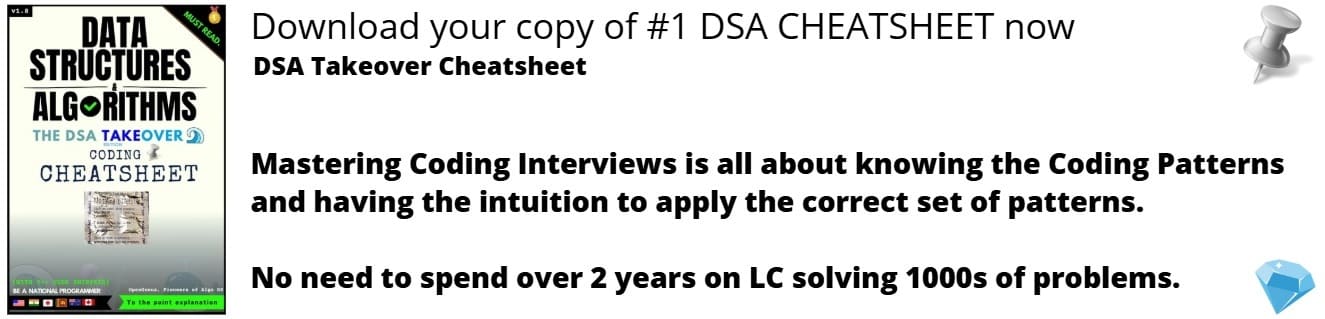
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Introduction to CORS
- Same-Origin Policy
- The Need for Cross-Origin Requests
- CORS Mechanism
- Simple Requests
- Preflight Requests
- Implementing CORS on the Server
- Example: Node.js with Express
- Example: Python with Flask
- Handling CORS on the Client-side
- Fetch API
- XMLHttpRequest
- CORS Libraries
- Common CORS Errors and Troubleshooting
7.1 Error: "Blocked by CORS policy"
7.2 Error: "No 'Access-Control-Allow-Origin' header"
7.3 Error: Preflight request fails
7.4 Error: CORS with Credentials
7.5 Error: CORS in Complex Requests
7.6 Error: Server Doesn't Support CORS
7.7 Error: Mixed Content - Security Considerations
- Conclusion
1. Introduction to CORS
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control how web pages or web applications hosted on one domain can request and interact with resources (such as data, APIs, or assets) hosted on another domain. CORS is a vital component of web security as it prevents unauthorized cross-origin requests that could potentially lead to security vulnerabilities.
2. Same-Origin Policy
The Same-Origin Policy is a fundamental security concept in web browsers that restricts web pages from making requests to a different domain than the one that served the web page. This policy ensures that scripts running in the context of one origin (domain) cannot access or modify the content of another origin.
3. The Need for Cross-Origin Requests
Modern web applications often require resources from multiple domains to function properly. For example, a web page served from https://www.example.com
might need to fetch data from an API hosted at https://api.example.com
. CORS provides a controlled way for servers to specify which origins are allowed to access their resources.
4. CORS Mechanism
CORS operates through two types of requests: simple requests and preflight requests.
Simple Requests
Simple requests are HTTP GET or POST requests with certain restrictions, such as only allowing certain headers. These requests do not trigger a preflight request and are directly processed by the server.
Preflight Requests
Preflight requests are issued by the browser when a request doesn't meet the criteria for a simple request (e.g., using custom headers or specific HTTP methods like PUT or DELETE). The server must respond to the preflight request with appropriate CORS headers indicating whether the actual request is allowed.
5. Implementing CORS on the Server
To enable CORS on a server, it needs to respond with the appropriate CORS headers to inform the browser about which origins are permitted to access its resources. Here are examples of implementing CORS in popular server-side languages:
Example: Node.js with Express
const express = require('express');
const app = express();
app.use((req, res, next) => {
res.header('Access-Control-Allow-Origin', '*');
res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
if (req.method === 'OPTIONS') {
res.sendStatus(200);
} else {
next();
}
});
// ...rest of your code
Example: Python with Flask
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.after_request
def add_cors_headers(response):
response.headers['Access-Control-Allow-Origin'] = '*'
response.headers['Access-Control-Allow-Methods'] = 'GET, POST, PUT, DELETE'
response.headers['Access-Control-Allow-Headers'] = 'Content-Type, Authorization'
return response
# ...rest of your code
6. Handling CORS on the Client-side
Client-side code needs to be aware of CORS when making requests to external domains.
Fetch API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
XMLHttpRequest
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
console.log(data);
}
};
xhr.send();
CORS Libraries
There are several JavaScript libraries that simplify CORS handling, such as Axios and jQuery.
7. Common CORS Errors and Troubleshooting
While Cross-Origin Resource Sharing (CORS) is a powerful tool for enabling cross-origin requests, it can sometimes lead to errors that hinder the proper functioning of your web application. Let's explore some common CORS errors, along with their corresponding code errors and solutions:
7.1 Error: "Blocked by CORS policy"
Code Error:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Solution:
This error occurs when the server does not include the appropriate CORS headers. To fix this, ensure that the server responds with the correct headers. On the server side (e.g., Node.js with Express), add the following headers:
res.header('Access-Control-Allow-Origin', '*');
Replace the *
with the specific origin(s) that you want to allow. It's recommended to be more restrictive in a production environment.
7.2 Error: "No 'Access-Control-Allow-Origin' header"
Code Error:
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data);
}
};
xhr.send();
Solution:
Similar to the previous error, this error indicates that the server is not sending the Access-Control-Allow-Origin
header. Make sure your server includes this header in its responses.
7.3 Error: Preflight request fails
Code Error:
Your client-side code is sending a request that triggers a preflight request (e.g., with custom headers or specific HTTP methods), but the server is not responding correctly to the preflight request.
Solution:
Ensure that your server responds appropriately to preflight requests. Include the necessary headers in the response, such as:
res.header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE');
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
7.4 Error: CORS with Credentials
Code Error:
fetch('https://api.example.com/data', {
credentials: 'include'
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Solution:
When using the credentials: 'include'
option, the server must also include the Access-Control-Allow-Credentials
header in its response. Update your server code to include this header:
res.header('Access-Control-Allow-Credentials', 'true');
7.5 Error: CORS in Complex Requests
Code Error:
Making a request with complex headers or non-standard HTTP methods triggers a preflight request, but the server does not respond to it correctly.
Solution:
Ensure that your server responds properly to preflight requests for complex requests. This includes sending the appropriate headers and handling the preflight request separately if needed.
7.6 Error: Server Doesn't Support CORS
Code Error:
You are trying to make cross-origin requests to a server that does not have CORS enabled.
Solution:
If you do not have control over the server, consider setting up a proxy on your own server that routes the requests to the target server. This way, you can add the necessary CORS headers on your server's responses.
7.7 Error: Mixed Content
Code Error:
Trying to make a secure (HTTPS) request to a non-secure (HTTP) endpoint.
Solution:
Ensure that your application serves content over consistent protocols. If your application is served over HTTPS, make sure the requests are also made over HTTPS.
By understanding these common CORS errors and their solutions, you can effectively troubleshoot and ensure a smooth cross-origin request experience for your web application users.
8. Security Considerations
While CORS enhances web application functionality, it must be configured carefully to prevent potential security vulnerabilities like cross-site request forgery (CSRF) attacks.
9. Conclusion
Cross-Origin Resource Sharing is a fundamental concept for building secure and functional web applications that require interactions with resources hosted on different domains. By understanding CORS mechanisms and implementing them correctly in this OpenGenus article, developers can ensure a seamless and secure user experience.