows or Unix), you can do so by importing PureWindowsPath
(for Windows) or PurePosixPath
(for Unix), as seen below.
from pathlib import Path, PurePosixPath
file_name = Path('my_folder/example_files/my_file.txt')
converted_posix_path = PurePosixPath(file_name)
print(converted_posix_path)
Otherwise, pathlib can convert a path to be compatible on any operating system without requiring unintuitive syntax. Pathlib has a very natural look and feel. As seen in the very first example, Python pre-3.4 required os.path.join
in order to create a new file in the same directory. Now, it's as simple as using forward slashes.
from pathlib import Path
new_folder = Path('my_folder/example_files/')
file_to_open = new_folder / 'my_file.txt'
open_file = open(file_to_open)
print(open_file.read())
In the above example, pathlib will convert each /
to a \
if needed. Needless to say, pathlib keeps your code clean and concise, and sometimes even safer than os.path.
Important Methods in Pathlib
In addition to a few of the methods that we have explored in earlier examples, below you will find some of the most common pathlib methods that you'll encounter. Many of these are self-explanatory from their names and can be incredibly useful when handling files. This isn't a comprehensive list and it's suggested to check out the official Python documentation to learn more about pathlib methods.
Path.home()
This method will return the user's home directory in an object.
>>> pathlib.Path.home()
PosixPath('/home/opengenus/')
Path.chmod()
chmod()
will allow the user to change the mode and permissions of a file. This includes read and write permissions in octal format. There is Python documentation that covers these in more depth, but below you can get an idea of how the chmod()
function works with the pathlib module.
>>> p = pathlib.Path('opengenus.txt')
>>> p.stat().st_mode
33277
# Make sure that you pass the mode that you would like to change into the method
>>> p.chmod(0o444)
>>> p.stat().st_mode
33060
Path.exists()
The exists()
method is straight-forward; it checks whether or not the path is pointing to an existing file.
>>> pathlib.Path('opengenus.py').exists()
True
Path.cwd()
Easily one of the most commonly used methods, path.cwd() checks the current working directory and returns it as a new path object.
>>> pathlib.Path.cwd()
WindowsPath('C:/Users/username/opengenus/pathlib.txt')
Path.stat()
While the importance of the path.stat() method may not be inherently obvious, it can be helpful when handling files. When invoked, stat()
returns information about the file that is being pointed to.
>>> p = pathlib.Path('pathlib.txt')
>>> p.stat()
os.stat_result(st_mode=33206, st_ino=325103598100813140, st_dev=2319402066, st_nlink=1, st_uid=0, st_gid=0, st_size=11, st_atime=1582292002, st_mtime=1582292002, st_ctime=1582291984)
To prevent the computer from giving back a large amount of unnecessary information, the stat()
method also contains several attributes that allow you to narrow down your results to exactly what you're looking for. In the example below, the st_size
attribute specifies the size of the file.
>>> p = pathlib.Path('pathlib.txt')
>>> p.stat().st_size
2891
Path.read_text()
In the third example, we demonstrated how to open files with the traditional open()
function. However, pathlib also makes it possible to read files without opening or closing them. This returns the everything in the file completely decoded for the user.
>>> p = pathlib.Path('opengenus.txt')
>>> p.read_text()
'Welcome to OpenGenus IQ'
Path.write_text()
Since we're on the subject of interacting with files without calling the open()
or close()
methods, let's take a look at the write_text()
method. It's similar to read_text()
except that it allows you to write data to the targeted file. Use write_text() with caution, though. This method will overwrite anything that is already written to the file.
>>> p = pathlib.Path('opengenus.txt')
>>> p.write_text('Hello, World!')
# Returns the number of characters written in the file
13
# To check that it worked, invoke the read_text() method
>>> p.read_text()
'Hello, World!'
Many of these method examples were formatted for the command line, but can also be used in a text editor when creating a program that handles files. Here is an example of the write_text()
method.
from pathlib import Path
# Absolute path to file
p = Path('my_folder/example_files/')
file_to_open = p / 'open_genus.txt'
print(file_to_open.read_text())
As stated before, there is much more to the pathlib module in Python. The best way to learn more about the pathlib module, its functions, and their attributes is to put them to practice. While it may seem intimidating, pathlib has made handling files in Python easier than before.
Read more:
- OS module (Operating System) in Python by Devansh Thapa
- More Python topics