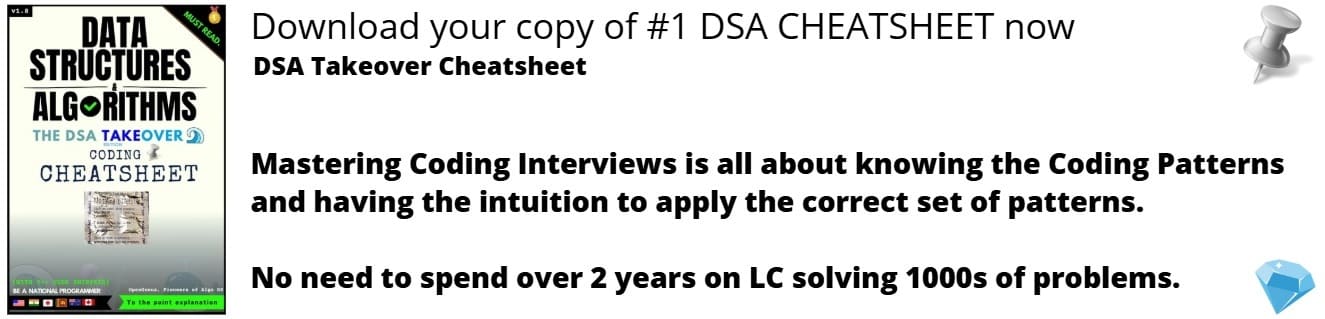
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Many of us are avid users of Twitter. I for one, am on Twitter almost 24/7, whether it is for developer news, networking with coders, or general entertainment!
However, one thing I have always wanted to do is to get a list of tweets with a trending or other particular hashtag (for example, #elonmusk or #python).
In fact, we can actually automate this process with code using the tweepy
library!
In this article, we will learn how to write a Python script to scrape recent tweets wtih a particular hashtag! Without further ado, lets jump in!
Overview
Our Python script should scrape recent tweets with a particular hashtag. Then, it should retweet the most recent tweet. It should continue to do this every minute (as a cron job), retweeting the most recent tweet with a particular hashtag each time.
First of all, we want to scrape data from twitter.com. This means we will need the Twitter API to do so.
Let's think about what we need. We will first require an API key, in order to provide us authentication to use the Twitter API. Next, we will need special elevated access, meaning that we require special permissions to scrape data about hashtags. Currently, we only have essential access. Finally, we will need to set up tweepy
to complete the web scraping.
What Is An API?
An API, or application programming interface, is a way for a program to interact with another through methods, sent using HTTP requests. For the specific case of twitter.com, we can use a built-in library called tweepy
to streamline this process.
However, in order to use the Twitter API and tweepy
, we must be authorized to use the Twitter API.
To set this up, first go to https://developer.twitter.com/en/apply-for-access, and apply for access to be a Twitter Developer! Once you log in and fill out all the information, you get access to your twitter developer portal. Here, you can find your dashboard, but most importantly, the project you created for this Python program. However, notice how you only have essential access.
To sign up for elevated access, fill out this developer form. After this, you wil have full access to all of the elevated features for the Twitter API! Included in this list of elevated features is the ability to find all the tweets from a specific time period with a particular hashtag!
Your Twitter Developer window should end up looking like this:
Finally, set up user authentication settings in your project in order to allow the Twitter API to access your personal Twitter account.
After completing the user authentication settings (setting callback URL to https://localhost/ works), you can now access the tweet searching elevated feature, and have the ability to retweet specific tweets with your Twitter account.
Setup
Next, let's set up the tweepy
library, to be able to use the Twitter API features effectively. Note that for this tutorial, we will be using tweepy version 3.10.0, but the code is not much different for any other versions of tweepy
!
To start off, we will go to our terminal, and in a new virtual environment, install the tweepy library.
pip install tweepy
Next, we will create a new file (not named tweepy.py
). We will first import a few auxilliary libraries required for creating the script.
from datetime import date
from dotenv import load_dotenv
import os
import tweepy
from time import time, sleep
Environment File
In the same folder as your main file, create a .env
file. This will contain any secret important environment files that you don't want others to access, like your API key.
Go to your Twitter Developer Dashboard, and copy your Consumer Keys and Access Keys.
Here is the structure of the .env
file.
export API_KEY={insert api key here}
export API_SECRET={insert api secret key here}
export ACCESS_KEY={insert access key here}
export ACCESS_SECRET={insert access secret key here}
Next, add these lines of code to your main file:
load_dotenv()
consumer_key = os.getenv("API_KEY")
consumer_secret = os.getenv("API_SECRET")
access_token = os.getenv("ACCESS_KEY")
access_token_secret = os.getenv("ACCESS_SECRET")
This will load the exported environment variables into your code, and you can use the os.getenv()
function to get certain environment variables by their names.
Next, we will authorize tweepy to access our Twitter account and the Twitter API.
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)t
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit = True)
We will then define a few constants for future use.
hashtag = "#python"
date_since = date.today()
numtweets = 1
We will be looking for the hashtag of #python
.
Since we are retweeting the most recent tweet, we only need to get that one. However, you can get more tweets if you want to retweet more tweets than the most recent one. .
Event Loop
In our event loop, we will find the most most recent tweets wtih a certain hashtag and retweet the newest one, every minute. Let's look at and break down the code.
while True:
for tweet in api.search(q = hashtag, count = numtweets, lang = "en", until = date_since):
try:
tweet.retweet()
print('Retweeted the tweet')
except tweepy.TweepError as e:
print(e.reason)
break # Only retweet the first one
sleep(60.0 - ((time() - starttime) % 60.0))
In this event loop, we search for tweets with a certain hashtag, and tweet the most recent one. Then, we wait (the rest) of one minute before doing it again.
As an exercise, try to modify the code to retweet the first 2 or 3 tweets.
Conclusion
Here is the full code:
from datetime import date
from dotenv import load_dotenv
import os
import tweepy
from time import time, sleep
load_dotenv()
consumer_key = os.getenv("API_KEY")
consumer_secret = os.getenv("API_SECRET")
access_token = os.getenv("ACCESS_KEY")
access_token_secret = os.getenv("ACCESS_SECRET")
hashtag = "#python"
date_since = date.today()
numtweets = 1
while True:
for tweet in api.search(q = hashtag, count = numtweets, lang = "en", until = date_since):
try:
tweet.retweet()
print('Retweeted the tweet')
except tweepy.TweepError as e:
print(e.reason)
break # Only retweet the first one
sleep(60.0 - ((time() - starttime) % 60.0))
After running the code, you should see that it works by checking your Twitter account, to see that the relevant tweet has been updated.
Now that you know how to write a Python script to retweet recent tweets with a specific hashtag, why not check out other methods of the Twitter API and tweepy
library! If you run into any issues, check out the tweepy
documentation here. As Elon Musk said, "When something is important enough, you do it even if the odds are not in your favor."