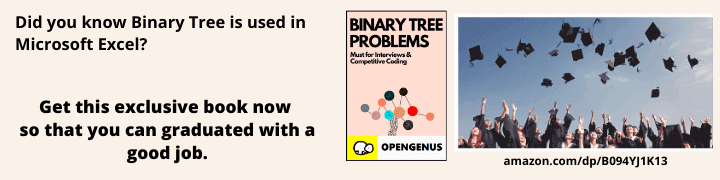
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Auto is a storage class/ keyword in C Programming language which is used to declare a local variable. A local variable is a variable which is accessed only within a function, memory is allocated to the variable automatically on entering the function and is freed on leaving the function.
GNU C has extended auto to allow forward declaration of nested functions as well.
Key points regarding auto keyword:
- auto is used to define local variables (also by default)
- auto is used for forward declaration of nested functions
- auto can result in non-contiguous memory allocation
Syntax:
auto <data_type> <variable_name>;
auto keyword is rarely used as local variables have this behaviour by default.
Example:
#include <stdio.h>
int function()
{
auto int a = 1;
printf("%d", a);
}
int main()
{
function();
return 0;
}
Output:
1
Forward declaration of nested functions
Consider this C code example where the function opengenus_function() is a nested function:
#include <stdio.h>
int function()
{
auto int a = 1;
printf("%d\n", a);
int opengenus_function()
{
int b = 2;
printf("%d", b);
}
opengenus_function();
}
int main()
{
function();
return 0;
}
Output:
1
2
Forward declaration means to add the function definition at the top and define it later. For normal functions, we do so after the define and include statements like:
#include <stdio.h>
int function();
int opengenus_function();
int function()
{
auto int a = 1;
printf("%d\n", a);
int opengenus_function()
{
int b = 2;
printf("%d", b);
}
opengenus_function();
}
int main()
{
function();
return 0;
}
We do this so that the compiler is aware of the function's existence.
If we try to declare the nested function opengenus_function() in the outer function function(), it will give an error. To do this successfully, we need auto. We need to do this as the nested function definition should not be available to code outside the wrapping function.
Consider this C code that declares the nested function wrongly:
#include <stdio.h>
int function();
int function()
{
int opengenus_function(); // compilation error
auto int a = 1;
printf("%d\n", a);
int opengenus_function()
{
int b = 2;
printf("%d", b);
}
opengenus_function();
}
int main()
{
function();
return 0;
}
Compilation error:
opengenus.c: In function 'function':
opengenus.c:11:9: error: static declaration of 'opengenus_function' follows non-static declaration
int opengenus_function()
^
opengenus.c:7:9: note: previous declaration of 'opengenus_function' was here
int opengenus_function(); // compilation error
^
To solve this, we need the auto keyword as:
auto int opengenus_function(); // correct
Complete C code:
#include <stdio.h>
int function();
int function()
{
auto int opengenus_function(); // correct
auto int a = 1;
printf("%d\n", a);
int opengenus_function()
{
int b = 2;
printf("%d", b);
}
opengenus_function();
}
int main()
{
function();
return 0;
}
Output:
1
2
GCC can allocate auto variables non-contiguously
For complex data types, using auto for variable (or by default) may result in non-contiguous memory allocation.
For example, consider this structure for a complex number:
#include <complex.h>
int main()
{
_Complex double x;
return 0;
}
In this example, the variable x is a complex variable (available in C99) with real (__real__
) and imaginary parts (__imag__
). It is possible that the real and imaginary parts are allocated memory far apart and even, real part can be in register while imaginary part can be in main memory.
This happens only in case of data types which involves several components.
With this, you will have a clear idea of using auto keyword in your C code base.