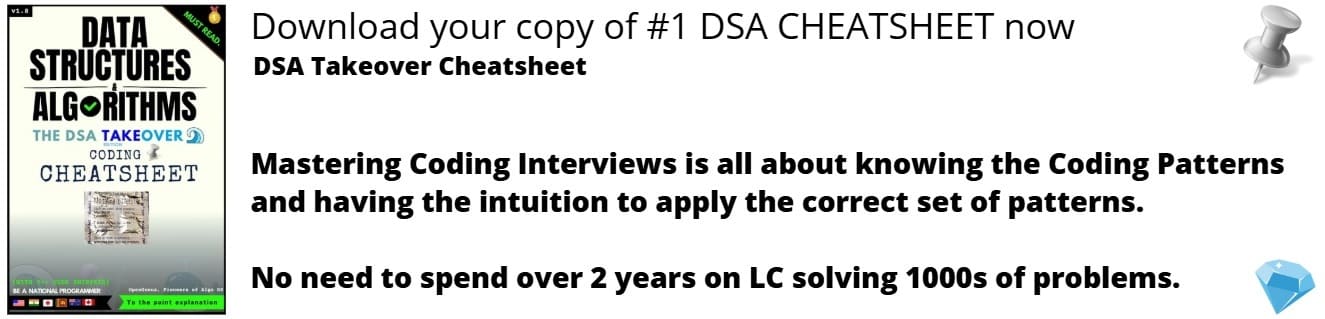
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored different ways to check if a file exists in Python. We have explored 6 different methods to do so in Python:
- try catch block
- isfile()
- isdir()
- exists()
- pathlib
- os.listdir()
Files are used to store information.They have path which determine the location of the file.There are 2 types of paths
- Absolute Path
- Relative Path
Absolute Path:
It is the file path which determines the location of the file starting from the root folder of the file system.
Example:
Lets say there is a file named "file.txt" in a folder named "Files" in D Directory then its absolute path will be "D:\Files\file.txt".
Relative Path:
It is the file path which is relative to the current working directory i.e it determines path from current working directory.There are 2 types of dots one is '.' and other is '..'. Here '.' represents this directory and '..' represents parent folder
Example:
Here the relative path of the same file discussed above is defined as "..\Files\file.txt".
We should use try block for using files because there is a possibility of exception due to different scenarios like
- If the file being searched does not exist
- If the file exists and cannot be accessed
- If the file exists during search and deleted before accessing the contents
The most easier way to check whether a file exists or not is by trying to open it within the try block because there is a possibility of occurence of exception.
It leads to IOError Exception when file does not exist.We would like to use try because it avoids Race Condition.
Race Condition:
If we have multiple processes which can access same file and when one process or thread deletes the file,access operation by other process leads to exception.It leads to sudden stopping of execution of program.
try:
f = open("filename.txt")
print("file opened")
except IOError:
print("File not accessible")
finally:
f.close()
Output:
Here it prints file opened if opened successfully otherwise prints File not accessible.
Other options are
In Python 2 We use different methods in os module to check of the file exists or not.This module allows to access operating system functions.We need to use the path submodule of os module. It consists definitions for different functions like isfile(),isdir(),exists() etc.
From Python 3.4 onwards we use Path object in the pathlib module.
from pathlib import Path
root = Path('/')
root.exists()
root.is_file()
Output:
It prints True if the path contains file or directory for exists() and prints True if the file exists in the given path for is_file.
Before using these methods, the os.path module should be imported.
1. isfile():
It is used to check whether a certain file exists or not.It takes path of the file as parameter and returns True when the file exists and False when the file cannot be found.
import os.path
file_path="/sample/file.txt"
print(os.path.isfile(path))
Output:
It prints True when file exists and False when the file does not exist.
2. isdir():
It is used to check whether a certain directory exists or not.It takes the path of the directory as parameter and returns True when directory is Found and False when directory is not found.
import os.path
dir_path="C/Sample/Games"
if os.path.isdir(dir_path):
print("Directory is found")
else:
print("Directory does not exist")
Output:
It prints Directory is found if directory exists in the given path otherwise prints Directory does not exist.
3. exists():
We can check certain file exists using isfile() and existence of directory using isdir(). But if use to check existence of certain directory using isfile() it returns False and similary if isdir() is used to check existence of file then it returns False.If we want to check the existence of file or directory then exists() is used.
import os.path
print(os.path.exists("this/is/a/directory"))
print(os.path.exists("this/is/a/file.txt"))
print(os.path.exists("not/a/directory/or/file"))
Output:
True
True
False
4. Using pathlib:
From python 3.4 onwards pathlib module is used to check the existence of file or directory.
import pathlib
file = pathlib.Path("sample.txt")
if file.exists ():
print ("File exist")
else:
print ("File not exist")
Output:
File exist
First we have to install pathlib using the following command as
pip install pathlib
The os.path module requires function nesting, but the pathlib modules Path class allows us to chain methods and attributes on Path objects to get an equivalent path representation.
It consists of the same functions of os module
- is_file()- checks whether given Path Object is file or not and returns True if file else False
- is_dir()- checks whether given Path Object is directory or not and returns True if it is a directory else False
- exists() - This method is used to check whether the given Path Object points to a file or directory. If it points to a valid file or directory it returns True otherwise False.
Question
Identify the output of the below code
```
import os.path
dir_path="C/Sample"
print(os.path.is_file(dir_path),end=" ")
print(os.path.is_dir(dir_path),end=" ")
os.rmdir()
print(os.path.exists(dir_path))
```
import os.path
dir_path="C/Sample"
print(os.path.is_file(dir_path),end=" ")
print(os.path.is_dir(dir_path),end=" ")
os.rmdir()
print(os.path.exists(dir_path))
```
5. Using os.listdir():
It takes path of the directory as parameter and returns list of all files and directories in the specified directory. If we don't specify any directory, then list of files and directories in the current working directory will be returned.
import os
dirpath="C:\\Sample\\Textfiles"
if 'file.txt' in os.listdir('dirpath'):
print("File exists in the directory")
else:
print("File does not exist")
Output:
Prints File exists in the directory if it is present in the given directory otherwise prints File does not exist.