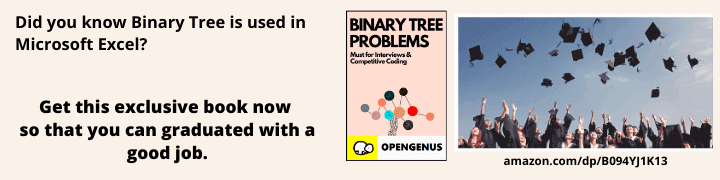
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have developed Python Script to Send WhatsApp Message using two approaches: one Whatsapp library and by using Selenium Web Driver.
Table of contents:
- Approach 1: PyWhatKit
- Approach 2: Selenium
Approach 1: PyWhatKit
A simple way to send a WhatsApp message is to use the PyWhatKit. This is a Python library that can send messages through the WhatsApp web client. The IDE used in this project is Visual Studio Code, but feel free to use any IDE with Python support.
In order to use it, you should have a WhatsApp account linked to your phone number. Then install Flask and PyWhatKit to your python environment.
First we'll make a new project with a python virtual environment:
mkdir whatsappscript
cd whatsappscript
python3 -m venv env
On Mac and Linux, we activate the environment using:
source env/bin/activate
On Windows, we use Powershell to activate the environment:
./env/Scripts/Activate.ps1
The terminal should now have an env tag:
(env) C:\Users\User\whatsappscript
Then we can install packages to the environment using pip
. pip
is installed along with Python and helps manage packages for your environment.
We'll install PyWhatKit and Flask.
pip install Flask
pip install pywhatkit
Then create a file called script.py
. In this script you can add the following code:
import pywhatkit
# Asks a user to type a phonenumber to message
# Note, use international syntax,
# for example a US number would be +17576243392
print("\n")
print("Type a number to message:")
number = input()
# Asks for user to type a message
print("\n")
print("Type a message:")
message = input()
# Send a WhatsApp Message to the contact instantly (gives 10s to load web client before sending)
pywhatkit.sendwhatmsg_instantly(number, message, 10)
The above script will first ask you to input a number and a message then instantly message that given number with a message. For first time use, the script will open up https://web.whatsapp.com/
and the site will require you to connect your phone with the web client by scanning a barcode through the WhatsApp app.
Once connected, the script will then message the given number. It can be run multiple times and different functions can be used depending on what you want the script to do.
The pywhatkit has several methods each with different parameters:
# Same as above but Closes the Tab in 2 Seconds after Sending the Message
pywhatkit.sendwhatmsg("+910123456789", "Hi", 13, 30, 15, True, 2)
# Send an Image to a Group with the Caption as Hello
pywhatkit.sendwhats_image("AB123CDEFGHijklmn", "Images/Hello.png", "Hello")
# Send an Image to a Contact with the no Caption
pywhatkit.sendwhats_image("+910123456789", "Images/Hello.png")
# Send a WhatsApp Message to a Group at 12:00 AM
pywhatkit.sendwhatmsg_to_group("AB123CDEFGHijklmn", "Hey All!", 0, 0)
# Send a WhatsApp Message to a Group instantly
pywhatkit.sendwhatmsg_to_group_instantly("AB123CDEFGHijklmn", "Hey All!")
# Play a Video on YouTube
pywhatkit.playonyt("PyWhatKit")
Approach 2: Selenium
If we wanted to implement this bot without using an external package like PyWhatKit. We can instead directly use the web interface to send WhatsApp messages. In this section, we'll use a tool called Selenium, which can automatically scrape a page for elements and perform actions on those elements.
First open up a Mac/Linux terminal or Windows Powershell.
Let's create a project directory and a new python environment:
mkdir whatsappselenium
cd whatsappselenium
python3 -m venv env
Then we activate our environment either in the Mac/Linux terminal:
source env/bin/activate
Or in Windows Powershell:
./env/Scripts/Activate.ps1
Next we want to install the following libraries, run pip install
in your terminal:
pip install selenium
pip install webdriver-manager
In the same directory we can create a new Python file and open it in any code editor. If you have VS Code install simply run the following to create a new Python file and open it in VS Code.
code script.py
Now add the following to your script. I'll explain it script below:
## IMPORTS ##
from selenium import webdriver
from selenium.webdriver.edge.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from urllib.parse import quote
from re import fullmatch
import time
# Driver Managers
from webdriver_manager.microsoft import EdgeChromiumDriverManager
from webdriver_manager.chrome import ChromeDriverManager
from webdriver_manager.firefox import GeckoDriverManager
from webdriver_manager.opera import OperaDriverManager
from webdriver_manager.utils import ChromeType
## RUNTIME VARIABLES ##
"""
Place the associated driver manager above depending on the browser you want to launch.
