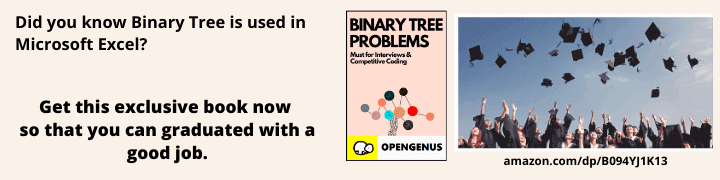
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we are going to learn about auth0, develop a really simple application with it,think about the pros and cons of using auth0 on your applications?
Table of contents:
- What is auth0?
- Why I shouldn’t use it?
- Developing your application
- Installing dependencies
- Components folder
- App.js and Index.js
What is auth0?
Auth0 is a simple way of implementing an authentication and authorizatio service to your application, using it you can manage the security of your application, and it has a simple integration to your code, making your life simple.
Why I shouldn’t use it?
As said before, auth0 is helpfull for when you are trying to develop fast and with security. For a frontend dev using it can add a lot to your projects without compromising the logic of your code, because in this case you shoulnd’t care a lot about this authentification logic, as your are a frontend developer.
But for a backend or fullstack developer you should learn about authentification, tokens, making a clean and good CRUD. So auth0 shoundn’t be use in this case. After making a lot of projects and using your on logic I think it is valid using auth0 for developing faster.
Developing your application
Now that you know the pros and cons of using auth0, let’s develop a simple logic so you can get the gist of it.
Installing dependencies
First you should go to the auth0 website and register, after that go to applications start a new single page application, go to settings and add http://localhost:3000 to allowed callback URLs, allowed logout URLs and to allowed web origins.
After that, go to your vscode and run:
yarn create react-app .
npx create-react-app .
let’s also install the auth0 package to our project
yarn add @auth0/auth0-react
Remove all the useless files in src, the folder should have only app.css, app.js and index.js.
Components folder
After removing all uselles files we are going to create a components' folder inside src, and inside this folder add loginButton.jsx, logoutButton.jsx and userProfile.jsx.
Let’s start developing the loginButton first.
import { useAuth0 } from "@auth0/auth0-react";
function LoginButton() {
const { loginWithRedirect } = useAuth0()
return (
<button onClick={() => loginWithRedirect()}>Log in</button>
)
}
export default LoginButton
On this function we are using the useAuht0, so the user can log inside our single app application with it, just by clicking the button our user is going to be redirected to the auth0 authentication site.
Now let’s develop the logoutButton
import { useAuth0 } from "@auth0/auth0-react";
function LogoutButton() {
const { logout } = useAuth0()
return (
<button onClick={() => logout({ returnTo: window.location.origin})}>Log out</button>
)
}
export default LogoutButton
This is a really simple function that uses auth0 to log out the user off our spa the returning location will be the same, but the user won’t be able to see the information without being logged, making our application logic really simple.
The last component is user Profile, it will be developed like this:
import { useAuth0 } from "@auth0/auth0-react";
function UserProfile() {
const { user, isAuthenticated, isLoading } = useAuth0()
if(isLoading){
return <div>Loading!</div>
}
return (
isAuthenticated && (
<div>
<img src={user.picture} alt="user picture" />
<h2>{user.name}</h2>
<p>{user.email}</p>
</div>
)
)
}
export default UserProfile
In this component we have the user information that we will get based on what email the user will enter our system, then there are 2 other functions, the isLoading function and isAuthenticated function. Isloading will make sure everything was loaded correctly and after that the isAuthenticated will make sure the user is logged and after that we will have a div with the general information of the user.
App.js and Index.js
App.js will have this structure:
import './App.css'
import LoginButton from "./components/loginButton";
import LogoutButton from "./components/logoutButton";
import UserProfile from "./components/userProfile";
function App() {
return (
<div className="App">
<LoginButton/>
<LogoutButton/>
<p>The user information are below!</p>
<UserProfile/>
</div>
);
}
export default App;
It will return all our components with a simple CSS.
This is the CSS code I imported o the app.js:
/* Styles for App component */
.App {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-family: sans-serif;
padding: 2rem;
}
/* Styles for LoginButton component */
.LoginButton {
background-color: #5CAF50;
color: white;
border: none;
padding: 0.5rem 1rem;
cursor: pointer;
margin-right: 1rem;
}
/* Styles for LogoutButton component */
.LogoutButton {
background-color: #f44336;
color: white;
border: none;
padding: 0.5rem 1rem;
cursor: pointer;
}
/* Styles for UserProfile component */
.UserProfile {
margin-top: 2rem;
border: 1px solid #ccc;
padding: 1rem;
max-width: 400px;
}
/* Styles for UserProfile component's header */
.UserProfile h2 {
margin-top: 0;
font-size: 1.5rem;
}
/* Styles for UserProfile component's label */
.UserProfile label {
display: block;
margin-bottom: 0.5rem;
}
/* Styles for UserProfile component's input */
.UserProfile input {
padding: 0.5rem;
border: 1px solid #ccc;
width: 100%;
margin-bottom: 1rem;
}
/* Styles for UserProfile component's button */
.UserProfile button {
background-color: #4CAF50;
color: white;
border: none;
padding: 0.5rem 1rem;
cursor: pointer;
}
The index.js is going to be like this:
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import {Auth0Provider} from '@auth0/auth0-react'
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<Auth0Provider
domain='dev-**************.us.auth0.com'
clientId='*******************************'
redirectUri={window.location.origin}
>
<App />
</Auth0Provider>
);
The only thing I have to point out here is the Auth0Provider that will function based on what information we will give to it, go to setting on your auth0 single page application and copy the domain and the client Id putting this information on the spaces it has to be. After that, your app should run perfectly.