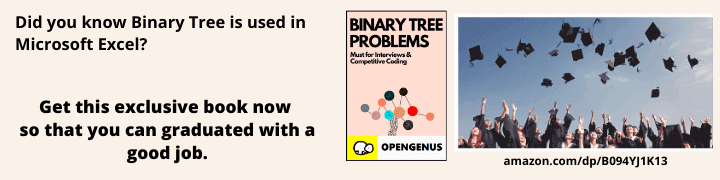
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
To check if an header file has been included or not in a C or C++ code, we need to check if the macro defined in the header file is being defined in the client code. Standard header files like math.h have their own unique macro (like _MATH_H
) which we need to check.
You can use a code as:
#ifndef _MATH_H
#error You need to include math.h
#endif
The macros defined in common header files are as follows:
header file | macro for checking |
---|---|
math.h | _MATH_H |
stdio.h | _STDIO_H |
assert.h | _ASSERT_H |
ctype.h | _CTYPE_H |
signal.h | _SIGNAL_H |
stdarg.h | _STDARG_H |
stdlib.h | _STDLIB_H |
Consider this example of checking if math.h header file has been included or not. If it has been included, the code compilation will proceed as usual or else an error should be raised and the client code will be in the else part of our include condition.
#include <stdio.h>
#ifndef _MATH_H
#error You need to include math.h
#else
int main()
{
// client code
return 0;
}
#endif
It will give a compilation error:
opengenus.c:4:2: error: #error You need to include math.h
#error You need to include math.h
^
If we include math.h, it will not give the error. Check this code:
#include <stdio.h>
#include <math.h>
#ifndef _MATH_H
#error You need to include math.h
#else
int main()
{
// client code
return 0;
}
#endif
It compiles successfully.
Similarly, we can check for two or more include conditions like the following code where we are checking for two header files:
- math.h
- stdio.h
#include <math.h>
#if !defined _MATH_H
#error You need to include math.h
#elif !defined _STDIO_H
#error You need to include stdio.math
#endif
int main()
{
// client code
return 0;
}
It will raise a compilation error:
opengenus.c:6:2: error: #error You need to include stdio.math
#error You need to include stdio.math
^
The macros are defined within the header files and for custom user defined header files, it is strongly advised that you defined custom macro to enable such checking. The first few lines in math.h header file are:
#ifndef _MATH_H
#define _MATH_H 1
#define __GLIBC_INTERNAL_STARTING_HEADER_IMPLEMENTATION
#include <bits/libc-header-start.h>
#if defined log && defined __GNUC__
# warning A macro called log was already defined when <math.h> was included.
# warning This will cause compilation problems.
#endif
__BEGIN_DECLS
/* Get definitions of __intmax_t and __uintmax_t. */
#include <bits/types.h>
/* Get machine-dependent vector math functions declarations. */
#include <bits/math-vector.h>
Now, you have the knowledge required to check where a header file has been included. To do so for custom or other header files, check the contents of the header file to figure out the special macro within it.