Selenium supports the following:
- Chrome
- Edge
- Firefox
- Opera
- Brave
Below the script is configured set to use an Edge browser. Be sure to change it according to the browser you use.
"""
browser = "Edge"
## INPUT ##
"""
Takes input related to sending a text from the recipient phone number and message.
"""
print("\n")
print("Write recipient phone number in format +[Country Code][Area Code][Rest]:")
phone_no = str(input())
print("\nWrite message:")
message = str(input())
## HELPERS ##
"""
Functions that do self explanatory tasks
"""
def modify_number(phone_no):
phone_no = phone_no.replace(" ", "").replace("-", "").replace("(", "").replace(")", "")
return phone_no
def validate_number(phone_no):
def check_number(number):
return "+" in number or "_" in number
if not check_number(number=phone_no):
raise Exception("Country Code Missing in Phone Number!")
if not fullmatch(r"^[\+]?[(]?[0-9]{3}[)]?[-\s\.]?[0-9]{3}[-\s\.]?[0-9]{4,6}$", phone_no):
raise Exception("Invalid Phone Number.")
return True
def set_browser(browser):
install = lambda dm : dm.install()
try:
if (browser == "Edge"):
bm = EdgeChromiumDriverManager()
return webdriver.Edge(service=Service(install(bm)))
elif (browser == "Chrome"):
bm = ChromeDriverManager()
return webdriver.Chrome(service=Service(install(bm)))
elif (browser == "Firefox"):
bm = GeckoDriverManager()
return webdriver.Firefox(service=Service(install(bm)))
elif (browser == "Opera"):
bm = OperaDriverManager()
return webdriver.Opera(service=Service(install(bm)))
elif (browser == "Brave"):
bm = ChromeDriverManager(chrome_type=ChromeType.BRAVE)
return webdriver.Chrome(service=Service(install(bm)))
except:
raise Exception("Browser not found")
## SCRIPT ##
"""
Uses Selenium to send a text
"""
phone_no = modify_number(phone_no)
if (validate_number(phone_no)):
# Loads browser
driver = set_browser(browser)
# Goes to site
site = f"https://web.whatsapp.com/send?phone={phone_no}&text={quote(message)}"
driver.get(site)
# Uses XPATH to find a send button
element = lambda d : d.find_elements(by=By.XPATH, value="//div//button/span[@data-icon='send']")
# Waits until send is found (in case of login)
loaded = WebDriverWait(driver, 1000).until(method=element, message="User never signed in")
# Loads a send button
driver.implicitly_wait(10)
send = element(driver)[0]
# Clicks the send button
send.click()
# Sleeps for 5 secs to allow time for text to send before closing window
time.sleep(5)
# Closes window
driver.close()
The above code is split into 5 sections:
IMPORTS
handles loading relevant libraries (selenium
andwebdriver-manager
)RUNTIME VARIABLES
sets 1 variable related to the browser the script will useINPUT
has code that handles user input from the command line. It collects a recipient phone number and message.HELPERS
functions that do isolated tasksSCRIPT
contains the core functionality of the program.
I'll explain the RUNTIME VARIABLES
, HELPERS
, and SCRIPT
portions in more detail below. INPUT
and IMPORTS
are self explanatory.
For the RUNTIME VARIABLES
section, we define a single variable called browser
. This variable contains a string naming the browser we want our script to use, with the current supported options being Chrome, Edge, Firefox, Opera, and Brave. Be sure to change it in case Edge isn't on your PC.
browser = "Edge"
This variable is passed to a function called set_browser
in the HELPERS
section. The set_browser
method uses a DriverManager() function to identify the location of a given browser
, and then loads its driver and returns it.
HELPERS
also contains two other methods, modify_number()
reformats a phone numbers into a passable form and validate_number()
validates a given phone number.
The SCRIPT
section does all the work.
First it loads a browser to use.
driver = set_browser(browser)
Then send a request to web.whatsapp.com with the given message and recipient:
site = f"https://web.whatsapp.com/send?phone={phone_no}&text={quote(message)}"
driver.get(site)
Then we tell Selenium to wait until a Send button loads in. Here we use an XPATH to find it in our HTML. It searchs for buttons in every div until it finds a button with the tag data-icon="send"
.
d.find_elements(by=By.XPATH, value="//div//button/span[@data-icon='send']")
find_elements()
returns an array of matching elements with the XPATH. In this case it will be an array of size 1.
After waiting we load the send button then click it, using an implicit wait when loading the button.
driver.implicitly_wait(10)
send = condition(driver)[0]
Then we tell Selenium to click the button:
send.click()
After which I tell the browser to stay open for 5 secs before closing the tab.
time.sleep(5)
driver.close()
Feel free to edit the script to add more functionality to the script, like scheduled messages.
With this article at OpenGenus, you must have the complete idea of how to develop a Python Script to Send WhatsApp Message.