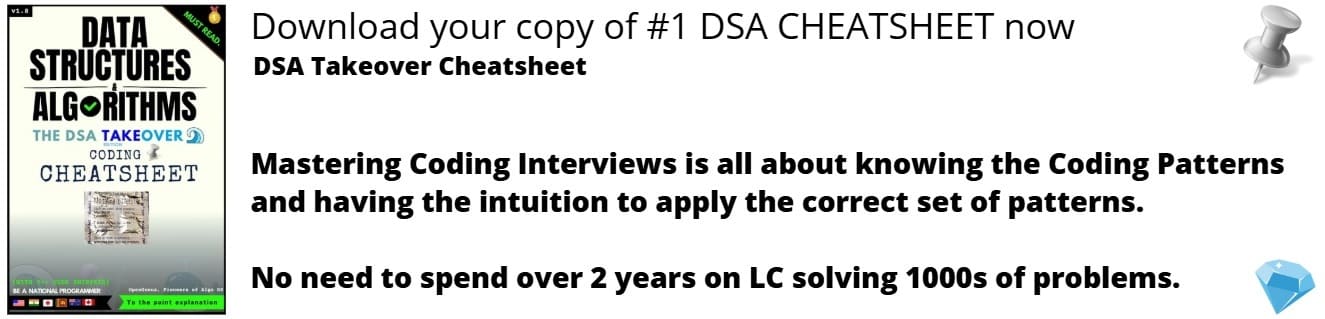
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
PyTorch is one of the most popular Deep Learning (DL) libraries today, thanks to its flexibility and ease of use. However, the extensive range of its features can sometimes lead to confusion, especially when two functions appear to have overlapping purposes. This is the case with torch.Tensor.max and torch.max, two methods that allow users to find maximum values in tensors. So, why does PyTorch provide two different ways for a similar operation? This article aims to clarify their differences, explore their use cases, and help you make an informed choice.
Why Two Functions for Maximum?
PyTorch offers two ways to find maximum values in a tensor because they address different needs:
- torch.Tensor.max is an instance method that applies directly to a torch.Tensor object and is mainly used to find maximum values within a single tensor.
- torch.max is a module-level function used to compare two tensors element-wise.
These two functions, therefore, offer specific possibilities that cater to different scenarios. Letβs examine each in detail.
1. Understanding torch.Tensor.max
Description and Syntax
torch.Tensor.max
is an instance method that applies directly to a torch.Tensor object. Its syntax is as follows:
tensor.max(dim=None, keepdim=False)
dim (int, optional)
: The dimension along which to find the maximum value. If no dimension is specified, the method returns the maximum value across the entire tensor.keepdim (bool, optional)
: If True, retains the original dimension in the output tensor. This is useful for keeping the tensor structure intact.
Use Cases of torch.Tensor.max
This method is ideal when you want to:
- Find the overall maximum value in a tensor.
- Obtain both the maximum value and its index along a specific dimension.
Example
Consider a 2D tensor:
import torch
# Create a 2D tensor
tensor = torch.tensor([[1, 5, 3],
[2, 4, 6]])
# Find the maximum across the entire tensor
max_value = tensor.max()
print(f"Maximum value in the entire tensor: {max_value}")
# Find the maximum value along each row
max_values, indices = tensor.max(dim=1)
print(f"Maximum values by row: {max_values}")
print(f"Indices of maximum values: {indices}")
Output:
Maximum value in the entire tensor: 6
Maximum values by row: tensor([5, 6])
Indices of maximum values: tensor([1, 2])
In this example, we find the global maximum (6) and also the maximum values by row by specifying dim=1.
2. Exploring torch.max
Description and Syntax
torch.max
is a module-level function that compares two tensors element-wise, returning a new tensor containing the maximum values at each position. The syntax is:
torch.max(input, other)
input
: The first tensor.other
: The second tensor (must have the same shape as the first).
Output:
Tensor with maximum values at each position:
tensor([[2, 5, 4],
[2, 5, 6]])
3. Comparing the Two Methods
When to Use torch.Tensor.max
?
If you need to extract the maximum value within a single tensor, whether across the entire tensor or along a specific dimension.
When you need to retrieve the index of the maximum value along a specified dimension.
When to Use torch.max
?
If you want to perform an element-wise comparison between two tensors and retain the maximum value for each position.
Ideal for cases like merging tensors from two sources while keeping the highest values at each position.
Performance and Code Readability
Choosing the appropriate method is important not only for optimization but also for code readability. Using torch.Tensor.max for a two-tensor comparison would require additional steps and make the code more complex.
Conclusion
In summary of this OpenGenus article, PyTorch offers both torch.Tensor.max and torch.max to meet specific needs. torch.Tensor.max is your choice for operations within a single tensor, while torch.max is ideal for element-wise comparisons between two tensors. These distinctions, though subtle, are essential for writing efficient and clear code.