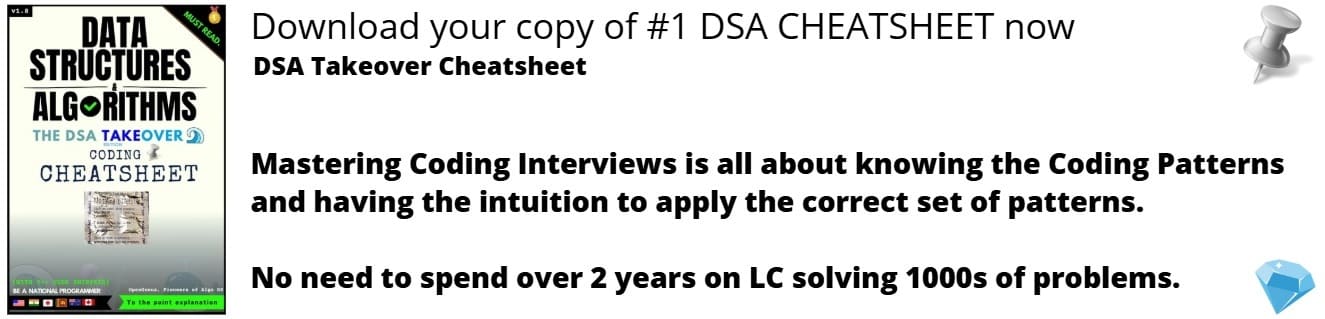
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, I will show 3 different ways to wait for page to load in Python using Selenium.
Table Of Contents:
- Selenium
- Why wait for the page to load
- Quick Selenium setup
- Implements of wait in Selenium
- Implicit wait
- Explicit wait
- Fluent wait
Selenium
Selenium is a free automated testing framework used to validate different web applications from other browsers and platforms. Selenium testing can be done with many different programming languages, such as Java or Python, and many more.
Why wait for the page to load
Many test cases need to be tested and emulated as if a natural person was using them in automated tests. Thus it is not expected in a real-life scenario that the person would be sending requests or using a website until it has fully loaded or at the very least partially loaded.
Quick Selenium setup
First pip install Selenium
pip install Selenium
Next download the corrosponding webdriver for a browser you already have.
Chrome - https://sites.google.com/chromium.org/driver/
Edge - https://developer.microsoft.com/en-us/microsoft-edge/tools/webdriver/
Firefox -https://github.com/mozilla/geckodriver/releases
Safari - https://webkit.org/blog/6900/webdriver-support-in-safari-10/
Next you want to either add the webdriver to your PATH or safe the path to the webdriver and reference it in your code.
Example
With webdriver in path
import selenium
driver = webdriver.Chrome()
driver.get("http://www.python.org")
With webdriver in not in path
import selenium
PATH = "\path to webdriver.exe"
driver = webdriver.Chrome(PATH)
driver.get("http://www.python.org")
Implements of wait in Selenium
Selenium gives the user access to several different options for wait commands. They can instruct a test to wait for a set period or until a condition is met and constantly check if a condition is met.
If using a wait command that waits for a condition that is never met, likely due to an element it was checking for not been locating, it will throw a corresponding exception.
The three main ways to implement a wait
- Implicit wait
- Explicit wait
- Fluent wait
Implicit wait
The implicit wait will wait for an implied or understood though not directly expressed, amount of time. The web driver is given a specified amount of time to wait for in the parameters, and it will wait for a limited amount of time before proceeding to the following instructions. The implicit wait is similar to a sleep function in Python.
The best use case for the implicit wait is if you are trying to create a quick testing prototype. You are using it to test your website, and you know that it takes about 5 seconds to wait for you. Would define the time, giving the website that much time to load.
This is how the implicit wait case could be implemented
from selenium import webdriver
driver = webdriver.Chrome()
driver.implicitly_wait(10) # seconds
driver.get("http://somedomain/url_that_delays_loading") #This is a dummy website URL
myDynamicElement = driver.find_element_by_id("myDynamicElement") #This is a dummy element
Explicit wait
The explicit wait will wait for a fully and clearly expressed amount of time. The web driver is given a specified amount of time to wait for in the parameters, and it will wait for that amount of time, or if a specific condition is met, it will stop waiting that is also in the parameters.
from telnetlib import EC
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.wait import WebDriverWait
driver = webdriver.Chrome()
driver.get("http://somedomain/url_that_delays_loading") #This is a dummy website URL
try:
elem = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.ID, "Element_to_be_found")) #This is a dummy element
)
finally:
driver.quit()
Fluent wait
The fluent wait is a more specified version of the explicit wait. It is more specified in the specific condition for the frequency of the checking if the condition has been met or not.
from telnetlib import EC
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.wait import WebDriverWait
driver = webdriver.Chrome()
driver.get("http://somedomain/url_that_delays_loading") #This is a dummy website URL
POLL_FREQUENCY = 100
try:
elem = WebDriverWait(driver, 10, POLL_FREQUENCY).until(
EC.presence_of_element_located((By.ID, "Element_to_be_found")) #This is a dummy element
)
finally:
driver.quit()
Conditions to wait on with Explict or Fluent wait
The below are a list of the different conditions you can wait on. Below them are the ways in which you can locate elements to then use these conditions with.
Each condition are made to be self explainitory.
- title_is (checks if the title is a certain parameter which you have inputed)
- title_contains (checks if the title contains a certain parameter which you have inputed)
- presence_of_element_located (if a certain element is located)
- visibility_of_element_located (if the visibility of the element is located)
An so on each condition purpose is described by it's name
A simple example using one of them with the line surrounded by comments:
from telnetlib import EC
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.wait import WebDriverWait
driver = webdriver.Chrome()
driver.get("http://somedomain/url_that_delays_loading") #This is a dummy website URL
POLL_FREQUENCY = 100
try:
elem = WebDriverWait(driver, 10, POLL_FREQUENCY).until(
########################################################################
EC.presence_of_element_located((By.ID, "Element_to_be_found"))
# .presence_of_element_located is the condition used in this example
########################################################################
)
finally:
driver.quit()
Rest of the conditions
- visibility_of
- presence_of_all_elements_located
- text_to_be_present_in_element
- text_to_be_present_in_element_value
- frame_to_be_available_and_switch_to_it
- invisibility_of_element_located
- element_to_be_clickable
- staleness_of
- element_to_be_selected
- element_located_to_be_selected
- element_selection_state_to_be
- element_located_selection_state_to_be
- alert_is_present
Locate elements By
Building of of the previous example here is an example using of how you can located the elements by a certain locators
EC.presence_of_element_located((By.ID, "Element_to_be_found"))
# (By.ID, "Element_to_be_found")
# By.ID is the locator being used in this example
# The other options are shown below an example how you would format the other ones
# is the following this would put into the paramaters for the condition
# By.LINK_TEXT, 'link text'
# By.NAME = 'name'
# and so on
- CLASS_NAME = 'class name'¶
- CSS_SELECTOR = 'css selector'
- ID = 'id'
- LINK_TEXT = 'link text'
- NAME = 'name'
- PARTIAL_LINK_TEXT = 'partial link text'
- TAG_NAME = 'tag name'
- XPATH = 'xpath'
With this article at OpenGenus, you must have the complete idea of how to Wait for page load in Selenium in Python.