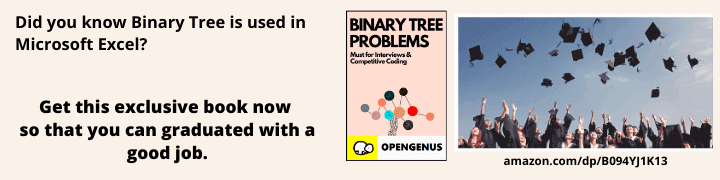
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
To check the operating system of the host in a C or C++ code, we need to check the macros defined by the compiler (GNU GCC or G++). For example, on Windows platform, the compiler has a special macro named _WIN32
defined. So, if the macro _WIN32
is defined, we are on Windows. Similarly, other operating systems have specific macro defined by the compiler.
Example of checking Windows OS:
#ifdef _WIN32
printf("You have Windows Operating System");
#endif
Following is the list of macros defined based on the Operating System:
Operating System | Macro present | Notes |
---|---|---|
Windows 32 bit + 64 bit | _WIN32 |
for all Windows OS |
Windows 64 bit | _WIN64 |
Only for 64 bit Windows |
Apple | __APPLE__ |
for all Apple OS |
Apple | __MACH__ |
alternative to above |
iOS embedded | TARGET_OS_EMBEDDED |
include TargetConditionals.h |
iOS stimulator | TARGET_IPHONE_SIMULATOR |
include TargetConditionals.h |
iPhone | TARGET_OS_IPHONE |
include TargetConditionals.h |
MacOS | TARGET_OS_MAC |
include TargetConditionals.h |
Android | __ANDROID__ |
subset of linux |
Unix based OS | __unix__ |
|
Linux | __linux__ |
subset of unix |
POSIX based | _POSIX_VERSION |
Windows with Cygwin |
Solaris | __sun |
|
HP UX | __hpux |
|
BSD | BSD |
all BSD flavors |
DragonFly BSD | __DragonFly__ |
|
FreeBSD | __FreeBSD__ |
|
NetBSD | __NetBSD__ |
|
OpenBSD | __OpenBSD__ |
Note that the macros are valid for GNU GCC and G++ and may vary for other compilers.
We will go through some basic examples and explore the use of this features in real life as well.
Simple example
In the following example, we are focused on detecting the flavor of Windows we are running which can be a 64 bit or 32 bit. For Windows, our table will be:
Operating System | Macro present |
---|---|
Windows OS 32 bit + 64 bit | _WIN32 |
Windows OS 64 bit | _WIN64 |
As _WIN32
is present in both 32 bit and 64 bit Windows Operating System, we need to first check the presence of _WIN32
to confirm that it is a Windows Operating System and then, check the presence of _WIN64
to confirm whether it is a 64 bit Windows Operating System or a 32 bit Windows Operating System.
Following is the code to check your Windows OS:
#include <stdio.h>
int main()
{
#ifdef _WIN32 // Includes both 32 bit and 64 bit
#ifdef _WIN64
printf("Windows 64 bit\n");
#else
printf("Windows 32 bit\n");
#endif
#else
printf("Not a Windows OS\n");
#endif
return 0;
}
Output:
Windows 32 bit
Example of Apple OS
In this example, we use the macros of Apple OS to detect which Apple OS like MacOS or iPhone is being used.
#include <stdio.h>
int main()
{
#if __APPLE__
#include "TargetConditionals.h"
#if TARGET_OS_IPHONE && TARGET_IPHONE_SIMULATOR
printf("iPhone stimulator\n");
#elif TARGET_OS_IPHONE
printf("iPhone\n");
#elif TARGET_OS_MAC
printf("MacOS\n");
#else
printf("Other Apple OS\n");
#endif
#else
printf("Not an Apple OS\n");
#endif
return 0;
}
Output:
MacOS
General example
In this example, we are detecting if we have a Windows OS or a Linux based OS.
#include <stdio.h>
int main() {
#ifdef _WIN32
printf("Windows\n");
#elif __linux__
printf("Linux\n");
#elif __unix__
printf("Other unix OS\n");
#else
printf("Unidentified OS\n");
#endif
return 0;
}
Usage
With the power to detect the operating system in the language (C and C++ in our case_, we can write a single cross platform code which works on all platforms by separating platform dependant code.
Example:
#include <stdio.h>
int main()
{
#if __APPLE__
// apple specific code
#elif _WIN32
// windows specific code
#elif __LINUX__
// linux specific code
#elif BSD
// BSD specific code
#else
// general code or warning
#endif
// general code
return 0;
}
At the same time, we can write code optimized for specific platforms. For example, a function call may be supported on all platforms but we can optimize it greatly for a specific platform say Linux but this new code will raise errors in other platforms. In this case, we can use the macros to detect if it is Linux and for this case, we can easily use other alternative optimized code.
Example:
#include <stdio.h>
int main()
{
#if __linux__
// linux optimized code (will fail in other platforms)
#else
// general code for all platforms
#endif
// general code
return 0;
}