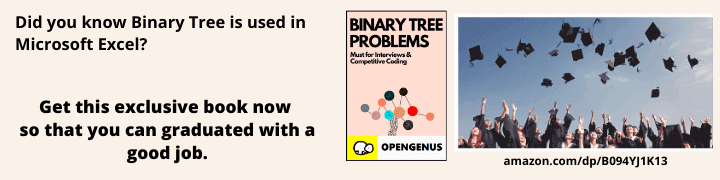
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
Whenever your python program runs into an error or tries to interpret invalid line of code it raises an exception and crashes giving us some information about the error. This should not happen in real world programs. Instead we should handle the exception and prevent the program from crashing.
We will learn about:
- try except block
- the optional else block
- Finally: How to make sure a code section runs irrespective of exceptions?
Try Except Block
Consider we are writing a program to divide two numbers.
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
print("Result: " + str(a/b))
If the user enters first number as 10 and second number as 2 the output will be 5.0. But if the user enters second number as 0 python will raise a ZeroDivisionError
.
Traceback (most recent call last):
File "test.py", line 3, in <module>
ZeroDivisionError: division by zero
That's where try except comes to the rescue. We run the code that can potentially create exceptions in try block and code to handle that exception in except block.
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
try:
print("Result: " + str(a/b))
except ZeroDivisionError:
print("Result: Undefined")
Now whenever user tries to divide by zero the result will be undefined. This is much better than arcane looking error. Note that we are are specifing the exception (ZeroDivisionError) in except block. This is not mandatory. Only using except:
would have worked fine but it is not recommended because it will also supress errors that are unexcpected.
Another scope for try else block is in first two lines of code. Notice that we are converting the input into floats but if the user enters a alphabet then typecasting will fail and python will raise ValueError
. The code below rectifies this problem.
try:
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
except ValueError:
print("Only numerical values required.")
try:
print("Result: " + str(a/b))
except ZeroDivisionError:
print("Result: Undefined")
The optional Else Block
You should have probably noticed by now that the above code is not exactly bug free. Suppose the user inputs a non numeric value for a or b. That will result in printing "Only numerical values required".
But if this happens we want the program to stop executing and not try to print the result. Basically we want to print the result only if the first try block does not raise an exception. This can be achieved by simply indenting the below code in an else block.
try:
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
except ValueError:
print("Only numerical values required.")
else:
try:
print("Result: " + str(a/b))
except ZeroDivisionError:
print("Result: Undefined")
When we use else with try except either the code inside except block or else block will execute.
Finally
: How to make sure a code section runs irrespective of exceptions?
There's also finally keyword which will execute no matter whether try raises an exception or not. Finally is generally used to clean up like closing any opened files before terminating the program.
try:
a = float(input("Enter first number: "))
b = float(input("Enter second number: "))
except ValueError:
print("Only numerical values required.")
else:
try:
print("Result: " + str(a/b))
except ZeroDivisionError:
print("Result: Undefined")
finally:
print("Program terminated.")
Finally our program is bug free and handles all exceptions that can possibly arise. Try it out with different inputs and see if it still spits out any unexpected errors.
We looked at how exception handling allows us to control the flow of execution of our program based on exceptions raised.