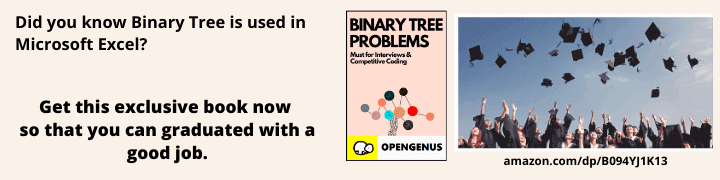
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to learn about the TCHAR, WCHAR, LPSTR, LPWSTR, LPCTSTR in C++ along with code examples.
Frequently, 1 or 2 bytes can be used to represent a character.
The ANSI standard, which is 1 byte in size, is used to represent all English characters.
ALL languages in the world are represented by Unicode, which is a 2 byte insize.
The Visual C++ compiler supports
TCHAR, WCHAR, LPSTR, LPWSTR, LPCTSTR for ANSI and Unicode characters.
TCHAR
TCHAR is used to describe ANSI or Unicode strings. type of char is referred to by the acronym TCHAR. All programming languages support coding in Unicode. TCHAR stands for char in ASCII or multibyte characters, where it allocates 1 byte, While in Unicode it indicates a WCHAR and allocates two bytes.
Syntax
Typedef char TCHAR;
It usually define like this :
#ifndef _UNICODE
typedef char TCHAR;
#else
typedef wchar_t TCHAR;
#endif
WCHAR
Windows data type which contain 16 bit Unicode character . it is referred to by the acronym WCHAR. Also knows as wchar_t type .
Syntax
Typedef char TCHAR;
It usually defined like this :
#if !defined(_NATIVE_WCHAR_T_DEFINED)
typedef unsigned short WCHAR;
#else
typedef wchar_t WCHAR;
#endif
Program Code
//wchar implementation in cpp
#include <iostream>
#include<cwchar>
using namespace std;
//main code
int main()
{
wchar_t string1[] = L"Hello" ;
wchar_t string2[] = L"world" ;
//concat stirng
wcscat(string1, string2);
//display string
wcout << L"STRING = "
<< string1 << endl;
return 0;
}
Output
STRING : Hello world
LPSTR
LPSTR is long pointer string. it is either char * or wchar_t * depend uopn
uncicod is defined or not.
where
LP stand for Long Pointer.
STR stand for string.
LPSTR means constant null-terminated string of CHAR or Long Pointer Constant.
Syntax
typedef const char* LPSTR;
Program code
#include<iostream>
#include<Windows.h>
using namespace std;
main()
{
string str1 = "Welcome";
LPSTR str2 = new TCHAR[str1.size() + 1];
//copy string
strcpy(str2, str1.c_str());
cout << "String 1 : " << str1 <<endl;
cout << "Copy String : " << str2 <<endl;
}
Output
String 1 : Welcome
Copy String : Welcome
LPWSTR
The LPWSTR is a 32-bit pointer to a string of Unicode characters of 16-bit .
It can be null-terminated by null charcter .
It another words it is null-terminated string of WCHAR type or Long Pointer wchar type of string.
Syntax
typedef const char* LPWSTR;
#include <string>
#include <iostream>
#include <Windows.h>
using namespace std;
int main()
{
LPWSTR str1 = L"Hello ";
LPWSTR str2 = L"World";
wstring w1(str1);
wstring w2(str2);
wstring w3 = w1+w2;
wcout << w3 << endl;
}
Output
Hello World
LPCSTR
LPCSTR is a 32-bit pointer to a constant null-terminated string of 8-bit Windows (ANSI) characters. In another word it is simple a string. which is defined by Microsoft . To declare this data type user must include windows.h header file into our progam.
where
LP stand for Long Pointer.
C stand for Constant
STR stand for string
Syntax
typedef const char* LPCSTR;
LPCSTR means constant null-terminated string of CHAR or Long Pointer Constant.
program code
#include<iostream>
#include<Windows.h>
using namespace std;
main()
{
wstring str1 = L"Hello World";
LPCSTR str2 ;
//copy string
str2 = str1.c_str();
wcout << "String 1 : " << str1 <<endl;
wcout << "String 2 : " << str2 <<endl;
}
Output
String 1 : Hello World
String 2 : Hello World